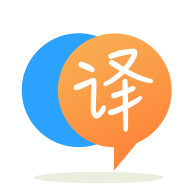
[英]How do I join a user-entered string to three arrays and print the result?
[英]How do I read a string entered by the user in C?
我想讀取用戶使用 C 程序輸入的名稱。
為此,我寫道:
char name[20];
printf("Enter name: ");
gets(name);
但是使用gets
並不好,那么有什么更好的方法呢?
你永遠不應該使用gets
(或具有無限字符串大小的scanf
),因為這會導致緩沖區溢出。 使用帶有stdin
句柄的fgets
,因為它允許您限制將放置在緩沖區中的數據。
這是我用於用戶行輸入的一個小片段:
#include <stdio.h>
#include <string.h>
#define OK 0
#define NO_INPUT 1
#define TOO_LONG 2
static int getLine (char *prmpt, char *buff, size_t sz) {
int ch, extra;
// Get line with buffer overrun protection.
if (prmpt != NULL) {
printf ("%s", prmpt);
fflush (stdout);
}
if (fgets (buff, sz, stdin) == NULL)
return NO_INPUT;
// If it was too long, there'll be no newline. In that case, we flush
// to end of line so that excess doesn't affect the next call.
if (buff[strlen(buff)-1] != '\n') {
extra = 0;
while (((ch = getchar()) != '\n') && (ch != EOF))
extra = 1;
return (extra == 1) ? TOO_LONG : OK;
}
// Otherwise remove newline and give string back to caller.
buff[strlen(buff)-1] = '\0';
return OK;
}
這允許我設置最大大小,將檢測是否在行上輸入了太多數據,並將刷新行的其余部分,因此它不會影響下一個輸入操作。
您可以使用以下方法對其進行測試:
// Test program for getLine().
int main (void) {
int rc;
char buff[10];
rc = getLine ("Enter string> ", buff, sizeof(buff));
if (rc == NO_INPUT) {
// Extra NL since my system doesn't output that on EOF.
printf ("\nNo input\n");
return 1;
}
if (rc == TOO_LONG) {
printf ("Input too long [%s]\n", buff);
return 1;
}
printf ("OK [%s]\n", buff);
return 0;
}
我認為讀取用戶輸入的字符串的最佳和最安全的方法是使用getline()
這是一個如何執行此操作的示例:
#include <stdio.h>
#include <stdlib.h>
int main(int argc, char *argv[])
{
char *buffer = NULL;
int read;
unsigned int len;
read = getline(&buffer, &len, stdin);
if (-1 != read)
puts(buffer);
else
printf("No line read...\n");
printf("Size read: %d\n Len: %d\n", read, len);
free(buffer);
return 0;
}
在 POSIX 系統上,如果可用,您可能應該使用getline
。
您還可以使用 Chuck Falconer 的公共領域ggets
函數,該函數提供更gets
get 的語法但沒有問題。 (Chuck Falconer 的網站不再可用,盡管archive.org 有一個副本,而且我已經為 ggets 制作了自己的頁面。)
我找到了一個簡單而好的解決方案:
char*string_acquire(char*s,int size,FILE*stream){
int i;
fgets(s,size,stream);
i=strlen(s)-1;
if(s[i]!='\n') while(getchar()!='\n');
if(s[i]=='\n') s[i]='\0';
return s;
}
它基於 fgets,但沒有 '\n' 和 stdin 額外字符(替換 fflush(stdin) 不適用於所有操作系統,如果在此之后必須獲取字符串,則很有用)。
在 BSD 系統和 Android 上,您還可以使用fgetln
:
#include <stdio.h>
char *
fgetln(FILE *stream, size_t *len);
像這樣:
size_t line_len;
const char *line = fgetln(stdin, &line_len);
該line
不是以空值結尾的,最后包含\n
(或您的平台使用的任何內容)。 在流上的下一個 I/O 操作后它變得無效。 您可以修改返回的line
緩沖區。
使用scanf
在鍵入字符串之前刪除所有空格並限制要讀取的字符數量:
#define SIZE 100
....
char str[SIZE];
scanf(" %99[^\n]", str);
/* Or even you can do it like this */
scanf(" %99[a-zA-Z0-9 ]", str);
如果你不限制使用scanf
讀取的字符數量,它可能和 get 一樣gets
ANSI C 未知最大長度解
只需從 Johannes Schaub 的https://stackoverflow.com/a/314422/895245復制
完成后不要忘記free
返回的指針。
char * getline(void) {
char * line = malloc(100), * linep = line;
size_t lenmax = 100, len = lenmax;
int c;
if(line == NULL)
return NULL;
for(;;) {
c = fgetc(stdin);
if(c == EOF)
break;
if(--len == 0) {
len = lenmax;
char * linen = realloc(linep, lenmax *= 2);
if(linen == NULL) {
free(linep);
return NULL;
}
line = linen + (line - linep);
linep = linen;
}
if((*line++ = c) == '\n')
break;
}
*line = '\0';
return linep;
}
此代碼使用malloc
分配 100 個字符。 然后它從用戶那里逐個字符地獲取字符。 如果用戶達到 101 個字符,它會使用realloc
將緩沖區加倍到 200。當達到 201 時,它會再次加倍到 400,依此類推,直到內存耗盡。
我們加倍而不是說,每次只增加 100 的原因是,使用realloc
增加緩沖區的大小會導致舊緩沖區的副本,這是一個潛在的昂貴操作。
數組在內存中必須是連續的,因為我們希望能夠通過內存地址有效地隨機訪問它們。 因此,如果我們在 RAM 中有:
content buffer[0] | buffer[1] | ... | buffer[99] | empty | empty | int i
RAM address 1000 | 1001 | | 1100 | 1101 | 1102 | 1103
我們不能只增加buffer
的大小,因為它會覆蓋我們的int i
。 所以realloc
需要在內存中找到另一個有 200 個空閑字節的位置,然后將舊的 100 個字節復制到那里並釋放 100 個舊字節。
通過加倍而不是相加,我們很快達到了當前字符串大小的數量級,因為指數增長非常快,所以只完成了合理數量的副本。
您可以使用 scanf 函數讀取字符串
scanf("%[^\n]",name);
我不知道接收字符串的其他更好的選擇,
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.