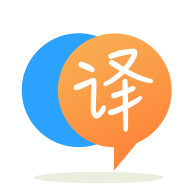
[英]How does a C++ std::container (vector) store its internals (element address, access by index)?
[英]C++ STL: How to iterate vector while requiring access to element and its index?
我經常發現自己需要迭代STL向量 。 當我這樣做時,我需要訪問vector 元素及其索引 。
我曾經這樣做:
typedef std::vector<Foo> FooVec;
typedef FooVec::iterator FooVecIter;
FooVec fooVec;
int index = 0;
for (FooVecIter i = fooVec.begin(); i != fooVec.end(); ++i, ++index)
{
Foo& foo = *i;
if (foo.somethingIsTrue()) // True for most elements
std::cout << index << ": " << foo << std::endl;
}
在發現BOOST_FOREACH之后 ,我將其縮短為:
typedef std::vector<Foo> FooVec;
FooVec fooVec;
int index = -1;
BOOST_FOREACH( Foo& foo, fooVec )
{
++index;
if (foo.somethingIsTrue()) // True for most elements
std::cout << index << ": " << foo << std::endl;
}
當需要引用向量元素及其索引時,是否有更好或更優雅的方法來迭代STL向量?
我知道替代方案: for (int i = 0; i < fooVec.size(); ++i)
但我一直在閱讀如何迭代這樣的STL容器不是一個好習慣。
for (size_t i = 0; i < vec.size(); i++)
elem = vec[i];
向量是C數組上的薄包裝器; 無論你使用迭代器還是索引,它都同樣快。 其他數據結構雖然不那么寬容,例如std :: list。
您始終可以在循環中計算索引:
std::size_t index = std::distance(fooVec.begin(), i);
對於向量,這很可能被實現為單指針減法操作,因此它不是特別昂貴。
優雅是旁觀者的眼睛,但請記住指針/迭代器算術:)
for (FooVecIter i = fooVec.begin(); i != fooVec.end(); ++i)
{
Foo& foo = *i;
if (foo.somethingIsTrue()) // True for most elements
std::cout << i - fooVec.begin() << ": " << foo << std::endl;
}
與距離方法相比,上行方法是你不會錯誤地為非random_access_iterator做這個,所以你總是在O(1)中 。
對於具體問題:
Is there a better or more elegant way to iterate over STL vectors when both reference to the vector element and its index is required?
恕我直言,
for (size_t i = 0; i < fooVec.size(); ++i) {
Foo & foo = fooVec[i]; // if 'foo' is to be modified
Foo const& foo = fooVec[i]; // if 'foo' is to be NOT modified
}
是最簡單,最優雅的解決方案。 根據問題的要求,不需要使用迭代器。
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.