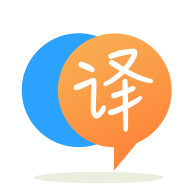
[英]Jackson Json Deserialisation: Unrecognized field “…” , not marked as ignorable
[英]Jackson with JSON: Unrecognized field, not marked as ignorable
我需要將某個 JSON 字符串轉換為 Java object。 我正在使用 Jackson 進行 JSON 處理。 我無法控制輸入 JSON(我從 web 服務讀取)。 這是我的輸入 JSON:
{"wrapper":[{"id":"13","name":"Fred"}]}
這是一個簡化的用例:
private void tryReading() {
String jsonStr = "{\"wrapper\"\:[{\"id\":\"13\",\"name\":\"Fred\"}]}";
ObjectMapper mapper = new ObjectMapper();
Wrapper wrapper = null;
try {
wrapper = mapper.readValue(jsonStr , Wrapper.class);
} catch (Exception e) {
e.printStackTrace();
}
System.out.println("wrapper = " + wrapper);
}
我的實體 class 是:
public Class Student {
private String name;
private String id;
//getters & setters for name & id here
}
我的 Wrapper class 基本上是一個容器 object 來獲取我的學生名單:
public Class Wrapper {
private List<Student> students;
//getters & setters here
}
我不斷收到此錯誤,“包裝器”返回null
。 我不確定缺少什么。 有人可以幫忙嗎?
org.codehaus.jackson.map.exc.UnrecognizedPropertyException:
Unrecognized field "wrapper" (Class Wrapper), not marked as ignorable
at [Source: java.io.StringReader@1198891; line: 1, column: 13]
(through reference chain: Wrapper["wrapper"])
at org.codehaus.jackson.map.exc.UnrecognizedPropertyException
.from(UnrecognizedPropertyException.java:53)
您可以使用 Jackson 的類級注釋:
import com.fasterxml.jackson.annotation.JsonIgnoreProperties
@JsonIgnoreProperties
class { ... }
它將忽略您尚未在 POJO 中定義的每個屬性。 當您只是在 JSON 中查找幾個屬性並且不想編寫整個映射時非常有用。 傑克遜網站上的更多信息。 如果你想忽略任何未聲明的屬性,你應該寫:
@JsonIgnoreProperties(ignoreUnknown = true)
你可以使用
ObjectMapper objectMapper = getObjectMapper();
objectMapper.configure(DeserializationFeature.FAIL_ON_UNKNOWN_PROPERTIES, false);
它將忽略所有未聲明的屬性。
第一個答案幾乎是正確的,但需要的是更改 getter 方法,而不是字段——字段是私有的(並且不會自動檢測到); 此外,如果兩者都可見,則 getter 優先於字段。(也有一些方法可以使私有字段可見,但如果您想擁有 getter,則沒有多大意義)
所以 getter 應該被命名為getWrapper()
,或者注釋為:
@JsonProperty("wrapper")
如果您更喜歡 getter 方法名稱。
使用 Jackson 2.6.0,這對我有用:
private static final ObjectMapper objectMapper =
new ObjectMapper()
.configure(DeserializationFeature.FAIL_ON_UNKNOWN_PROPERTIES, false);
並設置:
@JsonIgnoreProperties(ignoreUnknown = true)
它可以通過兩種方式實現:
標記 POJO 以忽略未知屬性
@JsonIgnoreProperties(ignoreUnknown = true)
如下配置序列化/反序列化 POJO/json 的 ObjectMapper:
ObjectMapper mapper =new ObjectMapper(); // for Jackson version 1.X mapper.configure(DeserializationConfig.Feature.FAIL_ON_UNKNOWN_PROPERTIES, false); // for Jackson version 2.X mapper.configure(DeserializationFeature.FAIL_ON_UNKNOWN_PROPERTIES, false)
這對我來說非常有效
ObjectMapper objectMapper = new ObjectMapper();
objectMapper.configure(
DeserializationConfig.Feature.FAIL_ON_UNKNOWN_PROPERTIES, false);
@JsonIgnoreProperties(ignoreUnknown = true)
注釋沒有。
添加 setter 和 getter 解決了問題,我覺得實際問題是如何解決它而不是如何抑制/忽略錯誤。 我收到錯誤“無法識別的字段.. 未標記為可忽略.. ”
盡管我在類的頂部使用了以下注釋,但它無法解析 json 對象並給我輸入
@JsonIgnoreProperties(ignoreUnknown = true)
然后我意識到我沒有添加 setter 和 getter,在將 setter 和 getter 添加到“Wrapper”和“Student”之后,它就像一個魅力。
這比 All 效果更好,請參閱此屬性。
import com.fasterxml.jackson.databind.DeserializationFeature;
import com.fasterxml.jackson.databind.ObjectMapper;
ObjectMapper objectMapper = new ObjectMapper();
objectMapper.configure(DeserializationFeature.FAIL_ON_UNKNOWN_PROPERTIES, false);
projectVO = objectMapper.readValue(yourjsonstring, Test.class);
如果您使用的是 Jackson 2.0
ObjectMapper mapper = new ObjectMapper();
mapper.disable(DeserializationFeature.FAIL_ON_UNKNOWN_PROPERTIES);
根據文檔,您可以忽略所選字段或所有未知字段:
// to prevent specified fields from being serialized or deserialized
// (i.e. not include in JSON output; or being set even if they were included)
@JsonIgnoreProperties({ "internalId", "secretKey" })
// To ignore any unknown properties in JSON input without exception:
@JsonIgnoreProperties(ignoreUnknown=true)
它使用以下代碼對我有用:
ObjectMapper mapper =new ObjectMapper();
mapper.configure(DeserializationConfig.Feature.FAIL_ON_UNKNOWN_PROPERTIES, false);
我已經嘗試了以下方法,它適用於使用 Jackson 讀取此類 JSON 格式。 使用已經建議的解決方案:使用@JsonProperty("wrapper")
注釋 getter
你的包裝類
public Class Wrapper{
private List<Student> students;
//getters & setters here
}
我對包裝類的建議
public Class Wrapper{
private StudentHelper students;
//getters & setters here
// Annotate getter
@JsonProperty("wrapper")
StudentHelper getStudents() {
return students;
}
}
public class StudentHelper {
@JsonProperty("Student")
public List<Student> students;
//CTOR, getters and setters
//NOTE: If students is private annotate getter with the annotation @JsonProperty("Student")
}
但是,這將為您提供格式的輸出:
{"wrapper":{"student":[{"id":13,"name":Fred}]}}
另請參閱https://github.com/FasterXML/jackson-annotations了解更多信息
希望這有幫助
Jackson 抱怨是因為它在您的類 Wrapper 中找不到一個名為“wrapper”的字段。 這樣做是因為您的 JSON 對象有一個名為“包裝器”的屬性。
我認為解決方法是將 Wrapper 類的字段重命名為“wrapper”而不是“students”。
此解決方案在讀取 json 流時是通用的,並且只需要獲取一些字段,而域類中未正確映射的字段可以忽略:
import org.codehaus.jackson.annotate.JsonIgnoreProperties;
@JsonIgnoreProperties(ignoreUnknown = true)
一個詳細的解決方案是使用諸如 jsonschema2pojo 之類的工具從 json 響應的架構中自動生成所需的域類,例如 Student。 您可以通過任何在線 json 到模式轉換器來完成后者。
由於 json 屬性和 java 屬性的名稱不匹配,因此將現場學生注釋如下
public Class Wrapper {
@JsonProperty("wrapper")
private List<Student> students;
//getters & setters here
}
正如沒有人提到的那樣,我以為我會......
問題是您在 JSON 中的財產被稱為“包裝器”,而您在 Wrapper.class 中的財產被稱為“學生”。
所以要么...
對我有用的是將財產公之於眾。 它為我解決了這個問題。
要么改變
public Class Wrapper {
private List<Student> students;
//getters & setters here
}
到
public Class Wrapper {
private List<Student> wrapper;
//getters & setters here
}
- - 或者 - -
將您的 JSON 字符串更改為
{"students":[{"id":"13","name":"Fred"}]}
將您的類字段設置為public而非private 。
public Class Student {
public String name;
public String id;
//getters & setters for name & id here
}
就我而言,唯一的線路
@JsonIgnoreProperties(ignoreUnknown = true)
也沒有用。
只需添加
@JsonInclude(Include.NON_EMPTY)
傑克遜 2.4.0
您的輸入
{"wrapper":[{"id":"13","name":"Fred"}]}
表示它是一個對象,有一個名為“wrapper”的字段,它是一個學生的集合。 所以我的建議是,
Wrapper = mapper.readValue(jsonStr , Wrapper.class);
其中Wrapper
定義為
class Wrapper {
List<Student> wrapper;
}
新的 Firebase Android 引入了一些巨大的變化; 在文檔副本下方:
[ https://firebase.google.com/support/guides/firebase-android] :
更新您的 Java 模型對象
與 2.x SDK 一樣,Firebase 數據庫會自動將您傳遞給DatabaseReference.setValue()
Java 對象轉換為 JSON,並且可以使用DataSnapshot.getValue()
將 JSON 讀入 Java 對象。
在新的 SDK 中,當使用DataSnapshot.getValue()
將 JSON 讀入 Java 對象時,現在默認忽略 JSON 中的未知屬性,因此您不再需要@JsonIgnoreExtraProperties(ignoreUnknown=true)
。
為了在將 Java 對象寫入 JSON 時排除字段/getter,注釋現在稱為@Exclude
而不是@JsonIgnore
。
BEFORE
@JsonIgnoreExtraProperties(ignoreUnknown=true)
public class ChatMessage {
public String name;
public String message;
@JsonIgnore
public String ignoreThisField;
}
dataSnapshot.getValue(ChatMessage.class)
AFTER
public class ChatMessage {
public String name;
public String message;
@Exclude
public String ignoreThisField;
}
dataSnapshot.getValue(ChatMessage.class)
如果您的 JSON 中有一個不在您的 Java 類中的額外屬性,您將在日志文件中看到此警告:
W/ClassMapper: No setter/field for ignoreThisProperty found on class com.firebase.migrationguide.ChatMessage
您可以通過在類上添加@IgnoreExtraProperties
注釋來消除此警告。 如果您希望 Firebase 數據庫像在 2.x SDK 中那樣運行並在存在未知屬性時拋出異常,您可以在類上添加@ThrowOnExtraProperties
注釋。
另一種可能性是 application.properties spring.jackson.deserialization.fail-on-unknown-properties=false
中的此spring.jackson.deserialization.fail-on-unknown-properties=false
,它不需要在您的應用程序中更改任何其他代碼。 當您認為合約穩定時,您可以刪除此屬性或將其標記為真。
如果由於某種原因您無法將 @JsonIgnoreProperties 注釋添加到您的類中,並且您位於 Web 服務器/容器(例如 Jetty)中。 您可以在自定義提供程序中創建和自定義 ObjectMapper
import javax.ws.rs.ext.ContextResolver;
import javax.ws.rs.ext.Provider;
import com.fasterxml.jackson.annotation.JsonInclude.Include;
import com.fasterxml.jackson.databind.DeserializationFeature;
import com.fasterxml.jackson.databind.ObjectMapper;
@Provider
public class CustomObjectMapperProvider implements ContextResolver<ObjectMapper> {
private ObjectMapper objectMapper;
@Override
public ObjectMapper getContext(final Class<?> cls) {
return getObjectMapper();
}
private synchronized ObjectMapper getObjectMapper() {
if(objectMapper == null) {
objectMapper = new ObjectMapper();
objectMapper.configure(DeserializationFeature.FAIL_ON_UNKNOWN_PROPERTIES, false);
}
return objectMapper;
}
}
這個問題已經有很多答案了。 我正在添加現有答案。
如果您想將@JsonIgnoreProperties
應用於應用@JsonIgnoreProperties
中的所有類,那么最好的方法是覆蓋 Spring Boot 默認的 jackson 對象。
在您的應用程序配置文件中定義一個 bean 來創建這樣的 jackson 對象映射器。
@Bean
public ObjectMapper getObjectMapper() {
ObjectMapper mapper = new ObjectMapper();
mapper.configure(DeserializationFeature.FAIL_ON_UNKNOWN_PROPERTIES, false);
return mapper;
}
現在,您不需要標記每個類,它會忽略所有未知屬性。
謝謝。
這對我來說非常有效
objectMapper.configure(DeserializationFeature.FAIL_ON_UNKNOWN_PROPERTIES, false);
我通過簡單地更改我的 POJO 類的 setter 和 getter 方法的簽名來解決這個問題。 我所要做的就是更改getObject方法以匹配映射器正在尋找的內容。 在我的情況下,我最初有一個getImageUrl ,但 JSON 數據有image_url ,這會導致映射器關閉。 我將我的 setter 和 getter 都更改為getImage_url 和 setImage_url 。
希望這會有所幫助。
這可能與 OP 遇到的問題不同,但如果有人遇到與我相同的錯誤,那么這將幫助他們解決問題。 當我使用來自不同依賴項的 ObjectMapper 作為 JsonProperty 注釋時,我遇到了與 OP 相同的錯誤。
這有效:
import com.fasterxml.jackson.databind.ObjectMapper;
import com.fasterxml.jackson.annotation.JsonProperty;
不起作用:
import org.codehaus.jackson.map.ObjectMapper; //org.codehaus.jackson:jackson-mapper-asl:1.8.8
import com.fasterxml.jackson.annotation.JsonProperty; //com.fasterxml.jackson.core:jackson-databind:2.2.3
不知何故,在 45 個帖子和 10 年后,沒有人為我的案例發布正確的答案。
@Data //Lombok
public class MyClass {
private int foo;
private int bar;
@JsonIgnore
public int getFoobar() {
return foo + bar;
}
}
就我而言,我們有一個名為getFoobar()
的方法,但沒有foobar
屬性(因為它是從其他屬性計算而來的)。 @JsonIgnoreProperties
上的@JsonIgnoreProperties
不起作用。
解決辦法是用@JsonIgnore
注解該方法
POJO 應定義為
響應類
public class Response {
private List<Wrapper> wrappers;
// getter and setter
}
包裝類
public class Wrapper {
private String id;
private String name;
// getters and setters
}
和映射器讀取值
Response response = mapper.readValue(jsonStr , Response.class);
我需要將某個JSON字符串轉換為Java對象。 我正在使用Jackson進行JSON處理。 我無法控制輸入的JSON(我從Web服務讀取)。 這是我輸入的JSON:
{"wrapper":[{"id":"13","name":"Fred"}]}
這是一個簡化的用例:
private void tryReading() {
String jsonStr = "{\"wrapper\"\:[{\"id\":\"13\",\"name\":\"Fred\"}]}";
ObjectMapper mapper = new ObjectMapper();
Wrapper wrapper = null;
try {
wrapper = mapper.readValue(jsonStr , Wrapper.class);
} catch (Exception e) {
e.printStackTrace();
}
System.out.println("wrapper = " + wrapper);
}
我的實體類是:
public Class Student {
private String name;
private String id;
//getters & setters for name & id here
}
我的包裝程序類基本上是一個容器對象,用於獲取我的學生列表:
public Class Wrapper {
private List<Student> students;
//getters & setters here
}
我不斷收到此錯誤,“包裝器”返回null
。 我不確定丟失了什么。 有人可以幫忙嗎?
org.codehaus.jackson.map.exc.UnrecognizedPropertyException:
Unrecognized field "wrapper" (Class Wrapper), not marked as ignorable
at [Source: java.io.StringReader@1198891; line: 1, column: 13]
(through reference chain: Wrapper["wrapper"])
at org.codehaus.jackson.map.exc.UnrecognizedPropertyException
.from(UnrecognizedPropertyException.java:53)
我需要將某個JSON字符串轉換為Java對象。 我正在使用Jackson進行JSON處理。 我無法控制輸入的JSON(我從Web服務讀取)。 這是我輸入的JSON:
{"wrapper":[{"id":"13","name":"Fred"}]}
這是一個簡化的用例:
private void tryReading() {
String jsonStr = "{\"wrapper\"\:[{\"id\":\"13\",\"name\":\"Fred\"}]}";
ObjectMapper mapper = new ObjectMapper();
Wrapper wrapper = null;
try {
wrapper = mapper.readValue(jsonStr , Wrapper.class);
} catch (Exception e) {
e.printStackTrace();
}
System.out.println("wrapper = " + wrapper);
}
我的實體類是:
public Class Student {
private String name;
private String id;
//getters & setters for name & id here
}
我的包裝程序類基本上是一個容器對象,用於獲取我的學生列表:
public Class Wrapper {
private List<Student> students;
//getters & setters here
}
我不斷收到此錯誤,“包裝器”返回null
。 我不確定丟失了什么。 有人可以幫忙嗎?
org.codehaus.jackson.map.exc.UnrecognizedPropertyException:
Unrecognized field "wrapper" (Class Wrapper), not marked as ignorable
at [Source: java.io.StringReader@1198891; line: 1, column: 13]
(through reference chain: Wrapper["wrapper"])
at org.codehaus.jackson.map.exc.UnrecognizedPropertyException
.from(UnrecognizedPropertyException.java:53)
我需要將某個JSON字符串轉換為Java對象。 我正在使用Jackson進行JSON處理。 我無法控制輸入的JSON(我從Web服務讀取)。 這是我輸入的JSON:
{"wrapper":[{"id":"13","name":"Fred"}]}
這是一個簡化的用例:
private void tryReading() {
String jsonStr = "{\"wrapper\"\:[{\"id\":\"13\",\"name\":\"Fred\"}]}";
ObjectMapper mapper = new ObjectMapper();
Wrapper wrapper = null;
try {
wrapper = mapper.readValue(jsonStr , Wrapper.class);
} catch (Exception e) {
e.printStackTrace();
}
System.out.println("wrapper = " + wrapper);
}
我的實體類是:
public Class Student {
private String name;
private String id;
//getters & setters for name & id here
}
我的包裝程序類基本上是一個容器對象,用於獲取我的學生列表:
public Class Wrapper {
private List<Student> students;
//getters & setters here
}
我不斷收到此錯誤,“包裝器”返回null
。 我不確定丟失了什么。 有人可以幫忙嗎?
org.codehaus.jackson.map.exc.UnrecognizedPropertyException:
Unrecognized field "wrapper" (Class Wrapper), not marked as ignorable
at [Source: java.io.StringReader@1198891; line: 1, column: 13]
(through reference chain: Wrapper["wrapper"])
at org.codehaus.jackson.map.exc.UnrecognizedPropertyException
.from(UnrecognizedPropertyException.java:53)
導入 com.fasterxml.jackson.annotation.JsonIgnoreProperties;
@JsonIgnoreProperties
當我們生成 getter 和 setter 時,特別是以 'is' 關鍵字開頭的,IDE 通常會刪除 'is'。 例如
private boolean isActive;
public void setActive(boolean active) {
isActive = active;
}
public isActive(){
return isActive;
}
就我而言,我只是更改了 getter 和 setter。
private boolean isActive;
public void setIsActive(boolean active) {
isActive = active;
}
public getIsActive(){
return isActive;
}
它能夠識別該領域。
就我而言,這很簡單:REST服務JSON對象已更新(添加了屬性),但REST客戶端JSON對象未更新。 一旦我更新了JSON客戶端對象,“無法識別的字段...”異常就消失了。
在我的情況下,我必須添加公共 getter 和 setter 以將字段設為私有。
ObjectMapper mapper = new ObjectMapper();
Application application = mapper.readValue(input, Application.class);
我使用 jackson-databind 2.10.0.pr3 。
以防萬一其他人像我一樣使用force-rest-api ,這是我如何使用這個討論來解決它(Kotlin):
var result = forceApi.getSObject("Account", "idhere")
result.jsonMapper.configure( DeserializationFeature.FAIL_ON_UNKNOWN_PROPERTIES, false)
val account: Account = result.`as`(Account::class.java)
看起來 force-rest-api 正在使用傑克遜的舊版本。
您只需將List的字段從“ students”更改為“ wrapper”即可,只需json文件,然后映射器將對其進行查找。
您的 json 字符串未與映射類內聯。 更改輸入字符串
String jsonStr = "{\"students\"\:[{\"id\":\"13\",\"name\":\"Fred\"}]}";
或者更改您的映射類
public class Wrapper {
private List<Student> wrapper;
//getters & setters here
}
在我的情況下,錯誤是由於以下原因造成的
最初它工作正常,然后我重命名了一個變量,在代碼中進行了更改,它給了我這個錯誤。
然后我也應用了傑克遜無知的財產,但它沒有用。
最后根據我的變量名稱重新定義我的 getter 和 setter 方法后,此錯誤已解決
所以一定要重新定義 getter 和 setter。
將 Wrapper 類更改為
public Class Wrapper {
@JsonProperty("wrapper") // add this line
private List<Student> students;
}
這樣做是將students
字段識別為 json 對象的wrapper
鍵。
此外,我個人更喜歡使用Lombok Annotations for Getter 和 Setter 作為
@Getter
@Setter
public Class Wrapper {
@JsonProperty("wrapper") // add this line
private List<Student> students;
}
由於我沒有使用 Lombok 和@JsonProperty
一起測試上述代碼,我建議您將以下代碼添加到 Wrapper 類以及覆蓋 Lombok 的默認 getter 和 setter。
public List<Student> getWrapper(){
return students;
}
public void setWrapper(List<Student> students){
this.students = students;
}
還要檢查這個以使用 Jackson 反序列化列表。
沒有 setter/getter 的最短解決方案是將@JsonProperty
添加到類字段:
public class Wrapper {
@JsonProperty
private List<Student> wrapper;
}
public class Student {
@JsonProperty
private String name;
@JsonProperty
private String id;
}
此外,您在 json 中將學生列表稱為“wrapper”,因此 Jackson 需要一個帶有名為“wrapper”的字段的類。
根據本文檔,您可以使用 Jackson2ObjectMapperBuilder 來構建您的 ObjectMapper:
@Autowired
Jackson2ObjectMapperBuilder objectBuilder;
ObjectMapper mapper = objectBuilder.build();
String json = "{\"id\": 1001}";
默認情況下,Jackson2ObjectMapperBuilder 禁用錯誤 unrecognizedpropertyexception。
json字段:
"blog_host_url": "some.site.com"
Kotlin 領域
var blogHostUrl: String = "https://google.com"
在我的情況下,我只需要在我的數據類中使用@JsonProperty
注釋。
例子:
data class DataBlogModel(
@JsonProperty("blog_host_url") var blogHostUrl: String = "https://google.com"
)
這是文章:https://www.baeldung.com/jackson-name-of-property
ObjectMapper objectMapper = new ObjectMapper()
.configure(DeserializationFeature.ACCEPT_EMPTY_ARRAY_AS_NULL_OBJECT, true);
當我的 JSON 有效負載包含 API 無法識別的屬性時,我遇到過這種情況。 解決方案是重命名/刪除有問題的屬性。
我遇到了類似的問題,唯一的區別是我使用的是 YAML 文件而不是 JSON 文件。 所以我在創建 ObjectMapper 時使用了 YamlFactory()。 此外,我的 Java POJO 的屬性是私有的。 但是,所有這些答案中提到的解決方案都沒有幫助。 我的意思是,它停止拋出錯誤並開始返回 Java 對象,但java 對象的屬性將為空。
我剛剛做了以下事情: -
objectMapper.setVisibility(PropertyAccessor.FIELD, Visibility.ANY);
並且不需要: -
objectMapper.configure(DeserializationFeature.FAIL_ON_UNKNOWN_PROPERTIES, false);
現在,我可以將 Yaml 文件中的數據反序列化為 Java 對象。 現在所有屬性都不為空。 該解決方案也適用於 JSON 方法。
下面是我試圖抑制不想解析的未知道具。 分享Kotlin代碼
val myResponse = jacksonObjectMapper().configure(DeserializationFeature.FAIL_ON_UNKNOWN_PROPERTIES, false)
.readValue(serverResponse, FooResponse::class.java)
Java 11 和更新版本的簡單解決方案:
var mapper = new ObjectMapper()
.registerModule(new JavaTimeModule())
.disable(FAIL_ON_UNKNOWN_PROPERTIES)
.disable(WRITE_DATES_AS_TIMESTAMPS)
.enable(ACCEPT_EMPTY_ARRAY_AS_NULL_OBJECT);
忽略重要的是禁用“FAIL_ON_UNKNOWN_PROPERTIES”
您需要驗證您正在解析的類的所有字段,使其與原始JSONObject
的相同。 它幫助了我,就我而言。
@JsonIgnoreProperties(ignoreUnknown = true)
根本沒有幫助。
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.