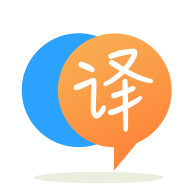
[英]How to scroll to top of page with JavaScript/jQuery on refresh and exclude specific term?
[英]How to scroll to top of page with JavaScript/jQuery?
有沒有辦法用 JavaScript/jQuery 控制瀏覽器滾動?
當我將頁面向下滾動一半,然后觸發重新加載時,我希望頁面打包到頂部,但它會嘗試找到最后一個滾動位置。 所以我這樣做了:
$('document').ready(function() {
$(window).scrollTop(0);
});
但沒有運氣。
編輯:
所以當我在頁面加載后給他們打電話時,你的兩個答案都有效 - 謝謝。 但是,如果我只是在頁面上刷新,看起來瀏覽器會在.ready
事件之后計算並滾動到其舊的滾動位置(我也測試了主體 onload() 函數)。
所以后續是,有沒有辦法阻止瀏覽器滾動到它過去的位置,或者在它完成它的事情后重新滾動到頂部?
跨瀏覽器的純 JavaScript 解決方案:
document.body.scrollTop = document.documentElement.scrollTop = 0;
你幾乎明白了 - 你需要在body
上設置scrollTop
,而不是window
:
$(function() {
$('body').scrollTop(0);
});
編輯:
也許您可以在頁面頂部添加一個空白錨點:
$(function() {
$('<a name="top"/>').insertBefore($('body').children().eq(0));
window.location.hash = 'top';
});
哇,我問這個問題晚了 9 年。 干得好:
將此代碼添加到您的 onload.xml 文件中。
// This prevents the page from scrolling down to where it was previously.
if ('scrollRestoration' in history) {
history.scrollRestoration = 'manual';
}
// This is needed if the user scrolls down during page load and you want to make sure the page is scrolled to the top once it's fully loaded. This has Cross-browser support.
window.scrollTo(0,0);
要在窗口加載時運行它,只需像這樣將它包裝起來(假設您引用了JQuery )
$(function() {
// put the code here
});
history.scrollRestoration 瀏覽器支持:
Chrome:支持(自 46 起)
Firefox:支持(自 46 起)
邊緣:支持(自 79 起)
IE:不支持
Opera:支持(自 33 起)
Safari:支持
對於IE,如果您想在它自動向下滾動后重新滾動到頂部,那么這對我有用:
var isIE11 = !!window.MSInputMethodContext && !!document.documentMode;
if(isIE11) {
setTimeout(function(){ window.scrollTo(0, 0); }, 300); // adjust time according to your page. The better solution would be to possibly tie into some event and trigger once the autoscrolling goes to the top.
}
有沒有辦法阻止瀏覽器滾動到它過去的位置,或者在它完成它的事情后重新滾動到頂部?
以下 jquery 解決方案對我有用:
$(window).unload(function() {
$('body').scrollTop(0);
});
這是一個純 JavaScript 動畫滾動版本,適用於非 jQuery 用戶:D
var stepTime = 20;
var docBody = document.body;
var focElem = document.documentElement;
var scrollAnimationStep = function (initPos, stepAmount) {
var newPos = initPos - stepAmount > 0 ? initPos - stepAmount : 0;
docBody.scrollTop = focElem.scrollTop = newPos;
newPos && setTimeout(function () {
scrollAnimationStep(newPos, stepAmount);
}, stepTime);
}
var scrollTopAnimated = function (speed) {
var topOffset = docBody.scrollTop || focElem.scrollTop;
var stepAmount = topOffset;
speed && (stepAmount = (topOffset * stepTime)/speed);
scrollAnimationStep(topOffset, stepAmount);
};
進而:
<button onclick="scrollTopAnimated(1000)">Scroll Top</button>
更新
現在在 javascript 中使用滾動效果轉到頁面頂部更容易一些:
https://developer.mozilla.org/en-US/docs/Web/API/Window/scroll
有兩種使用scroll API
。
這是我推薦的方法。 使用選項對象:
window.scroll(options)
這是一個更好的選擇,因為您可以定義一個應用內置緩動動畫的behavior
道具。
window.scroll({
top: 0,
left: 0,
behavior: 'smooth'
});
另一種方法是使用 x 和 y 坐標。
window.scroll(x-coord, y-coord)
x-coord - 是要在左上角顯示的文檔水平軸上的像素。
y-coord - 是要在左上角顯示的文檔垂直軸上的像素。
舊答案請勿使用
這是我們的原生javascript
實現。 它具有簡單的緩動效果,因此用戶在單擊“頂部”按鈕后不會感到震驚。
它非常小,縮小后變得更小。 正在尋找 jquery 方法的替代方法但希望獲得相同結果的開發人員可以試試這個。
JS
document.querySelector("#to-top").addEventListener("click", function(){
var toTopInterval = setInterval(function(){
var supportedScrollTop = document.body.scrollTop > 0 ? document.body : document.documentElement;
if (supportedScrollTop.scrollTop > 0) {
supportedScrollTop.scrollTop = supportedScrollTop.scrollTop - 50;
}
if (supportedScrollTop.scrollTop < 1) {
clearInterval(toTopInterval);
}
}, 10);
},false);
HTML
<button id="to-top">To Top</button>
干杯!
您可以與 jQuery 一起使用
jQuery(window).load(function(){
jQuery("html,body").animate({scrollTop: 100}, 1000);
});
這對我有用:
window.onload = function() {
// short timeout
setTimeout(function() {
$(document.body).scrollTop(0);
}, 15);
};
在onload
使用短的setTimeout
使瀏覽器有機會進行滾動。
使用以下功能
window.scrollTo(xpos, ypos)
這里 xpos 是必需的。 沿 x 軸(水平)滾動到的坐標,以像素為單位
ypos 也是必需的。 沿 y 軸(垂直)滾動到的坐標,以像素為單位
$(function() {
// the element inside of which we want to scroll
var $elem = $('#content');
// show the buttons
$('#nav_up').fadeIn('slow');
$('#nav_down').fadeIn('slow');
// whenever we scroll fade out both buttons
$(window).bind('scrollstart', function(){
$('#nav_up,#nav_down').stop().animate({'opacity':'0.2'});
});
// ... and whenever we stop scrolling fade in both buttons
$(window).bind('scrollstop', function(){
$('#nav_up,#nav_down').stop().animate({'opacity':'1'});
});
// clicking the "down" button will make the page scroll to the $elem's height
$('#nav_down').click(
function (e) {
$('html, body').animate({scrollTop: $elem.height()}, 800);
}
);
// clicking the "up" button will make the page scroll to the top of the page
$('#nav_up').click(
function (e) {
$('html, body').animate({scrollTop: '0px'}, 800);
}
);
});
用這個
我的純(動畫)Javascript 解決方案:
function gototop() {
if (window.scrollY>0) {
window.scrollTo(0,window.scrollY-20)
setTimeout("gototop()",10)
}
}
解釋:
window.scrollY
是一個由瀏覽器維護的變量,表示窗口已滾動的距離頂部的像素量。
window.scrollTo(x,y)
是一個函數,它在 x 軸和 y 軸上滾動窗口特定數量的像素。
因此, window.scrollTo(0,window.scrollY-20)
將頁面向上移動 20 個像素。
setTimeout
在 10 毫秒后再次調用該函數,以便我們可以將它再移動 20 個像素(動畫),並且if
語句檢查我們是否仍然需要滾動。
以下代碼適用於 Firefox、Chrome 和Safari ,但我無法在 Internet Explorer 中對此進行測試。 有人可以測試它,然后編輯我的答案或對其發表評論嗎?
$(document).scrollTop(0);
在我的情況下,身體不起作用:
$('body').scrollTop(0);
但 HTML 有效:
$('html').scrollTop(0);
跨瀏覽器滾動到頂部:
if($('body').scrollTop()>0){
$('body').scrollTop(0); //Chrome,Safari
}else{
if($('html').scrollTop()>0){ //IE, FF
$('html').scrollTop(0);
}
}
跨瀏覽器滾動到 id = div_id 的元素:
if($('body').scrollTop()>$('#div_id').offset().top){
$('body').scrollTop($('#div_id').offset().top); //Chrome,Safari
}else{
if($('html').scrollTop()>$('#div_id').offset().top){ //IE, FF
$('html').scrollTop($('#div_id').offset().top);
}
}
為什么不在 html 文件的開頭使用一些參考元素,例如
<div id="top"></div>
然后,當頁面加載時,只需執行
$(document).ready(function(){
top.location.href = '#top';
});
如果瀏覽器在此功能觸發后滾動,您只需執行
$(window).load(function(){
top.location.href = '#top';
});
如果您處於怪癖模式(感謝@Niet the Dark Absol):
document.body.scrollTop = document.documentElement.scrollTop = 0;
如果您處於嚴格模式:
document.documentElement.scrollTop = 0;
這里不需要 jQuery。
這是有效的:
jQuery(document).ready(function() {
jQuery("html").animate({ scrollTop: 0 }, "fast");
});
要回答您編輯的問題,您可以像這樣注冊onscroll
處理程序:
document.documentElement.onscroll = document.body.onscroll = function() {
this.scrollTop = 0;
this.onscroll = null;
}
這將使第一次嘗試滾動(這可能是瀏覽器自動完成的)將被有效取消。
這兩者的結合幫助了我。 其他答案都沒有幫助我,因為我有一個沒有滾動的sidenav。
setTimeout(function () {
window.scroll({
top: 0,
left: 0,
behavior: 'smooth'
});
document.body.scrollTop = document.documentElement.scrollTop = 0;
}, 15);
var totop = $('#totop');
totop.click(function(){
$('html, body').stop(true,true).animate({scrollTop:0}, 1000);
return false;
});
$(window).scroll(function(){
if ($(this).scrollTop() > 100){
totop.fadeIn();
}else{
totop.fadeOut();
}
});
<img id="totop" src="img/arrow_up.png" title="Click to go Up" style="display:none;position:fixed;bottom:10px;right:10px;cursor:pointer;cursor:hand;"/>
沒有動畫,只是scroll(0, 0)
(香草JS)
如果有人在 sidenav 中使用角度和材料設計。 這會將您帶到頁面頂部:
let ele = document.getElementsByClassName('md-sidenav-content');
let eleArray = <Element[]>Array.prototype.slice.call(ele);
eleArray.map( val => {
val.scrollTop = document.documentElement.scrollTop = 0;
});
先在你想去的地方添加一個空白的錨標簽
<a href="#topAnchor"></a>
現在在標題部分添加一個函數
function GoToTop() {
var urllocation = location.href;
if (urllocation.indexOf("#topAnchor") > -1) {
window.location.hash = "topAnchor";
} else {
return false;
}
}
最后在body標簽中添加一個onload事件
<body onload="GoToTop()">
適用於任何 X 和 Y 值的通用版本,與 window.scrollTo api 相同,只是增加了 scrollDuration。
*與window.scrollTo瀏覽器api匹配的通用版本**
function smoothScrollTo(x, y, scrollDuration) {
x = Math.abs(x || 0);
y = Math.abs(y || 0);
scrollDuration = scrollDuration || 1500;
var currentScrollY = window.scrollY,
currentScrollX = window.scrollX,
dirY = y > currentScrollY ? 1 : -1,
dirX = x > currentScrollX ? 1 : -1,
tick = 16.6667, // 1000 / 60
scrollStep = Math.PI / ( scrollDuration / tick ),
cosParameterY = currentScrollY / 2,
cosParameterX = currentScrollX / 2,
scrollCount = 0,
scrollMargin;
function step() {
scrollCount = scrollCount + 1;
if ( window.scrollX !== x ) {
scrollMargin = cosParameterX + dirX * cosParameterX * Math.cos( scrollCount * scrollStep );
window.scrollTo( 0, ( currentScrollX - scrollMargin ) );
}
if ( window.scrollY !== y ) {
scrollMargin = cosParameterY + dirY * cosParameterY * Math.cos( scrollCount * scrollStep );
window.scrollTo( 0, ( currentScrollY - scrollMargin ) );
}
if (window.scrollX !== x || window.scrollY !== y) {
requestAnimationFrame(step);
}
}
step();
}
<script>
sessionStorage.scrollDirection = 1;//create a session variable
var pageScroll = function() {
window.scrollBy ({
top: sessionStorage.scrollDirection,
left: 0,
behavior: 'smooth'
});
if($(window).scrollTop() + $(window).height() > $(document).height() - 1)
{
sessionStorage.scrollDirection= Number(sessionStorage.scrollDirection )-300;
setTimeout(pageScroll,50);//
}
else{
sessionStorage.scrollDirection=Number(sessionStorage.scrollDirection )+1
setTimeout(pageScroll,300);
}
};
pageScroll();
</script>
我記得在其他地方看到過這個帖子(我找不到在哪里),但這真的很好用:
setTimeout(() => {
window.scrollTo(0, 0);
}, 0);
這很奇怪,但它的工作方式是基於 JavaScript 堆棧隊列的工作方式。 完整的解釋發現這里的零延遲部分。
基本思想是setTimeout
的時間實際上並沒有指定它要等待的時間量,而是它要等待的最短時間。 因此,當您告訴它等待 0 毫秒時,瀏覽器會運行所有其他排隊的進程(例如將窗口滾動到您上次所在的位置),然后執行回調。
Seeint 哈希應該可以完成這項工作。 如果您有標題,則可以使用
window.location.href = "#headerid";
否則, # 單獨會起作用
window.location.href = "#";
當它被寫入 url 時,如果你刷新它就會留下來。
實際上,如果您想在 onclick 事件上執行此操作,則不需要 JavaScript,您應該只在元素周圍放置一個鏈接,並將其指定為 # 作為 href。
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.