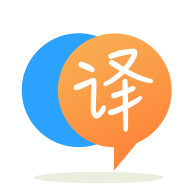
[英](Delphi 10.2) How can I open a URL in Android's web browser from my application?
[英]How can I open a URL in Android's web browser from my application?
如何從內置 web 瀏覽器中的代碼而不是在我的應用程序中打開 URL?
我試過這個:
try {
Intent myIntent = new Intent(Intent.ACTION_VIEW, Uri.parse(download_link));
startActivity(myIntent);
} catch (ActivityNotFoundException e) {
Toast.makeText(this, "No application can handle this request."
+ " Please install a webbrowser", Toast.LENGTH_LONG).show();
e.printStackTrace();
}
但我有一個例外:
No activity found to handle Intent{action=android.intent.action.VIEW data =www.google.com
嘗試這個:
Intent browserIntent = new Intent(Intent.ACTION_VIEW, Uri.parse("http://www.google.com"));
startActivity(browserIntent);
這對我來說很好用。
至於缺少的“http://”,我只會做這樣的事情:
if (!url.startsWith("http://") && !url.startsWith("https://"))
url = "http://" + url;
我也可能會預先填充用戶正在使用“http://”鍵入 URL 的 EditText。
String url = "http://www.stackoverflow.com";
Intent i = new Intent(Intent.ACTION_VIEW);
i.setData(Uri.parse(url));
startActivity(i);
Intent intent = new Intent(Intent.ACTION_VIEW).setData(Uri.parse("http://www.stackoverflow.com"));
startActivity(intent);
Intent intent = new Intent(Intent.ACTION_VIEW, Uri.parse("http://www.stackoverflow.com"));
startActivity(intent);
startActivity(new Intent(Intent.ACTION_VIEW, Uri.parse("http://www.stackoverflow.com")));
快樂編碼!
您可以查看來自 Android Developer 的官方示例。
/**
* Open a web page of a specified URL
*
* @param url URL to open
*/
public void openWebPage(String url) {
Uri webpage = Uri.parse(url);
Intent intent = new Intent(Intent.ACTION_VIEW, webpage);
if (intent.resolveActivity(getPackageManager()) != null) {
startActivity(intent);
}
}
請看一下Intent
的構造函數:
public Intent (String action, Uri uri)
您可以將android.net.Uri
實例傳遞給第二個參數,並根據給定的數據 url 創建一個新的 Intent。
然后,只需調用startActivity(Intent intent)
即可啟動一個新的 Activity,該 Activity 與具有給定 URL 的 Intent 捆綁在一起。
if
check 語句嗎?是的。 文檔說:
如果設備上沒有可以接收隱式意圖的應用程序,您的應用程序將在調用 startActivity() 時崩潰。 要首先驗證應用程序是否存在以接收 Intent,請在您的 Intent 對象上調用 resolveActivity()。 如果結果不為空,則至少有一個應用程序可以處理該意圖,並且調用 startActivity() 是安全的。 如果結果為空,則不應使用該意圖,如果可能,應禁用調用該意圖的功能。
您可以在創建 Intent 實例時寫在一行中,如下所示:
Intent intent = new Intent(Intent.ACTION_VIEW, Uri.parse(url));
在 2.3 中,我有更好的運氣
final Intent intent = new Intent(Intent.ACTION_VIEW).setData(Uri.parse(url));
activity.startActivity(intent);
不同之處在於使用Intent.ACTION_VIEW
而不是字符串"android.intent.action.VIEW"
嘗試這個:
Uri uri = Uri.parse("https://www.google.com");
startActivity(new Intent(Intent.ACTION_VIEW, uri));
或者,如果您想在您的活動中打開網絡瀏覽器,請執行以下操作:
WebView webView = (WebView) findViewById(R.id.webView1);
WebSettings settings = webview.getSettings();
settings.setJavaScriptEnabled(true);
webView.loadUrl(URL);
如果您想在瀏覽器中使用縮放控件,則可以使用:
settings.setSupportZoom(true);
settings.setBuiltInZoomControls(true);
如果您想向用戶顯示所有瀏覽器列表的對話,以便他可以選擇首選,這里是示例代碼:
private static final String HTTPS = "https://";
private static final String HTTP = "http://";
public static void openBrowser(final Context context, String url) {
if (!url.startsWith(HTTP) && !url.startsWith(HTTPS)) {
url = HTTP + url;
}
Intent intent = new Intent(Intent.ACTION_VIEW, Uri.parse(url));
context.startActivity(Intent.createChooser(intent, "Choose browser"));// Choose browser is arbitrary :)
}
就像其他人寫的解決方案(工作正常)一樣,我想回答同樣的問題,但我認為大多數人更願意使用一個技巧。
如果您希望應用程序開始在一個新任務中打開,獨立於您自己的任務,而不是停留在同一個堆棧上,您可以使用以下代碼:
final Intent intent=new Intent(Intent.ACTION_VIEW,Uri.parse(url));
intent.addFlags(Intent.FLAG_ACTIVITY_NO_HISTORY|Intent.FLAG_ACTIVITY_CLEAR_WHEN_TASK_RESET|Intent.FLAG_ACTIVITY_NEW_TASK|Intent.FLAG_ACTIVITY_MULTIPLE_TASK);
startActivity(intent);
還有一種方法可以在Chrome Custom Tabs 中打開 URL。 Kotlin 中的示例:
@JvmStatic
fun openWebsite(activity: Activity, websiteUrl: String, useWebBrowserAppAsFallbackIfPossible: Boolean) {
var websiteUrl = websiteUrl
if (TextUtils.isEmpty(websiteUrl))
return
if (websiteUrl.startsWith("www"))
websiteUrl = "http://$websiteUrl"
else if (!websiteUrl.startsWith("http"))
websiteUrl = "http://www.$websiteUrl"
val finalWebsiteUrl = websiteUrl
//https://github.com/GoogleChrome/custom-tabs-client
val webviewFallback = object : CustomTabActivityHelper.CustomTabFallback {
override fun openUri(activity: Activity, uri: Uri?) {
var intent: Intent
if (useWebBrowserAppAsFallbackIfPossible) {
intent = Intent(Intent.ACTION_VIEW, Uri.parse(finalWebsiteUrl))
intent.addFlags(Intent.FLAG_ACTIVITY_NEW_TASK or Intent.FLAG_ACTIVITY_NO_HISTORY
or Intent.FLAG_ACTIVITY_CLEAR_WHEN_TASK_RESET or Intent.FLAG_ACTIVITY_MULTIPLE_TASK)
if (!CollectionUtil.isEmpty(activity.packageManager.queryIntentActivities(intent, 0))) {
activity.startActivity(intent)
return
}
}
// open our own Activity to show the URL
intent = Intent(activity, WebViewActivity::class.java)
WebViewActivity.prepareIntent(intent, finalWebsiteUrl)
activity.startActivity(intent)
}
}
val uri = Uri.parse(finalWebsiteUrl)
val intentBuilder = CustomTabsIntent.Builder()
val customTabsIntent = intentBuilder.build()
customTabsIntent.intent.addFlags(Intent.FLAG_ACTIVITY_NEW_TASK or Intent.FLAG_ACTIVITY_NO_HISTORY
or Intent.FLAG_ACTIVITY_CLEAR_WHEN_TASK_RESET or Intent.FLAG_ACTIVITY_MULTIPLE_TASK)
CustomTabActivityHelper.openCustomTab(activity, customTabsIntent, uri, webviewFallback)
}
使用 Webview 在同一應用程序中加載 URL 中的其他選項
webView = (WebView) findViewById(R.id.webView1);
webView.getSettings().setJavaScriptEnabled(true);
webView.loadUrl("http://www.google.com");
你也可以這樣走
在 xml 中:
<?xml version="1.0" encoding="utf-8"?>
<WebView
xmlns:android="http://schemas.android.com/apk/res/android"
android:id="@+id/webView1"
android:layout_width="fill_parent"
android:layout_height="fill_parent" />
在java代碼中:
public class WebViewActivity extends Activity {
private WebView webView;
public void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.webview);
webView = (WebView) findViewById(R.id.webView1);
webView.getSettings().setJavaScriptEnabled(true);
webView.loadUrl("http://www.google.com");
}
}
在清單中不要忘記添加互聯網權限...
val browserIntent = Intent(Intent.ACTION_VIEW, uri)
ContextCompat.startActivity(context, browserIntent, null)
我在Uri
上添加了一個擴展,使這更容易
myUri.openInBrowser(context)
fun Uri?.openInBrowser(context: Context) {
this ?: return // Do nothing if uri is null
val browserIntent = Intent(Intent.ACTION_VIEW, this)
ContextCompat.startActivity(context, browserIntent, null)
}
作為獎勵,這里有一個簡單的擴展函數,可以安全地將字符串轉換為 Uri。
"https://stackoverflow.com".asUri()?.openInBrowser(context)
fun String?.asUri(): Uri? {
try {
return Uri.parse(this)
} catch (e: Exception) {}
return null
}
Webview 可用於在您的應用程序中加載 Url。 URL 可以在文本視圖中由用戶提供,也可以硬編碼。
也不要忘記 AndroidManifest 中的互聯網權限。
String url="http://developer.android.com/index.html"
WebView wv=(WebView)findViewById(R.id.webView);
wv.setWebViewClient(new MyBrowser());
wv.getSettings().setLoadsImagesAutomatically(true);
wv.getSettings().setJavaScriptEnabled(true);
wv.setScrollBarStyle(View.SCROLLBARS_INSIDE_OVERLAY);
wv.loadUrl(url);
private class MyBrowser extends WebViewClient {
@Override
public boolean shouldOverrideUrlLoading(WebView view, String url) {
view.loadUrl(url);
return true;
}
}
在您的 try 塊中,粘貼以下代碼,Android Intent 直接使用 URI(統一資源標識符)大括號內的鏈接來標識您的鏈接的位置。
你可以試試這個:
Intent myIntent = new Intent(Intent.ACTION_VIEW, Uri.parse("http://www.google.com"));
startActivity(myIntent);
一個簡短的代碼版本...
if (!strUrl.startsWith("http://") && !strUrl.startsWith("https://")){
strUrl= "http://" + strUrl;
}
startActivity(new Intent(Intent.ACTION_VIEW, Uri.parse(strUrl)));
簡單和最佳實踐
方法一:
String intentUrl="www.google.com";
Intent webIntent = new Intent(Intent.ACTION_VIEW, Uri.parse(intentUrl));
if(webIntent.resolveActivity(getPackageManager())!=null){
startActivity(webIntent);
}else{
/*show Error Toast
or
Open play store to download browser*/
}
方法二:
try{
String intentUrl="www.google.com";
Intent webIntent = new Intent(Intent.ACTION_VIEW, Uri.parse(intentUrl));
startActivity(webIntent);
}catch (ActivityNotFoundException e){
/*show Error Toast
or
Open play store to download browser*/
}
String url = "http://www.example.com";
Intent i = new Intent(Intent.ACTION_VIEW);
i.setData(Uri.parse(url));
startActivity(i);
所以我已經尋找了很長時間,因為所有其他答案都是為該鏈接打開默認應用程序,而不是默認瀏覽器,這就是我想要的。
我終於做到了:
// gathering the default browser
final Intent intent = new Intent(Intent.ACTION_VIEW, Uri.parse("http://"));
final ResolveInfo resolveInfo = context.getPackageManager()
.resolveActivity(intent, PackageManager.MATCH_DEFAULT_ONLY);
String defaultBrowserPackageName = resolveInfo.activityInfo.packageName;
final Intent intent2 = new Intent(Intent.ACTION_VIEW);
intent2.setData(Uri.parse(url));
if (!defaultBrowserPackageName.equals("android") {
// android = no default browser is set
// (android < 6 or fresh browser install or simply no default set)
// if it's the case (not in this block), it will just use normal way.
intent2.setPackage(defaultBrowserPackageName);
}
context.startActivity(intent2);
順便說一句,您可以注意到context
無論如何,因為我已將其用於靜態 util 方法,如果您在活動中執行此操作,則不需要它。
Intent getWebPage = new Intent(Intent.ACTION_VIEW, Uri.parse(MyLink));
startActivity(getWebPage);
簡單,通過意圖查看網站,
Intent viewIntent = new Intent("android.intent.action.VIEW", Uri.parse("http://www.yoursite.in"));
startActivity(viewIntent);
使用這個簡單的代碼在 android 應用程序中查看您的網站。
在清單文件中添加互聯網權限,
<uses-permission android:name="android.permission.INTERNET" />
Chrome 自定義標簽現在可用:
第一步是將自定義選項卡支持庫添加到您的 build.gradle 文件中:
dependencies {
...
compile 'com.android.support:customtabs:24.2.0'
}
然后,打開一個 chrome 自定義選項卡:
String url = "https://www.google.pt/";
CustomTabsIntent.Builder builder = new CustomTabsIntent.Builder();
CustomTabsIntent customTabsIntent = builder.build();
customTabsIntent.launchUrl(this, Uri.parse(url));
更多信息: https : //developer.chrome.com/multidevice/android/customtabs
MarkB 的回答是對的。 就我而言,我使用的是 Xamarin,與 C# 和 Xamarin 一起使用的代碼是:
var uri = Android.Net.Uri.Parse ("http://www.xamarin.com");
var intent = new Intent (Intent.ActionView, uri);
StartActivity (intent);
此信息取自: https : //developer.xamarin.com/recipes/android/fundamentals/intent/open_a_webpage_in_the_browser_application/
根據 Mark B 的回答和以下評論:
protected void launchUrl(String url) {
Uri uri = Uri.parse(url);
if (uri.getScheme() == null || uri.getScheme().isEmpty()) {
uri = Uri.parse("http://" + url);
}
Intent browserIntent = new Intent(Intent.ACTION_VIEW, uri);
if (browserIntent.resolveActivity(getPackageManager()) != null) {
startActivity(browserIntent);
}
}
android.webkit.URLUtil
有方法guessUrl(String)
工作得很好(即使使用file://
或data://
),因為Api level 1
(Android 1.0)。 用於:
String url = URLUtil.guessUrl(link);
// url.com -> http://url.com/ (adds http://)
// http://url -> http://url.com/ (adds .com)
// https://url -> https://url.com/ (adds .com)
// url -> http://www.url.com/ (adds http://www. and .com)
// http://www.url.com -> http://www.url.com/
// https://url.com -> https://url.com/
// file://dir/to/file -> file://dir/to/file
// data://dataline -> data://dataline
// content://test -> content://test
在活動調用中:
Intent intent = new Intent(Intent.ACTION_VIEW, Uri.parse(URLUtil.guessUrl(download_link)));
if (intent.resolveActivity(getPackageManager()) != null)
startActivity(intent);
查看完整的guessUrl
代碼以獲取更多信息。
好的,我檢查了每個答案,但是哪個應用程序具有與用戶想要使用的相同 URL 的深層鏈接?
今天我得到了這個案例,答案是browserIntent.setPackage("browser_package_name");
例如:
Intent browserIntent = new Intent(Intent.ACTION_VIEW, Uri.parse("http://www.google.com"));
browserIntent.setPackage("com.android.chrome"); // Whatever browser you are using
startActivity(browserIntent);
謝謝!
只需使用簡短的方法即可在瀏覽器中打開您的網址:
Intent browserIntent = new Intent(Intent.ACTION_VIEW, Uri.parse("YourUrlHere"));
startActivity(browserIntent);
String url = "https://www.thandroid-mania.com/";
if (url.startsWith("https://") || url.startsWith("http://")) {
Uri uri = Uri.parse(url);
Intent intent = new Intent(Intent.ACTION_VIEW, uri);
startActivity(intent);
}else{
Toast.makeText(mContext, "Invalid Url", Toast.LENGTH_SHORT).show();
}
該錯誤是由於 URL 無效而發生的,Android 操作系統無法為您的數據找到操作視圖。 所以你已經驗證了 URL 是否有效。
簡短而甜蜜的Kotlin輔助函數:
private fun openUrl(link: String) =
startActivity(Intent(Intent.ACTION_VIEW, Uri.parse(link)))
我認為這是最好的
openBrowser(context, "http://www.google.com")
將以下代碼放入全局類
public static void openBrowser(Context context, String url) {
if (!url.startsWith("http://") && !url.startsWith("https://"))
url = "http://" + url;
Intent browserIntent = new Intent(Intent.ACTION_VIEW, Uri.parse(url));
context.startActivity(browserIntent);
}
這種方式使用一種方法,允許您輸入任何字符串而不是固定輸入。 如果重復使用,這確實會節省一些代碼行,因為您只需要三行代碼即可調用該方法。
public Intent getWebIntent(String url) {
//Make sure it is a valid URL before parsing the URL.
if(!url.contains("http://") && !url.contains("https://")){
//If it isn't, just add the HTTP protocol at the start of the URL.
url = "http://" + url;
}
//create the intent
Intent intent = new Intent(Intent.ACTION_VIEW, Uri.parse(url)/*And parse the valid URL. It doesn't need to be changed at this point, it we don't create an instance for it*/);
if (intent.resolveActivity(getPackageManager()) != null) {
//Make sure there is an app to handle this intent
return intent;
}
//If there is no app, return null.
return null;
}
使用這種方法使其普遍可用。 不必將 IT 置於特定活動中,因為您可以像這樣使用它:
Intent i = getWebIntent("google.com");
if(i != null)
startActivity();
或者,如果您想在活動之外啟動它,只需在活動實例上調用 startActivity 即可:
Intent i = getWebIntent("google.com");
if(i != null)
activityInstance.startActivity(i);
正如在這兩個代碼塊中看到的,有一個空檢查。 這是因為如果沒有應用程序來處理意圖,它會返回 null。
如果沒有定義協議,則此方法默認為 HTTP,因為有些網站沒有 SSL 證書(HTTPS 連接所需的證書),如果您嘗試使用 HTTPS 而沒有 SSL 證書,這些網站將停止工作. 任何網站仍然可以強制使用 HTTPS,因此無論哪種方式,這些方面都會讓您使用 HTTPS
由於此方法使用外部資源來顯示頁面,因此您無需聲明 Internet 權限。 顯示網頁的應用程序必須這樣做
//點擊監聽器
@Override
public void onClick(View v) {
String webUrl = news.getNewsURL();
if(webUrl!="")
Utils.intentWebURL(mContext, webUrl);
}
//你的Util方法
public static void intentWebURL(Context context, String url) {
if (!url.startsWith("http://") && !url.startsWith("https://")) {
url = "http://" + url;
}
boolean flag = isURL(url);
if (flag) {
Intent browserIntent = new Intent(Intent.ACTION_VIEW,
Uri.parse(url));
context.startActivity(browserIntent);
}
}
科特林
startActivity(Intent(Intent.ACTION_VIEW).apply {
data = Uri.parse(your_link)
})
從Anko庫方法
fun Context.browse(url: String, newTask: Boolean = false): Boolean {
try {
val intent = Intent(Intent.ACTION_VIEW)
intent.data = Uri.parse(url)
if (newTask) {
intent.addFlags(Intent.FLAG_ACTIVITY_NEW_TASK)
}
startActivity(intent)
return true
} catch (e: ActivityNotFoundException) {
e.printStackTrace()
return false
}
}
在 Android 11 中打開來自 URL 的鏈接的一種新的更好的方法。
try {
val intent = Intent(ACTION_VIEW, Uri.parse(url)).apply {
// The URL should either launch directly in a non-browser app
// (if it’s the default), or in the disambiguation dialog
addCategory(CATEGORY_BROWSABLE)
flags = FLAG_ACTIVITY_NEW_TASK or FLAG_ACTIVITY_REQUIRE_NON_BROWSER or
FLAG_ACTIVITY_REQUIRE_DEFAULT
}
startActivity(intent)
} catch (e: ActivityNotFoundException) {
// Only browser apps are available, or a browser is the default app for this intent
// This code executes in one of the following cases:
// 1. Only browser apps can handle the intent.
// 2. The user has set a browser app as the default app.
// 3. The user hasn't set any app as the default for handling this URL.
openInCustomTabs(url)
}
參考:
https://medium.com/androiddevelopers/package-visibility-in-android-11-cc857f221cd9和https://developer.android.com/training/package-visibility/use-cases#avoid-a-disambiguation-dialog
檢查您的網址是否正確。 對我來說,URL前沒有多余的空格。
基本介紹:
https://在“代碼”中使用該代碼,因此介於兩者之間的任何人都無法讀取它們。 這樣可以使您的信息免受黑客攻擊。
http://只是出於共享目的,因此不安全。
關於您的問題:
XML設計:
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:orientation="vertical"
tools:context="com.example.sridhar.sharedpreferencesstackoverflow.MainActivity">
<LinearLayout
android:orientation="horizontal"
android:background="#228b22"
android:layout_weight="1"
android:layout_width="match_parent"
android:layout_height="0dp">
<Button
android:id="@+id/normal_search"
android:text="secure Search"
android:onClick="secure"
android:layout_weight="1"
android:layout_width="0dp"
android:layout_height="wrap_content" />
<Button
android:id="@+id/secure_search"
android:text="Normal Search"
android:onClick="normal"
android:layout_weight="1"
android:layout_width="0dp"
android:layout_height="wrap_content" />
</LinearLayout>
<LinearLayout
android:layout_weight="9"
android:id="@+id/button_container"
android:layout_width="match_parent"
android:layout_height="0dp"
android:orientation="horizontal">
<WebView
android:id="@+id/webView1"
android:layout_width="match_parent"
android:layout_height="match_parent" />
</LinearLayout>
</LinearLayout>
活動設計:
public class MainActivity extends Activity {
//securely open the browser
public String Url_secure="https://www.stackoverflow.com";
//normal purpouse
public String Url_normal="https://www.stackoverflow.com";
WebView webView;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
webView=(WebView)findViewById(R.id.webView1);
}
public void secure(View view){
webView.setWebViewClient(new SecureSearch());
webView.getSettings().setLoadsImagesAutomatically(true);
webView.getSettings().setJavaScriptEnabled(true);
webView.setScrollBarStyle(View.SCROLLBARS_INSIDE_OVERLAY);
webView.loadUrl(Url_secure);
}
public void normal(View view){
webView.setWebViewClient(new NormalSearch());
webView.getSettings().setLoadsImagesAutomatically(true);
webView.getSettings().setJavaScriptEnabled(true);
webView.setScrollBarStyle(View.SCROLLBARS_INSIDE_OVERLAY);
webView.loadUrl(Url_normal);
}
public class SecureSearch extends WebViewClient{
@Override
public boolean shouldOverrideUrlLoading(WebView view, String Url_secure) {
view.loadUrl(Url_secure);
return true;
}
}
public class NormalSearch extends WebViewClient{
@Override
public boolean shouldOverrideUrlLoading(WebView view, String Url_normal) {
view.loadUrl(Url_normal);
return true;
}
}
}
Android Manifest.Xml權限:
<uses-permission android:name="android.permission.INTERNET"/>
實施此方法時,您會遇到問題:
如果您想不以編程方式使用 XML 執行此操作,您可以在 TextView 上使用:
android:autoLink="web"
android:linksClickable="true"
試試這個。為我工作!
public void webLaunch(View view) {
WebView myWebView = (WebView) findViewById(R.id.webview);
myWebView.setVisibility(View.VISIBLE);
View view1=findViewById(R.id.recharge);
view1.setVisibility(View.GONE);
myWebView.getSettings().setJavaScriptEnabled(true);
myWebView.loadUrl("<your link>");
}
xml代碼:-
<WebView xmlns:android="http://schemas.android.com/apk/res/android"
android:id="@+id/webview"
android:visibility="gone"
android:layout_width="fill_parent"
android:layout_height="fill_parent"
/>
- - - - - 要么 - - - - - - - - -
String url = "";
Intent i = new Intent(Intent.ACTION_VIEW);
i.setData(Uri.parse(url));
startActivity(i);
OmegaIntentBuilder.from(context)
.web("Your url here")
.createIntentHandler()
.failToast("You don't have app for open urls")
.startActivity();
dataWebView.setWebViewClient(new VbLinksWebClient() {
@Override
public void onPageFinished(WebView webView, String url) {
super.onPageFinished(webView, url);
}
});
public class VbLinksWebClient extends WebViewClient
{
@Override
public boolean shouldOverrideUrlLoading(WebView view, String url)
{
view.getContext().startActivity(new Intent(Intent.ACTION_VIEW, Uri.parse(url.trim())));
return true;
}
}
試試這個代碼
AndroidManifest.xml
<manifest xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools"
package="com.example.myapplication5">
<uses-permission android:name="android.permission.INTERNET" />
<application
android:usesCleartextTraffic="true"
android:allowBackup="true"
.....
/>
<activity android:name=".MainActivity"
android:screenOrientation="portrait"
tools:ignore="LockedOrientationActivity">
<intent-filter>
<action android:name="android.intent.action.MAIN" />
<category android:name="android.intent.category.LAUNCHER" />
</intent-filter>
</activity>
</application>
</manifest>
主活動.java
import android.app.Activity;
import android.content.res.Resources;
import android.os.Bundle;
import android.view.View;
import android.view.Window;
import android.webkit.WebSettings;
import android.webkit.WebView;
import android.webkit.WebViewClient;
import android.widget.Toast;
public class MainActivity extends Activity {
private WebView mWebview;
String link = "";// global variable
Resources res;// global variable
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
requestWindowFeature(Window.FEATURE_NO_TITLE);
setContentView(R.layout.home);
loadWebPage();
}
public void loadWebPage()
{
mWebview = (WebView) findViewById(R.id.webView);
WebSettings webSettings = mWebview.getSettings();
webSettings.setJavaScriptEnabled(true);
webSettings.setUseWideViewPort(true);
webSettings.setLoadWithOverviewMode(true);
final Activity activity = this;
mWebview.setWebViewClient(new WebViewClient() {
public void onReceivedError(WebView view, int errorCode, String description, String failingUrl) {
Toast.makeText(activity, description, Toast.LENGTH_SHORT).show();
}
});
mWebview.loadUrl("http://www.google.com");
}
public void reLoad(View v)
{
loadWebPage();
}
}
布局.xml
<?xml version="1.0" encoding="utf-8"?>
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:orientation="vertical">
<TextView
android:id="@+id/textView"
android:layout_width="335dp"
android:layout_height="47dp"
android:layout_alignParentStart="true"
android:layout_alignParentTop="true"
android:layout_marginStart="9dp"
android:layout_marginTop="8dp"
android:paddingLeft="10dp"
android:paddingTop="5dp"
android:text="URL : https://ktmmovie.co/"
android:textSize="18dp"
android:layout_marginLeft="9dp"
android:layout_alignParentLeft="true" />
<com.google.android.material.floatingactionbutton.FloatingActionButton
android:id="@+id/floatingActionButton2"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_alignParentTop="true"
android:layout_alignParentEnd="true"
android:layout_marginStart="7dp"
android:layout_marginLeft="7dp"
android:layout_marginEnd="8dp"
android:layout_toEndOf="@+id/textView"
android:layout_toRightOf="@+id/textView"
android:clickable="true"
android:src="@android:drawable/ic_popup_sync"
android:layout_marginRight="8dp"
android:layout_alignParentRight="true"
android:onClick="reLoad"/>
<WebView
android:id="@+id/webView"
android:layout_width="401dp"
android:layout_height="665dp"
android:layout_below="@+id/textView"
android:layout_alignParentStart="true"
android:layout_alignParentLeft="true"
android:layout_alignParentBottom="true"
android:layout_centerHorizontal="true"
android:layout_marginStart="3dp"
android:layout_marginLeft="3dp"
android:layout_marginTop="3dp"
android:layout_marginBottom="7dp" />
</RelativeLayout>
Kotlin 開發人員可以使用這個
var webpage = Uri.parse(url)
if (!url.startsWith("http://") && !url.startsWith("https://")) {
webpage = Uri.parse("http://$url")
}
val intent = Intent(Intent.ACTION_VIEW, webpage)
if (intent.resolveActivity(packageManager) != null) {
startActivity(intent)
}
Kotlin 解決方案
所有答案都是在url
的默認應用程序中打開 url。 我想總是在瀏覽器中打開任何 url。 我需要 kotlin 中的一些解決方案並實現下面的代碼。
fun getPackageNameForUrl(context: Context, url: String): String? {
val intent = Intent(Intent.ACTION_VIEW, Uri.parse(url))
val resolveInfo = context.packageManager.resolveActivity(intent, PackageManager.MATCH_DEFAULT_ONLY)
return resolveInfo?.activityInfo?.packageName
}
fun openInBrowser(context: Context, url: String) {
val intent = Intent(Intent.ACTION_VIEW, Uri.parse(url))
val packageName = getPackageNameForUrl(context, "http://")
packageName?.takeIf {
it == "android"
}?.let { intent.setPackage(defaultBrowserPackageName); }
startActivity(context, intent, null)
}
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.