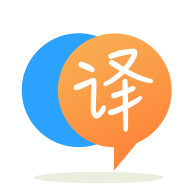
[英]Android: How to align button to the bottom and above the keyboard when it is up?
[英]Android: How can you align a button at the bottom and listview above?
我想在列表視圖的底部有一個按鈕。
如果我使用relativeLayout / FrameLayout,它會對齊,但listView會變得非常緊張。
(在底部的按鈕后面)
的FrameLayout:
<?xml version="1.0" encoding="utf-8"?>
<FrameLayout
xmlns:android="http://schemas.android.com/apk/res/android"
android:orientation="vertical"
android:layout_width="fill_parent"
android:layout_height="fill_parent">
<ListView
android:id="@+id/listview"
android:layout_width="match_parent"
android:layout_height="wrap_content"
/>
<FrameLayout
android:layout_width="wrap_content"
android:layout_height="match_parent"
android:layout_alignParentBottom="true">
<Button
android:id="@+id/btnButton"
android:text="Hello"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_gravity="bottom" />
</FrameLayout>
</FrameLayout>
RelativeLayout的:
<?xml version="1.0" encoding="utf-8"?>
<RelativeLayout
xmlns:android="http://schemas.android.com/apk/res/android"
android:orientation="vertical"
android:layout_width="fill_parent"
android:layout_height="fill_parent">
<ListView
android:id="@+id/listview"
android:layout_width="match_parent"
android:layout_height="match_parent"
/>
<RelativeLayout
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_alignParentBottom="true">
<Button
android:id="@+id/btnButton"
android:text="Hello"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_gravity="bottom" />
</RelativeLayout>
</RelativeLayout>
以上兩個代碼只能像第一張圖像一樣工作。 我想要的是第二張圖片。
有人可以幫忙嗎?
謝謝。
FrameLayout
的目的是將事物疊加在一起。 這不是你想要的。
在RelativeLayout
示例中,將ListView
的高度和寬度設置為MATCH_PARENT
這將使其占用與其父級相同的空間量,從而占用頁面上的所有空間(並覆蓋按鈕)。
嘗試類似的東西:
<LinearLayout
android:layout_width="match_parent"
android:layout_height="match_parent"
android:orientation="vertical">
<ListView
android:layout_width="match_parent"
android:layout_height="0dip"
android:layout_weight="1"/>
<Button
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_weight="0"/>
</LinearLayout>
layout_weight
指示如何使用額外空間。 Button
不想超出它所需的空間,因此它的權重為ListView
希望占用所有額外空間,因此它的權重為1。
您可以使用RelativeLayout
完成類似的操作,但如果只是這兩個項目,那么我認為LinearLayout
更簡單。
我需要在底部並排放置兩個按鈕。 我使用了水平線性布局,但為按鈕的線性布局分配android:layout_height="0dp"
和android:layout_weight="0"
不起作用。 為按鈕的線性布局分配android:layout_height="wrap_content"
。 這是我的工作布局:
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:orientation="vertical" >
<ListView
android:id="@+id/listView1"
android:layout_width="match_parent"
android:layout_height="0dp"
android:layout_weight="1" />
<LinearLayout
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:orientation="horizontal">
<Button
android:id="@+id/new_button"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_weight="1"
android:text="New" />
<Button
android:id="@+id/suggest_button"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_weight="1"
android:text="Suggest" />
</LinearLayout>
</LinearLayout>
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:orientation="vertical"
android:layout_width="fill_parent"
android:layout_height="fill_parent"
android:background="#ffffff"
>
<ListView android:id="@+id/ListView01"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_weight="1">
</ListView>
<FrameLayout android:id="@+id/FrameLayout01"
android:layout_width="fill_parent"
android:layout_height="wrap_content">
<Button android:id="@+id/Button01"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="button"
android:layout_gravity="center_horizontal">
</Button>
</FrameLayout>
</LinearLayout>
這是您正在尋找的設計。 試試吧。
RelativeLayout
將忽略其子女android:layout_width
或android:layout_height
屬性,如果孩子有一個正確定義它們的屬性left
和right
或top
和bottom
分別值。
要在右側圖像上顯示結果,顯示按鈕上方的列表,您的布局應如下所示:
<RelativeLayout
xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="match_parent">
<android.support.v7.widget.RecyclerView
android:id="@android:id/list"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:layout_above="@android:id/button1"
android:layout_alignParentTop="true"/>
<Button
android:id="@android:id/button1"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_alignParentBottom="true"
android:text="@android:string/ok"/>
</RelativeLayout>
關鍵是要定義android:layout_alignParentTop
(定義top
值)和android:layout_above
(定義bottom
值)在RecyclerView
。 這樣, RelativeLayout
將忽略android:layout_height="match_parent"
,並且RecyclerView
將被放置在Button
上方。
另外,請確保查看android:layout_alignWithParentIfMissing
,如果您有更復雜的布局,並且仍需要定義這些值。
我正在使用Xamarin Android,我的要求與上面的William T. Mallard完全相同,即下面有2個並排按鈕的ListView。 解決方案是這個答案在Xamarin Studio中不起作用 - 當我將ListView的高度設置為“0dp”時,ListView就消失了。
我的Xamarin Android代碼如下:
<?xml version="1.0" encoding="utf-8"?>
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:orientation="vertical"
android:layout_width="fill_parent"
android:layout_height="fill_parent">
<ListView
android:id="@+id/ListView1"
android:layout_width="fill_parent"
android:layout_height="wrap_content"
android:layout_weight="1"
android:layout_above="@+id/ButtonsLinearLayout" />
<LinearLayout
android:id="@id/ButtonsLinearLayout"
android:layout_height="wrap_content"
android:layout_width="fill_parent"
android:orientation="horizontal"
android:layout_alignParentBottom="true">
<Button
android:id="@+id/Button1"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_weight="1" />
<Button
android:id="@+id/Button2"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_weight="1" />
</LinearLayout>
</RelativeLayout>
我將ButtonsLinearLayout對齊到屏幕的底部,並將ListView設置為高於ButtonsLinearLayout。
在你的相對布局中,listview的高度是match_parent
,這是fill_parent(對於2.1及更早版本),所以最好的解決方案是如果你想使用相對布局然后首先聲明你的按鈕然后你的列表視圖,使列表視圖位置如上面你的按鈕ID,如果你想要按鈕總是在底部然后使它alignParentBottom ..片段是
<RelativeLayout
android:layout_width="fill_parent" android:layout_height="fill_parent"
android:id="@+id/rl1"><Button
android:layout_width="MATCH_PARENT"
android:layout_height="WRAP_CONTENT"
/><ListView
android:layout_width="MATCH_PARENT"
android:layout_height="0"
android:layout_above="@id/listview"/></RelativeLayout>
這可以防止您的列表視圖占據整個位置,並使您的按鈕出現..
@jclova你可以做的另一件事是在相對布局中使用layout-below=@+id/listviewid
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.