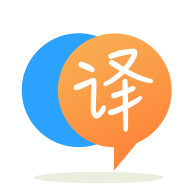
[英]How to open browser window as minimized in selenium python?
[英]Open a program with python minimized or hidden
我想要做的是編寫一個腳本,它只會在進程列表中打開一個應用程序。 這意味着它將被“隱藏”。 我什至不知道它是否可能在python中。
如果它不可能,我什至會滿足於一個允許程序以最小化狀態用python打開的函數,可能是這樣的:
import subprocess
def startProgram():
subprocess.Hide(subprocess.Popen('C:\test.exe')) # I know this is wrong but you get the idea...
startProgram()
有人建議使用 win32com.client 但問題是我要啟動的程序沒有以該名稱注冊的 COM 服務器。
有任何想法嗎?
這很簡單 :)
Python Popen 接受 STARTUPINFO 結構...
關於 STARTUPINFO 結構: https ://msdn.microsoft.com/en-us/library/windows/desktop/ms686331(v=vs.85).aspx
運行隱藏:
import subprocess
def startProgram():
SW_HIDE = 0
info = subprocess.STARTUPINFO()
info.dwFlags = subprocess.STARTF_USESHOWWINDOW
info.wShowWindow = SW_HIDE
subprocess.Popen(r'C:\test.exe', startupinfo=info)
startProgram()
運行最小化:
import subprocess
def startProgram():
SW_MINIMIZE = 6
info = subprocess.STARTUPINFO()
info.dwFlags = subprocess.STARTF_USESHOWWINDOW
info.wShowWindow = SW_MINIMIZE
subprocess.Popen(r'C:\test.exe', startupinfo=info)
startProgram()
您應該使用 win32api 並隱藏您的窗口,例如使用win32gui.EnumWindows您可以枚舉所有頂部窗口並隱藏您的窗口
這是一個小例子,你可以這樣做:
import subprocess
import win32gui
import time
proc = subprocess.Popen(["notepad.exe"])
# lets wait a bit to app to start
time.sleep(3)
def enumWindowFunc(hwnd, windowList):
""" win32gui.EnumWindows() callback """
text = win32gui.GetWindowText(hwnd)
className = win32gui.GetClassName(hwnd)
#print hwnd, text, className
if text.find("Notepad") >= 0:
windowList.append((hwnd, text, className))
myWindows = []
# enumerate thru all top windows and get windows which are ours
win32gui.EnumWindows(enumWindowFunc, myWindows)
# now hide my windows, we can actually check process info from GetWindowThreadProcessId
# http://msdn.microsoft.com/en-us/library/ms633522(VS.85).aspx
for hwnd, text, className in myWindows:
win32gui.ShowWindow(hwnd, False)
# as our notepad is now hidden
# you will have to kill notepad in taskmanager to get past next line
proc.wait()
print "finished."
什么目的?
如果您希望隱藏(無窗口)進程在后台工作,最好的方法是編寫一個 Windows 服務並使用通常的窗口服務機制啟動/停止它。 Windows 服務可以很容易地用 python 編寫,例如這里是我自己的服務的一部分(如果不做一些修改它不會運行)
import os
import time
import traceback
import pythoncom
import win32serviceutil
import win32service
import win32event
import servicemanager
import jagteraho
class JagteRahoService (win32serviceutil.ServiceFramework):
_svc_name_ = "JagteRaho"
_svc_display_name_ = "JagteRaho (KeepAlive) Service"
_svc_description_ = "Used for keeping important services e.g. broadband connection up"
def __init__(self,args):
win32serviceutil.ServiceFramework.__init__(self,args)
self.stop = False
def SvcStop(self):
self.ReportServiceStatus(win32service.SERVICE_STOP_PENDING)
self.log('stopping')
self.stop = True
def log(self, msg):
servicemanager.LogMsg(servicemanager.EVENTLOG_INFORMATION_TYPE,
servicemanager.PYS_SERVICE_STARTED,
(self._svc_name_,msg))
def SvcDoRun(self):
self.log('folder %s'%os.getcwd())
self.ReportServiceStatus(win32service.SERVICE_RUNNING)
self.start()
def shouldStop(self):
return self.stop
def start(self):
try:
configFile = os.path.join(jagteraho.getAppFolder(), "jagteraho.cfg")
jagteraho.start_config(configFile, self.shouldStop)
except Exception,e:
self.log(" stopped due to eror %s [%s]" % (e, traceback.format_exc()))
self.ReportServiceStatus(win32service.SERVICE_STOPPED)
if __name__ == '__main__':
win32serviceutil.HandleCommandLine(AppServerSvc)
你可以安裝它
python svc_jagteraho.py--startup auto install
並運行它
python python svc_jagteraho.py start
我也會出現在服務列表中,例如 services.msc 會顯示它,你可以啟動/停止它,否則你可以使用命令行
sc stop jagteraho
運行隱藏:
from subprocess_maximize import Popen
Popen("notepad.exe",show='hidden', priority=0)
在上面的代碼之前,使用以下命令:
pip install subprocess-maximize
如果出現的是終端,則重定向進程的標准輸出。
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.