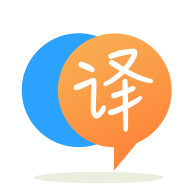
[英]Remote image doesn't get displayed in html page displayed in UIWebView
[英]How to Get the Title of a HTML Page Displayed in UIWebView?
我需要從 UIWebView 中顯示的 HTML 頁面中提取標題標記的內容。 這樣做的最可靠方法是什么?
我知道我可以做到:
- (void)webViewDidFinishLoad:(UIWebView *)webView{
NSString *theTitle=[webView stringByEvaluatingJavaScriptFromString:@"document.title"];
}
但是,這僅在啟用 javascript 時才有效。
或者,我可以只掃描標題的 HTML 代碼文本,但這感覺有點麻煩,而且如果頁面的作者對他們的代碼感到奇怪,可能會證明它很脆弱。 如果涉及到這一點,在 iPhone API 中處理 html 文本的最佳方法是什么?
我覺得我忘記了一些明顯的事情。 還有比這兩種選擇更好的方法嗎?
以下是對這個問題的回答: UIWebView: Can You Disable Javascript? 似乎無法在 UIWebView 中關閉 Javascript。 因此,上面的 Javascript 方法將始終有效。
對於那些只是向下滾動以找到答案的人:
- (void)webViewDidFinishLoad:(UIWebView *)webView{
NSString *theTitle=[webView stringByEvaluatingJavaScriptFromString:@"document.title"];
}
這將始終有效,因為無法在 UIWebView 中關閉 Javascript。
WKWebView
有 'title' 屬性,就這樣做,
func webView(_ wv: WKWebView, didFinish navigation: WKNavigation!) {
title = wv.title
}
我認為UIWebView
現在不合適。
如果啟用了 Javascript 使用這個:-
NSString *theTitle=[webViewstringByEvaluatingJavaScriptFromString:@"document.title"];
如果禁用 Javascript,請使用此:-
NSString * htmlCode = [NSString stringWithContentsOfURL:[NSURL URLWithString:@"http://www.appcoda.com"] encoding:NSASCIIStringEncoding error:nil];
NSString * start = @"<title>";
NSRange range1 = [htmlCode rangeOfString:start];
NSString * end = @"</title>";
NSRange range2 = [htmlCode rangeOfString:end];
NSString * subString = [htmlCode substringWithRange:NSMakeRange(range1.location + 7, range2.location - range1.location - 7)];
NSLog(@"substring is %@",subString);
我在 NSMakeRange 中使用 +7 和 -7 來消除<title>
的長度,即 7
編輯:剛剛看到你找到了答案...... sheeeiiitttt
我真的剛剛學會了這個! 為此,您甚至不需要在 UIWebView 中顯示它。 (但是在使用的過程中,只能獲取當前頁面的URL)
無論如何,這是代碼和一些(無力的)解釋:
//create a URL which for the site you want to get the info from.. just replace google with whatever you want
NSURL *currentURL = [NSURL URLWithString:@"http://www.google.com"];
//for any exceptions/errors
NSError *error;
//converts the url html to a string
NSString *htmlCode = [NSString stringWithContentsOfURL:currentURL encoding:NSASCIIStringEncoding error:&error];
所以我們有了 HTML 代碼,現在我們如何獲得標題? 好吧,在每個基於 html 的文檔中,標題都由 This Is the Title 表示。因此,最簡單的方法可能是在 htmlCode 字符串中搜索 、 、 和子字符串,這樣我們就可以得到介於兩者之間的內容。
//so let's create two strings that are our starting and ending signs
NSString *startPoint = @"<title>";
NSString *endPoint = @"</title>";
//now in substringing in obj-c they're mostly based off of ranges, so we need to make some ranges
NSRange startRange = [htmlCode rangeOfString:startPoint];
NSRange endRange = [htmlCode rangeOfString:endPoint];
//so what this is doing is it is finding the location in the html code and turning it
//into two ints: the location and the length of the string
//once we have this, we can do the substringing!
//so just for easiness, let's make another string to have the title in
NSString *docTitle = [htmlString substringWithRange:NSMakeRange(startRange.location + startRange.length, endRange.location)];
NSLog(@"%@", docTitle);
//just to print it out and see it's right
就是這樣! 所以基本上是為了解釋 docTitle 中發生的所有惡作劇,如果我們僅僅通過說 NSMakeRange(startRange.location, endRange.location) 來創建一個范圍,我們將獲得標題和 startString 的文本(即 ),因為位置是字符串的第一個字符。 所以為了抵消它,我們只是添加了字符串的長度
現在請記住,此代碼未經測試.. 如果有任何問題,則可能是拼寫錯誤,或者我沒有/確實添加了不應該添加的指針。
如果標題有點奇怪並且不完全正確,請嘗試使用 NSMakeRange - 我的意思是添加/減去字符串的不同長度/位置 - 任何看起來合乎邏輯的東西。
如果您有任何疑問或有任何問題,請隨時提問。 這是我在這個網站上的第一個答案,如果它有點混亂,很抱歉
這是 Swift 4 版本,基於這里的答案
func webViewDidFinishLoad(_ webView: UIWebView) {
let theTitle = webView.stringByEvaluatingJavaScript(from: "document.title")
}
到目前為止,我還沒有使用 webviews 的經驗,但是,我相信它將它的標題設置為頁面標題,因此,我建議的一個技巧是在 webview 上使用一個類別並覆蓋 self.title 的設置器,以便您向其中添加一條消息你們中的一個人反對或修改某些屬性以獲得標題。
你能試着告訴我它是否有效嗎?
如果您在代碼中經常需要它,我建議您像這樣在“擴展 UIWebView”中添加一個 func
extension UIWebView {
func title() -> String {
let title: String = self.stringByEvaluatingJavaScript(from: "document.title")!
return title
}
}
或者最好使用 WKWebView。
不幸的是,它在 ARKit 中並沒有得到很好的支持。 我不得不放棄 WKWebView。 我無法將網站加載到 webView 中。 如果有人在這里有解決此問題的方法-> 我有一個類似的問題,這將有很大幫助。
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.