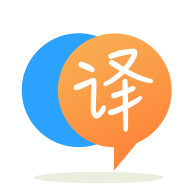
[英]Getting xml element name from unmarshalled java object with JAXB
[英]posting xml to a spring REST endpoint is not getting unmarshalled into the relevant Java object
我有一個項目,其中給出了xsd。 我使用xjc編譯器生成Java類。 然后我用XmlRootElement屬性注釋該類。 我已經在AnnotationMethodHandlerAdapter中使用Jaxb2編組/解組bean配置了sevlet。 當我發送一個沒有命名空間的xml時,我得到415錯誤。
源代碼如下文件 - web.xml
<?xml version="1.0" encoding="UTF-8"?>
<web-app xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xmlns="http://java.sun.com/xml/ns/javaee" xmlns:web="http://java.sun.com/xml/ns/javaee/web-app_2_5.xsd" xsi:schemaLocation="http://java.sun.com/xml/ns/javaee http://java.sun.com/xml/ns/javaee/web-app_3_0.xsd" id="WebApp_ID" version="3.0">
<display-name>Test</display-name>
<servlet>
<servlet-name>dispatcher</servlet-name>
<servlet-class> org.springframework.web.servlet.DispatcherServlet </servlet-class>
<load-on-startup>1</load-on-startup>
</servlet>
<servlet-mapping>
<servlet-name>dispatcher</servlet-name>
<url-pattern>/rest/*</url-pattern>
</servlet-mapping>
</web-app>
file - dispatcher-servlet.xml
<beans xmlns="http://www.springframework.org/schema/beans"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xmlns:p="http://www.springframework.org/schema/p"
xmlns:context="http://www.springframework.org/schema/context" xmlns:tx="http://www.springframework.org/schema/tx"
xmlns:mvc="http://www.springframework.org/schema/mvc"
xsi:schemaLocation="
http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans-3.0.xsd
http://www.springframework.org/schema/context http://www.springframework.org/schema/context/spring-context-3.0.xsd
http://www.springframework.org/schema/tx http://www.springframework.org/schema/tx/spring-tx-3.0.xsd
http://www.springframework.org/schema/mvc http://www.springframework.org/schema/mvc/spring-mvc-3.0.xsd
http://www.springframework.org/schema/oxm http://www.springframework.org/schema/oxm/spring-oxm-3.0.xsd"
xmlns:oxm="http://www.springframework.org/schema/oxm">
<context:component-scan base-package="com.test.endpoints" />
<tx:annotation-driven />
<bean class="org.springframework.web.servlet.mvc.annotation.AnnotationMethodHandlerAdapter">
<property name="messageConverters">
<list>
<ref bean="marshallingHttpMessageConverter"/>
</list>
</property>
</bean>
<bean id="marshallingHttpMessageConverter"
class="org.springframework.http.converter.xml.MarshallingHttpMessageConverter">
<constructor-arg ref="jaxb2Marshaller" />
</bean>
<bean id="jaxb2Marshaller" class="org.springframework.oxm.jaxb.Jaxb2Marshaller">
<property name="classesToBeBound">
<list>
<value>com.test.users.User</value>
<value>com.test.users.Users</value>
</list>
</property>
</bean>
<!-- Should be defined last! -->
<!-- <mvc:annotation-driven />-->
</beans>
file - user.xsd
<element name="users">
<complexType>
<sequence>
<element name="user" type="tns:user" minOccurs="0"
maxOccurs="unbounded" />
</sequence>
</complexType>
</element>
<complexType name="user">
<sequence>
<element name="id" type="int" />
<element name="email" type="string"></element>
<element name="first_name" type="string"></element>
<element name="last_name" type="string"></element>
</sequence>
</complexType>
</schema>
使用此命令為上述xsd生成Java類。
xjc -p com.test.users ..\xsd\user.xsd
此命令的輸出是
parsing a schema...
compiling a schema...
com\test\users\ObjectFactory.java
com\test\users\User.java
com\test\users\Users.java
com\test\users\package-info.java
帶有@XmlRootElement(name =“user”)的帶注釋的User.java。
file - UserService.java
package com.test.endpoints;
import org.springframework.stereotype.Controller;
import org.springframework.web.bind.annotation.RequestBody;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RequestMethod;
import org.springframework.web.bind.annotation.ResponseBody;
import com.test.users.User;
@Controller
@RequestMapping("/users")
public class UserService {
@RequestMapping(value="/new", method=RequestMethod.POST)
@ResponseBody
public User createUser(@RequestBody User user) {
System.out.println(user.getFirstName());
return user;
}
}
使用此curl命令測試REST api
curl -X POST -HContent-type:application/xml -HAccept:application/xml --data "<?xml version="1.0" encoding="UTF-8"?><user><id>1</id><email>email@email.com</email><first_name>first_name</first_name><last_name>last_name</last_name></user>" http://localhost:8080/Test/rest/users/new
輸出是
The request sent by the client was syntactically incorrect ()
有人可以指出我哪里出錯了。
謝謝
我也遇到了同樣的問題。 這是我的Controller方法的樣子:
@RequestMapping(method=RequestMethod.POST, value="/users/create",
headers="Accept=application/xml")
public @ResponseBody User createUser(@RequestBody User user) {
return user;
}
基本上,我只是將用戶對象作為概念驗證返回,以確保我的POST正常工作。 但是,我一直在遇到“語法不正確”的消息。 實際上,我實際更新了代碼以檢索有效的User對象,如下所示:
@RequestMapping(method=RequestMethod.POST, value="/users/create",
headers="Accept=application/xml")
public @ResponseBody User createUser(@RequestBody User user) {
userService.createUser(user);
// do work
User newUser = userService.getUserById(user.getId());
return newUser;
}
它開始正常工作。
我使用以下CURL命令測試了我的REST API:
curl -X POST -HContent-type:application/xml -HAccept:application/xml --data "<user><username>Quinnster</username><password>password</password></user>" http://localhost:8080/api/users/create
使用不同的API列出用戶,我能夠驗證我的新用戶是否已正確創建。
再一次,不確定這是否是你遇到的完全相同的問題,但這解決了我。
謝謝,奎因
您可以嘗試更改類的@RequestMapping:
@RequestMapping("/users")
至:
@RequestMapping("/users/*")
然后將方法聲明更改為:
@RequestMaqpping(method=RequestMethod.POST)
@ResponseBody
public void create(@RequestBody User user) {
然后該URL將變為: http:// localhost:8080 / rest / users / create ,因為spring將從方法名稱獲取url的最后部分。
嘗試將卷曲線更改為
curl -X POST -HContent-type:application/xml -HAccept:application/xml \
--data '<?xml version="1.0" encoding="UTF-8"?><user><id>1</id><email>email@email.com</email><first_name>first_name</first_name><last_name>last_name</last_name></user>' \
http://localhost:8080/Test/rest/users/new
它們是XML中的引號,可能干擾了輸入
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.