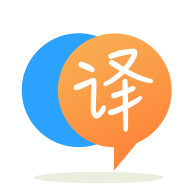
[英]Programmatically set the width and height of GridView And ImageView
[英]Set ImageView width and height programmatically?
如何以編程方式設置ImageView
的寬度和高度?
可能為時已晚,但為了其他有同樣問題的人,設置ImageView
的高度:
imageView.getLayoutParams().height = 20;
重要的。 如果您在布局已經“布局”之后設置高度,請確保您還調用:
imageView.requestLayout();
如果您的圖像視圖是動態的,則包含 getLayout 的答案將因空異常而失敗。
在這種情況下,正確的方法是:
LinearLayout.LayoutParams layoutParams = new LinearLayout.LayoutParams(100, 100);
iv.setLayoutParams(layoutParams);
這種完成任務的簡單方法:
setContentView(R.id.main);
ImageView iv = (ImageView) findViewById(R.id.left);
int width = 60;
int height = 60;
LinearLayout.LayoutParams parms = new LinearLayout.LayoutParams(width,height);
iv.setLayoutParams(parms);
和另一種方式,如果你想在高度和寬度上給出屏幕尺寸,然后使用下面的代碼:
setContentView(R.id.main);
Display display = getWindowManager().getDefaultDisplay();
ImageView iv = (LinearLayout) findViewById(R.id.left);
int width = display.getWidth(); // ((display.getWidth()*20)/100)
int height = display.getHeight();// ((display.getHeight()*30)/100)
LinearLayout.LayoutParams parms = new LinearLayout.LayoutParams(width,height);
iv.setLayoutParams(parms);
希望對你充分利用。
我用pixel
和dp
玩過這個。
private int dimensionInPixel = 200;
如何按像素設置:
view.getLayoutParams().height = dimensionInPixel;
view.getLayoutParams().width = dimensionInPixel;
view.requestLayout();
如何通過dp設置:
int dimensionInDp = (int) TypedValue.applyDimension(TypedValue.COMPLEX_UNIT_DIP, dimensionInPixel, getResources().getDisplayMetrics());
view.getLayoutParams().height = dimensionInDp;
view.getLayoutParams().width = dimensionInDp;
view.requestLayout();
希望這會幫助你。
如果您需要將寬度或高度設置為match_parent
(與其父級一樣大)或wrap_content
(大到足以容納其內部內容),則ViewGroup.LayoutParams
具有以下兩個常量:
imageView.setLayoutParams(
new ViewGroup.LayoutParams(
// or ViewGroup.LayoutParams.WRAP_CONTENT
ViewGroup.LayoutParams.MATCH_PARENT,
// or ViewGroup.LayoutParams.WRAP_CONTENT,
ViewGroup.LayoutParams.MATCH_PARENT ) );
或者您可以像在 Hakem Zaied 的回答中一樣設置它們:
imageView.getLayoutParams().width = ViewGroup.LayoutParams.MATCH_PARENT;
//...
您可以為所有情況設置值。
demoImage.getLayoutParams().height = 150;
demoImage.getLayoutParams().width = 150;
demoImage.setScaleType(ImageView.ScaleType.FIT_XY);
動態設置按鈕的最佳和最簡單的方法是
Button index=new Button(this);
int height = (int) TypedValue.applyDimension(TypedValue.COMPLEX_UNIT_DIP, 45, getResources().getDisplayMetrics());
int width = (int) TypedValue.applyDimension(TypedValue.COMPLEX_UNIT_DIP, 42, getResources().getDisplayMetrics());
上面的高度和寬度以像素為單位px 。 45 是dp的高度,42 是dp的寬度。
index.setLayoutParams(new <Parent>.LayoutParams(width, height));
因此,例如,如果您已將按鈕放置在 TableLayout 內的 TableRow 中,則應將其作為 TableRow.LayoutParams
index.setLayoutParams(new TableRow.LayoutParams(width, height));
只需創建一個 LayoutParams 對象並將其分配給您的 imageView
LinearLayout.LayoutParams params = new LinearLayout.LayoutParams(150, 150);
imageView.setLayoutParams(params);
image_view.getLayoutParams().height
以像素為單位返回高度值。 您需要先獲取屏幕尺寸才能正確設置值。
Display display = context.getWindowManager().getDefaultDisplay();
DisplayMetrics outMetrics = new DisplayMetrics();
display.getMetrics(outMetrics);
int screen_height = outMetrics.heightPixels;
int screen_width = outMetrics.widthPixels;
獲得屏幕尺寸后,您可以使用適當的邊距設置圖像視圖的寬度和高度。
view.getLayoutParams().height = screen_height - 140;
view.getLayoutParams().width = screen_width - 130 ;
為了以編程方式設置 ImageView 和高度,您可以執行
//Makesure you calculate the density pixel and multiply it with the size of width/height
float dpCalculation = getResources().getDisplayMetrics().density;
your_imageview.getLayoutParams().width = (int) (150 * dpCalculation);
//Set ScaleType according to your choice...
your_imageview.setScaleType(ImageView.ScaleType.CENTER_CROP);
科特林
val density = Resources.getSystem().displayMetrics.density
view.layoutParams.height = 20 * density.toInt()
image.setLayoutParams(new ViewGroup.LayoutParams(width, height));
例子:
image.setLayoutParams(new ViewGroup.LayoutParams(150, 150));
在需要以編程方式設置小部件大小的場景中,請確保以下規則。
LayoutParam
。 在我的情況下,我添加到TableRow
所以我不得不做TableRow.LayoutParams
final float scale = getResources().getDisplayMetrics().density; int dpWidthInPx = (int) (17 * scale); int dpHeightInPx = (int) (17 * scale);
TableRow.LayoutParams deletelayoutParams = new TableRow.LayoutParams(dpWidthInPx, dpHeightInPx); button.setLayoutParams(deletelayoutParams); tableRow.addView(button, 1);
如果您只想在圖像視圖中適合圖像,您可以在高度和寬度屬性中使用“包裹內容”和比例類型,但如果您想手動設置,則必須使用 LayoutParams。
Layoutparams 對於以編程方式設置布局高度和寬度非常有效。
int newHeight = 150;
int newWidth = 150;
holder.iv_arrow.requestLayout();
holder.iv_arrow.getLayoutParams().height = newHeight;
holder.iv_arrow.getLayoutParams().width = newWidth;
holder.iv_arrow.setScaleType(ImageView.ScaleType.FIT_XY);
holder.iv_arrow.setImageResource(R.drawable.video_menu);
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.