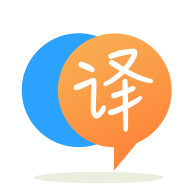
[英]If a word is repeated many times in a string, how can I count the number of repetitions of the word and their positions?
[英]Max Repeated Word in a string
我試圖做一個非常常見的面試問題“在一個字符串中找到最大重復的單詞”,並且在c / c ++實現中找不到很多網絡資源。 所以我自己在這里編碼。 我試圖從頭開始編寫大部分代碼以便更好地理解。 你能查看我的代碼並提供我的算法評論。 有些人建議使用哈希表來存儲計數,但這里沒有使用哈希表。
#include<stdafx.h>
#include<stdlib.h>
#include<stdio.h>
#include<string>
#include<iostream>
using namespace std;
string word[10];
//splitting string into words
int parsestr(string str)
{
int index = 0;
int i = 0;
int maxlength = str.length();
int wordcnt = 0;
while(i < maxlength)
{
if(str[i]!= ' ')
{
word[index] = word[index]+str[i];
}
else
{
index++;//new word
wordcnt = index;
}
i++;
}
return wordcnt;
}
//find the max word count out of the array and return the word corresponding to that index.
string maxrepeatedWord(int wordcntArr[],int count)
{
int max = 0;
int index = 0;
for(int i=0;i<=count;i++)
{
if(wordcntArr[i] > max)
{
max = wordcntArr[i];
index = i;
}
}
return word[index];
}
void countwords(int count)
{
int wordcnt = 0;
int wordcntArr[10];
string maxrepeatedword;
for(int i=0;i<=count ;i++)
{
for(int j=0;j<=count;j++)
{
if(word[i]==word[j])
{
wordcnt++;
//word[j] = "";
}
else
{}
}
cout<<" word "<< word[i] <<" occurs "<< wordcnt <<" times "<<endl;
wordcntArr[i] = wordcnt;
wordcnt = 0;
}
maxrepeatedword = maxrepeatedWord(wordcntArr,count);
cout<< " Max Repeated Word is " << maxrepeatedword;
}
int main()
{
string str = "I am am am good good";
int wordcount = 0;
wordcount = parsestr(str);
countwords(wordcount);
}
僅僅為了比較,最明顯的方法是C ++是:
#include <map>
#include <string>
#include <iostream>
#include <sstream>
int main()
{
std::istringstream input("I am am am good good");
std::map<std::string, int> count;
std::string word;
decltype(count)::const_iterator most_common;
while (input >> word)
{
auto iterator = count.emplace(word, 0).first;
++iterator->second;
if (count.size() == 1 ||
iterator->second > most_common->second)
most_common = iterator;
}
std::cout << '\"' << most_common->first << "' repeated "
<< most_common->second << " times\n";
}
看到它在這里運行。
筆記:
map::emplace
返回一pair<iterator,bool>
指示單詞及其計數在map
,以及是否新插入。 我們只關心捕捉安置的地方 emplace(...).first
。
當我們更新計數時,我們檢查是否使該單詞成為迄今為止看到的最常見的單詞。 如果是這樣,我們將迭代器復制到局部變量most_common
,因此我們記錄了目前最常見的單詞及其計數。
你正在做的一些事情值得考慮:
word
是一個全局變量 - 將事物作為函數參數傳遞是一個好習慣,除非它非常不方便,這意味着代碼可以更容易地從異步信號處理程序或其他線程中重用,並且在查看函數調用站點時更明顯的是輸入和輸出可能是。 按countwords(wordcount)
,調用countwords(wordcount)
使其看起來像countwords'唯一的輸入是int wordcount
。 std::string::operator+=(char)
來更簡潔地附加一個char
ala my_string += my_char;
一般來說,你的代碼非常合理,並且對迭代和問題解決有很好的理解,所有這些都是非常低級的,但這是一個很好的東西,需要以非常實際的方式理解。
void mostRepeat(string words[], int n)
{
int hash[n]={0};
for(int j=0; j<n; j++)
{
for(int i=0; i<n; i++)
{
if(words[j]==words[i]) hash[j]++;
}
}
int maxi = hash[0];
int index = 0;
for(int i=0; i<n; i++)
{
if(maxi<hash[i])
{
maxi=hash[i];
index = i;
}
}
cout<<words[index]<<endl;
}
完整計划: 鏈接
import java.util.*;
public class StringWordDuplicates {
static void duplicate(String inputString){
HashMap<String, Integer> wordCount = new HashMap<String,Integer>();
String[] words = inputString.split(" ");
for(String word : words){
if(wordCount.containsKey(word)){
wordCount.put(word, wordCount.get(word)+1);
}
else{
wordCount.put(word, 1);
}
}
//Extracting of all keys of word count
Set<String> wordsInString = wordCount.keySet();
for(String word : wordsInString){
if(wordCount.get(word)>1){
System.out.println(word+":"+wordCount.get(word));
}
}
}
public static void main(String args[]){
duplicate("I am Java Programmer and IT Server Programmer with Java as Best Java lover");
}
}
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.