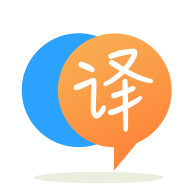
[英]unrecognized selector sent to instance while trying to add an object to a mutable array
[英]How can i add new object instance in a mutable array using same object reference as the previous one
//viewController.h file
//---------------------
#import <UIKit/UIKit.h>
@interface ItemClass : NSObject
{
NSString* name;
}
@property (nonatomic, retain) NSString* name;
@end
@interface PlaceClass : ItemClass
{
NSString* coordinates;
}
@property (nonatomic, retain) NSString* coordinates;
@end
@interface viewController : UIViewController {
NSMutableArray* placesMutArray;
PlaceClass* currentPlace;
}
@end
//viewController.m file
//------------------------
#import "viewController.h"
@implementation ItemClass
@synthesize name;
@end
@implementation PlaceClass
@synthesize coordinates;
@end
@implementation viewController
- (void)viewDidLoad {
[super viewDidLoad];
placesMutArray = [[NSMutableArray alloc] init];
currentPlace = [[PlaceClass alloc] init];
// at some point in code the properties of currentPlace are set
currentPlace.name = [NSString stringWithFormat:@"abc"];
currentPlace.coordinates = [NSString stringWithFormat:@"45.25,24.22"];
// currentPlace added to mutable array
[placesMutArray addObject:currentPlace];
//now the properties of currentPlace are changed
currentPlace.name = [NSString stringWithFormat:@"def"];
currentPlace.coordinates = [NSString stringWithFormat:@"45.48,75.25"];
// again currentPlace added to mutable array
[placesMutArray addObject:currentPlace];
for(PlaceClass* x in placesMutArray)
{
NSLog(@"Name is : %@", x.name);
}
}
@end
我得到的輸出:
Name is : def
Name is : def
期望的輸出:
Name is : abc
Name is : def
我希望placesMutArray具有兩個單獨的對象(每個對象分配了單獨的內存空間),每個對象都有自己的“名稱”和“坐標”屬性集。 但是,上面的代碼顯然只是更改了同一對象“ currentPlaces”的屬性,並且其引用兩次添加到了數組中。 暗示我只在內存中分配了一個對象。 當我使用快速枚舉和NSlog遍歷數組時,兩個元素的name屬性都會兩次出現最后一次設置的值。
采用NSCopying協議可以解決問題嗎?
[placesMutArray addObject:[currentPlace copy]];
如果是,那我應該怎么做? 我嘗試過,但是我遇到很多錯誤。
您僅創建了一個PlaceClass對象(currentPlace),然后將對此PlaceClass對象的2個引用添加到了數組中。 您必須創建第二個對象
secondPlace = [[PlaceClass alloc] init];
secondPlace.name = [NSString stringWithFormat:@"def"];
secondPlace.coordinates = [NSString stringWithFormat:@"45.48,75.25"];
[placesMutArray addObject:secondPlace];
要么
secondPlace = [currentPlace mutableCopy];
secondPlace.name = [NSString stringWithFormat:@"def"];
secondPlace.coordinates = [NSString stringWithFormat:@"45.48,75.25"];
[placesMutArray addObject:secondPlace];
無論哪種方式,請記住在使用alloc,復制或保留時釋放對象
[secondPlace release];
您使用的是同一個實例,這是正確的。 您只需要制作一個新的。
嘗試這個 :
// Create a second PlaceClass before setting it's properties to 'def'
currentPlace = [[PlaceClass alloc] init];
currentPlace.name = [NSString stringWithFormat:@"def"];
currentPlace.coordinates = [NSString stringWithFormat:@"45.48,75.25"];
將對象添加到數組不會為您復制該對象-只是意味着該數組知道該對象。 在將第一個currentPlace添加到數組后, currentPlace
變量仍指向第一個對象,因此當開始設置新名稱和坐標時,您將更新第一個對象,而不創建新對象。
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.