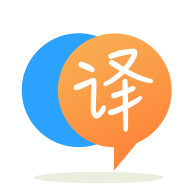
[英]whitespace before or after characters, symbols, or numbers; but, not in-between
[英]Logic for checking in-between two numbers
我想確定區域的任意編號。
zones = [0, 150, 300, 400, 600, 800]
function checkZone(mouseX) {
// if mouseX is 321, it should be index 2 of zones array
}
您可以使用以下循環來實現。 我已經包括了整個頁面用於測試。
<html>
<body>
<script type="text/javascript">
var i; var y = 0; var val = 321; var zones = [0,150,300,400,600,800];
for (i = 0; i < zones.length; i++)
if (val >= zones[i])
y = i;
document.write("Value " + val + " is at position " + y);
</script>
</body>
</html>
使用各種測試數據:
Value -99 is at position 0
Value 0 is at position 0
Value 149 is at position 0
Value 150 is at position 1
Value 321 is at position 2
Value 521 is at position 3
Value 799 is at position 4
Value 800 is at position 5
Value 999 is at position 5
如果數組很大,檢查每個元素將很慢。 在這種情況下,二進制搜索可能會很好。 這是一些示例代碼(未經測試,但在夜間的這個時間也要深思熟慮):
function checkZone(mouseX) {
var low = 0, high = zones.length, i;
if (mouseX >= zones[high]) {
low = high
}
while (high - low > 1) {
i = low + Math.floor((high - low) / 2);
if (mouseX >= zones[i]) {
low = i;
} else {
high = i;
}
}
return zones[low];
}
注意 :我嘗試在大約15分鍾前發布此內容,但是我吃了我的答案,所以我不得不重做大部分內容。
數字數組是否按升序排序? 如果是,那么您有兩個選擇。 如果數組的大小相對較小,則只需循環遍歷它,然后找到合適的區域即可。 否則,執行二進制搜索(快得多)。
這應該更好,更快:
console.clear();
var zones = [800, 400, 150, 0, 300, 600]; // unsorted array
zones.sort(); // sort for function comparison
console.log(zones);
function checkZone(mouseX) {
for( var i = (zones.length-1); mouseX < zones[i--];){ }
return ++i;
}
// perform 25 tests with random mouseX values 0-1000
for (var rnd=1,runs=25; runs-- && (rnd=Math.floor( Math.random()*1000 ))>=0; ){
console.log( (25-runs) + ". "
+ "mouseX:" + rnd + " , "
+ "index:" + checkZone(rnd) // checkZone(number) is all that's really needed
);
}
函數中的for循環從最后一個數組值到開頭搜索數組。 一旦參數不再小於數組值,則退出循環,函數返回該元素。 我們必須在返回變量中加1,因為當循環條件失敗時,循環不會加1。
如果您對console.log
感到不滿意,可以用document.write
或alert
替換它。 完成測試后,只需要排序數組,函數和函數調用。 您可以取消測試循環以及所有隨機數jibber-jabber。
輸出示例:
[0, 150, 300, 400, 600, 800]
1. mouseX:458 , index:3
2. mouseX:17 , index:0
3. mouseX:377 , index:2
4. mouseX:253 , index:1
5. mouseX:446 , index:3
6. mouseX:764 , index:4
7. mouseX:619 , index:4
8. mouseX:653 , index:4
9. mouseX:337 , index:2
10. mouseX:396 , index:2
11. mouseX:107 , index:0
12. mouseX:820 , index:5
13. mouseX:850 , index:5
14. mouseX:117 , index:0
15. mouseX:659 , index:4
16. mouseX:393 , index:2
17. mouseX:906 , index:5
18. mouseX:128 , index:0
19. mouseX:435 , index:3
20. mouseX:712 , index:4
21. mouseX:841 , index:5
22. mouseX:259 , index:1
23. mouseX:447 , index:3
24. mouseX:809 , index:5
25. mouseX:892 , index:5
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.