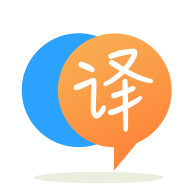
[英]Java Thread Sleep exactly specific amount of time (including some process)
[英]How to make a thread sleep for specific amount of time in java?
我有一個場景,我希望一個線程睡眠特定的時間。
碼:
public void run(){
try{
//do something
Thread.sleep(3000);
//do something after waking up
}catch(InterruptedException e){
// interrupted exception hit before the sleep time is completed.so how do i make my thread sleep for exactly 3 seconds?
}
}
現在我如何處理我試圖運行的線程在完成睡眠之前被中斷的異常命中的情況? 線程在被中斷后喚醒並且它是否進入可運行狀態,或者只有在它進入runnable之后它才會進入catch塊?
當你的線程被中斷命中時,它將進入InterruptedException
catch塊。 然后,您可以檢查線程花費多長時間睡眠並計算出睡眠時間。 最后,不要吞下異常,最好還原中斷狀態,以便調用堆棧上方的代碼可以處理它。
public void run(){
//do something
//sleep for 3000ms (approx)
long timeToSleep = 3000;
long start, end, slept;
boolean interrupted;
while(timeToSleep > 0){
start=System.currentTimeMillis();
try{
Thread.sleep(timeToSleep);
break;
}
catch(InterruptedException e){
//work out how much more time to sleep for
end=System.currentTimeMillis();
slept=end-start;
timeToSleep-=slept;
interrupted=true
}
}
if(interrupted){
//restore interruption before exit
Thread.currentThread().interrupt();
}
}
我用這種方式:
因此沒有必要等待特定時間結束。
public void run(){
try {
//do something
try{Thread.sleep(3000);}catch(Exception e){}
//do something
}catch(Exception e){}
}
試試這個:
public void run(){ try{ //do something long before = System.currentTimeMillis(); Thread.sleep(3000); //do something after waking up }catch(InterruptedException e){ long diff = System.currentTimeMillis()-before; //this is approximation! exception handlers take time too.... if(diff < 3000) //do something else, maybe go back to sleep. // interrupted exception hit before the sleep time is completed.so how do i make my thread sleep for exactly 3 seconds? } }
如果你不打擾自己的睡眠,為什么這個線程會被喚醒? 似乎你做的事情非常錯誤......
根據此頁面,您必須對其進行編碼以按您希望的方式運行。 使用睡眠上方的線程將被中斷,您的線程將退出。 理想情況下,您將重新拋出異常,以便啟動線程時可以采取適當的操作。
如果您不希望這種情況發生,您可以將整個事情放在一個while(true)循環中。 現在,當中斷發生時,睡眠中斷,你吃異常,然后循環開始新的睡眠。
如果你想完成3秒的睡眠,你可以通過比較10次300毫秒的睡眠來近似它,並將循環計數器保持在while循環之外。 當你看到中斷時,吃掉它,設置一個“我必須死”的標志,然后繼續循環,直到你睡得足夠。 然后以受控方式中斷線程。
這是一種方式:
public class ThreadThing implements Runnable {
public void run() {
boolean sawException = false;
for (int i = 0; i < 10; i++) {
try {
//do something
Thread.sleep(300);
//do something after waking up
} catch (InterruptedException e) {
// We lose some up to 300 ms of sleep each time this
// happens... This can be tuned by making more iterations
// of lesser duration. Or adding 150 ms back to a 'sleep
// pool' etc. There are many ways to approximate 3 seconds.
sawException = true;
}
}
if (sawException) Thread.currentThread().interrupt();
}
}
你為什么要睡3秒鍾? 如果只是在一段時間后執行某些操作,請嘗試使用Timer 。
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.