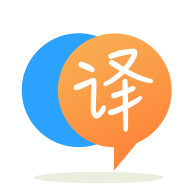
[英]Count number of lines in a string in java - BufferedReader behavior
[英]Count the number of lines in a Java String
需要一些緊湊的代碼來計算 Java 字符串中的行數。 字符串由\\r
或\\n
分隔。 這些換行符的每個實例都將被視為一個單獨的行。 例如 -
"Hello\nWorld\nThis\nIs\t"
應該返回 4. 原型是
private static int countLines(String str) {...}
有人可以提供一組緊湊的陳述嗎? 我在這里有一個解決方案,但我認為它太長了。 謝謝你。
private static int countLines(String str){
String[] lines = str.split("\r\n|\r|\n");
return lines.length;
}
這個怎么樣:
String yourInput = "...";
Matcher m = Pattern.compile("\r\n|\r|\n").matcher(yourInput);
int lines = 1;
while (m.find())
{
lines ++;
}
這樣你就不需要將 String 拆分成很多新的 String 對象,這些對象稍后會被垃圾收集器清理掉。 (當使用String.split(String);
時會發生這種情況)。
一個不創建 String 對象、數組或其他(復雜)對象的非常簡單的解決方案是使用以下內容:
public static int countLines(String str) {
if(str == null || str.isEmpty())
{
return 0;
}
int lines = 1;
int pos = 0;
while ((pos = str.indexOf("\n", pos) + 1) != 0) {
lines++;
}
return lines;
}
請注意,如果您使用其他 EOL 終止符,則需要稍微修改此示例。
對於 Java-11 及更高版本,您可以使用String.lines()
API 執行相同的操作,如下所示:
String sample = "Hello\nWorld\nThis\nIs\t";
System.out.println(sample.lines().count()); // returns 4
API 文檔將以下內容作為描述的一部分:-
Returns: the stream of lines extracted from this string
我在用:
public static int countLines(String input) throws IOException {
LineNumberReader lineNumberReader = new LineNumberReader(new StringReader(input));
lineNumberReader.skip(Long.MAX_VALUE);
return lineNumberReader.getLineNumber();
}
LineNumberReader
在java.io
包中: https : //docs.oracle.com/javase/7/docs/api/java/io/LineNumberReader.html
如果文件中的行已經在字符串中,則可以執行以下操作:
int len = txt.split(System.getProperty("line.separator")).length;
編輯:
以防萬一您需要從文件中讀取內容(我知道您說過沒有,但這是供將來參考),我建議使用Apache Commons將文件內容讀入字符串。 這是一個很棒的庫,還有許多其他有用的方法。 這是一個簡單的例子:
import org.apache.commons.io.FileUtils;
int getNumLinesInFile(File file) {
String content = FileUtils.readFileToString(file);
return content.split(System.getProperty("line.separator")).length;
}
如果您使用 Java 8,則:
long lines = stringWithNewlines.chars().filter(x -> x == '\n').count() + 1;
(如果字符串被修剪,最后+1是計算最后一行)
一條線解決方案
這是一個更快的版本:
public static int countLines(String str)
{
if (str == null || str.length() == 0)
return 0;
int lines = 1;
int len = str.length();
for( int pos = 0; pos < len; pos++) {
char c = str.charAt(pos);
if( c == '\r' ) {
lines++;
if ( pos+1 < len && str.charAt(pos+1) == '\n' )
pos++;
} else if( c == '\n' ) {
lines++;
}
}
return lines;
}
試試這個:
public int countLineEndings(String str){
str = str.replace("\r\n", "\n"); // convert windows line endings to linux format
str = str.replace("\r", "\n"); // convert (remaining) mac line endings to linux format
return str.length() - str.replace("\n", "").length(); // count total line endings
}
行數 = countLineEndings(str) + 1
你好 :)
//import java.util.regex.Matcher;
//import java.util.regex.Pattern;
private static Pattern newlinePattern = Pattern.compile("\r\n|\r|\n");
public static int lineCount(String input) {
Matcher m = newlinePattern.matcher(input);
int count = 0;
int matcherEnd = -1;
while (m.find()) {
matcherEnd = m.end();
count++;
}
if (matcherEnd < input.length()) {
count++;
}
return count;
}
如果它不以 cr/lf cr lf 結尾,這將計算最后一行
"Hello\nWorld\nthis\nIs\t".split("[\n\r]").length
你也可以這樣做
"Hello\nWorld\nthis\nis".split(System.getProperty("line.separator")).length
使用系統默認的行分隔符。
嗯,這是一個不使用“神奇”正則表達式或其他復雜 sdk 功能的解決方案。
顯然,正則表達式匹配器可能更適合在現實生活中使用,因為它編寫起來更快。 (而且它可能也沒有錯誤......)
另一方面,你應該能夠理解這里發生了什么......
如果您想將這種情況 \\r\\n 作為單個換行符(msdos-convention)處理,您必須添加自己的代碼。 提示,您需要另一個變量來跟蹤匹配的前一個字符...
int lines= 1;
for( int pos = 0; pos < yourInput.length(); pos++){
char c = yourInput.charAt(pos);
if( c == "\r" || c== "\n" ) {
lines++;
}
}
new StringTokenizer(str, "\r\n").countTokens();
請注意,這不會計算空行 (\\n\\n)。
CRLF (\\r\\n) 算作單換行符。
此方法將分配一個字符數組,應使用它而不是迭代 string.length(),因為 length() 使用 Unicode 字符數而不是字符數。
int countChars(String str, char chr) {
char[] charArray = str.toCharArray();
int count = 0;
for(char cur : charArray)
if(cur==chr) count++;
return count;
}
StringUtils.countMatches()
?
在你的情況下
StringUtils.countMatches("Hello\nWorld\nThis\nIs\t", "\n") + StringUtils.countMatches("Hello\nWorld\nThis\nIs\t", "\r") + 1
應該工作正常。
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.