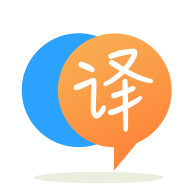
[英]I would like to store all command-line arguments to a Bash script into a single variable
[英]bash: store all command-line args after all parameters
如何創建這個小腳本?
例如:
~$ script.sh -b my small string... other things -a other string -c any other string ant etc
我只想要字符串,每個都有一個模式。
-b
my small string... other things
-a
other string
-c
any other string ant etc
有人知道如何實現嗎?
謝謝
這是一個非常簡單的命令行參數循環。 命令行參數為$1
, $2
等,命令行參數為$#
。 在使用完參數后, shift
命令將其丟棄。
#!/bin/bash
while [[ $# -gt 0 ]]; do
case "$1" in
-a) echo "option $1, argument: $2"; shift 2;;
-b) echo "option $1, argument: $2"; shift 2;;
-c) echo "option $1, argument: $2"; shift 2;;
-*) echo "unknown option: $1"; shift;;
*) echo "$1"; shift;;
esac
done
UNIX命令通常期望您自己引用多字參數,因此它們顯示為單個參數。 用法如下所示:
~$ script.sh -b 'my small string... other things' -a 'other string' -c 'any other string ant etc'
option -b, argument: my small string... other things
option -a, argument: other string
option -c, argument: any other string ant etc
注意我是如何引用長參數的。
我不建議這樣做,但是,如果您確實想在命令行中傳遞多個單詞,但將它們視為單個參數,則需要一些更復雜的操作:
#!/bin/bash
while [[ $# -gt 0 ]]; do
case "$1" in
-a) echo "option: $1"; shift;;
-b) echo "option: $1"; shift;;
-c) echo "option: $1"; shift;;
-*) echo "unknown option: $1"; shift;;
*) # Concatenate arguments until we find the next `-x' option.
OTHER=()
while [[ $# -gt 0 && ! ( $1 =~ ^- ) ]]; do
OTHER+=("$1")
shift
done
echo "${OTHER[@]}"
esac
done
用法示例:
~$ script.sh -b my small string... other things -a other string -c any other string ant etc
option: -b
my small string... other things
option: -a
other string
option: -c
any other string ant etc
同樣,不建議這種用法。 這樣做會違反UNIX規范和約定來連接參數。
我考慮使用getopt
進行此操作,但我認為它沒有能力。 將未加引號的字符串作為一個參數是非常不尋常的。 我認為您將不得不手動執行此操作; 例如:
long_str=""
for i; do
if [ ${i:0:1} = '-' ]; then
[ -z "$long_str" ] || echo ${long_str:1}
long_str=""
echo $i
else
long_str="$long_str $i"
fi
done
[ -z "$long_str" ] || echo ${long_str:1}
您應該考慮引用傳遞給腳本的參數:
例如:
展覽A:
script.sh -a one string here -b another string here
圖表B:
script.sh -a "one string here" -b "another string here"
和script.sh:
echo "$1:$2:$3:$4"
對於展覽A,腳本將顯示:-a:one:string:here
對於展覽B,腳本將顯示:-a:此處為一個字符串:-b:此處為另一個字符串
我用冒號分隔事物,使其更明顯。
在Bash中,如果引用參數,則禁止對字符串進行標記化,從而將以空格分隔的字符串強制為一個標記,而不是多個標記。
附帶說明一下,您應該引用Bash中使用的每個變量,僅在其值包含令牌分隔符(空格,制表符等)的情況下,因為“ $ var”和$ var是兩個不同的東西,尤其是如果var =“帶空格的字符串”。
為什么? 因為有時您可能想要這樣的東西:
script.sh -a "a string with -b in it" -b "another string, with -a in it"
而且,如果您不使用帶引號的參數,而是嘗試使用啟發式方法來查找下一個參數的位置,則代碼在碰到偽造的-a和-b標記時就會剎車。
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.