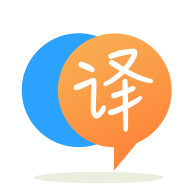
[英]How can I add a $key=>$value pair to an associative array using a foreach loop in PHP?
[英]PHP - when using foreach to loop through array, how can I tell if I'm on the last pair?
我正在嘗試使用如下語法'漂亮'打印出一個數組:
$outString = "[";
foreach($arr as $key=>$value) {
// Do stuff with the key + value, putting the result in $outString.
$outString .= ", ";
}
$outString .= "]";
然而,這種方法的明顯缺點是它會在數組打印結束時,在結束“]之前顯示”,“。 有沒有一種好方法使用$ key => $ value語法來檢測你是否在數組中的最后一對,或者我應該使用each()
來切換到循環?
構建一個數組,然后使用implode
:
$parts = array();
foreach($arr as $key=>$value) {
$parts[] = process($key, $value);
}
$outString = "[". implode(", ", $parts) . "]";
您可以通過使用子字符串來修剪最后一個“,”。
$outString = "[";
foreach($arr as $key=>$value) {
// Do stuff with the key + value, putting the result in $outString.
$outString .= ", ";
}
$outString = substr($outString,-2)."]"; //trim off last ", "
單獨進行處理,然后使用implode()
加入:
$outArr = array();
foreach($arr as $key => $value) {
$outArr[] = process($key, $value);
}
$outString = '[' . implode(', ', $outArr) . ']';
盡管你的例子的情況下(加入用逗號串,為此爆是去了解它的正確方法),來回答您的具體問題,規范的方式,通過數組進行迭代和檢查,如果你是最后一個元素是使用CachingIterator :
<?php
$array = array('k'=>'v', 'k1'=>'v1');
$iter = new CachingIterator(new ArrayIterator($array));
$out = '';
foreach ($iter as $key=>$value) {
$out .= $value;
// CachingIterator->hasNext() tells you if there is another
// value after the current one.
if ($iter->hasNext()) {
$out .= ', ';
}
}
echo $out;
這樣做可能更容易,如果它不是第一個項目,則在項目之前為字符串添加逗號。
$first= true;
foreach ($arr as $key => $value) {
if (! $first) {
$outString .=', ';
} else {
$first = false;
}
//add stuff to $outString
}
輸出格式是JSON嗎? 如果是這樣,你可以考慮使用json_encode()
代替。
您可以將其作為正常的循環:
$outString = "[";
for ($i = 0; $i < count($arr); $i++) {
// Do stuff
if ($i != count($arr) - 1) {
$outString += ", ";
}
}
然而,最好的方法,恕我直言,將所有組件存儲在一個數組中,然后插入:
$outString = '[' . implode(', ', $arr) . ']';
implode()獲取數組中的所有元素,並將它們放在一個字符串中,由第一個參數分隔。
有很多種可能性。 我認為這是最簡單的解決方案之一:
$arr = array(0 => 'a', 1 => 'b', 2 => 'c', 3 => 'd', 4 => 'e', 5 => 'f', 6 => 'g');
echo '[', current($arr);
while ($n = next($arr)) echo ',', $n;
echo ']';
輸出:
[a,b,c,d,e,f,g]
我傾向於避免在諸如此類的情況下使用循環。 您應該使用implode()來連接具有公共分隔符的列表,並使用array_map()在連接之前處理該數組上的任何處理。 請注意以下實現應表達這些功能的多功能性。 數組映射可以采用表示內置函數或用戶定義函數的字符串(函數名稱)(前3個示例)。 您可以使用create_function()傳遞一個函數,或者傳遞一個lambda / anonymous函數作為第一個參數。
$a = array(1, 2, 3, 4, 5);
// Using the user defined function
function fn ($n) { return $n * $n; }
printf('[%s]', implode(', ', array_map('fn', $a)));
// Outputs: [1, 4, 9, 16, 25]
// Using built in htmlentities (passing additional parameter
printf('[%s]', implode(', ', array_map( 'intval' , $a)));
// Outputs: [1, 2, 3, 4, 5]
// Using built in htmlentities (passing additional parameter
$b = array('"php"', '"&<>');
printf('[%s]', implode(', ', array_map( 'htmlentities' , $b, array_fill(0 , count($b) , ENT_QUOTES) )));
// Outputs: ["php", "&<>]
// Using create_function <PHP 5
printf('[%s]', implode(', ', array_map(create_function('$n', 'return $n + $n;'), $a)));
// Outputs: [2, 4, 6, 8, 10]
// Using a lambda function (PHP 5.3.0+)
printf('[%s]', implode(', ', array_map(function($n) { return $n; }, $a)));
// Outputs: [1, 2, 3, 4, 5]
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.