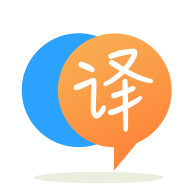
[英]PHP MySQL - How easy way to update the database when one or more fields value changed
[英]how to update one or more fields ignoring the empty fields into mysql database?
我正在尋求有關忽略 null 值以更新 mysql 數據庫的幫助:-
$cst = $_POST['custname'];
$a = $_POST['tel'];
$b = $_POST['fax'];
$c = $_POST['email'];
$sql = mysql_query("UPDATE contacts SET TEL = '$a', FAX = '$b', EMAIL = '$c'
WHERE Cust_Name = '$cst' ");
我如何合並一個選項,用戶只能 select 一個或所有字段進行更新。
我嘗試根據收到的響應使用以下代碼,但它做同樣的事情。 用空白數據覆蓋現有數據。
$upd = mysql_query("UPDATE custcomm_T SET
Telephone = ".(is_null($a)?'Telephone':"'$a'").",
Fax = ".(is_null($b)?'Fax':"'$b'").",
Mobile = ".(is_null($c)?'Mobile':"'$c'").",
EMail = ".(is_null($d)?'EMail':"'$d'").",
trlicense = ".(is_null($e)?'trlicense':"'$e'").",
trlicexp = ".(is_null($f)?'trlicexp':"'$f'")."
WHERE Cust_Name_VC = '$g' ") or die(mysql_error());
首先要記住通過 POST、GET 或 REQUEST 轉義任何給您的字符串(如果您不確定原因,請閱讀 SQL 注入攻擊)。
像這樣的東西可能會起作用:
$semaphore = false;
$query = "UPDATE contacts SET ";
$fields = array('tel','fax','email');
foreach ($fields as $field) {
if (isset($_POST[$field]) and !empty($_POST[$field]) {
$var = mysql_real_escape_string($_POST[$field]);
$query .= uppercase($field) . " = '$var'";
$semaphore = true;
}
}
if ($semaphore) {
$query .= " WHERE Cust_Name = '$cst'";
mysql_query($query);
}
注意:永遠不要簡單地遍歷 $_POST 數組來創建 SQL 語句。 對手可以添加額外的 POST 字段並可能造成惡作劇。 遍歷用戶輸入數組也可能導致注入向量:字段名稱需要添加到語句中,這意味着它們是潛在向量。 標准注入預防技術(准備好的語句參數、驅動程序提供的引用函數)不適用於標識符。 相反,使用字段的白名單進行設置,並在白名單上循環或通過白名單傳遞輸入數組。
您需要構建您的查詢。 像這樣的東西:
$query = 'update contacts set ';
if ($_POST['tel'] != '') $query .= 'TEL="'.$_POST['tel'].'", ';
if ($_POST['fax'] != '') $query .= 'FAX="'.$_POST['fax'].'", ';
if ($_POST['email'] != '') $query .= 'EMAIL="'.$_POST['email'].'", ';
$query .= "Cust_Name = '$cst' where Cust_Name = '$cst'";
最后一個更新字段:Cust_Name = '$cst' 基本上是“刪除”最后一個逗號。
請記住,在使用之前應該清理 $_POST 值,並且所有 $_POST 值都是字符串,所以一個空字段是 '' 而不是 null,這樣的事情會起作用:
foreach ($_POST as $var=>$value) {
if(empty($value)) continue; //skip blank fields (may be problematic if you're trying to update a field to be empty)
$sets[]="$var= '$value";
}
$set=implode(', ',$sets);
$q_save="UPDATE mytable SET $set WHERE blah=$foo";
這應該有效(MySQL 方式):
"UPDATE `custcomm_T`
SET `Telephone` = IF(TRIM('" . mysql_real_escape_string($a) . "') != '', '" . mysql_real_escape_string($a) . "', `Telephone`),
SET `Fax` = IF(TRIM('" . mysql_real_escape_string($b) . "') != '', '" . mysql_real_escape_string($b) . "', `Fax`),
SET `Mobile` = IF(TRIM('" . mysql_real_escape_string($c) . "') != '', '" . mysql_real_escape_string($c) . "', `Mobile`),
SET `EMail` = IF(TRIM('" . mysql_real_escape_string($d) . "') != '', '" . mysql_real_escape_string($d) . "', `EMail`),
SET `trlicense` = IF(TRIM('" . mysql_real_escape_string($e) . "') != '', '" . mysql_real_escape_string($e) . "', `trilicense`),
SET `trlicexp` = IF(TRIM('" . mysql_real_escape_string($f) . "') != '', '" . mysql_real_escape_string($f) . "', `trlicexp`)
WHERE Cust_Name_VC = '" . mysql_real_escape_string($g) . '";
我已嘗試將列和變量保留為您在問題中發布的內容,但請隨時根據您的架構進行更正。
希望能幫助到你。
循環可選輸入字段,建立要設置的字段。 字段名稱和值應分開保存,以便您可以使用准備好的語句。 作為基本驗證步驟,您還可以遍歷必填字段。
# arrays of input => db field names. If both are the same, no index is required.
$optional = array('tel' => 'telephone', 'fax', 'email');
$required = array('custname' => 'cust_name');
# $input is used rather than $_POST directly, so the code can easily be adapted to
# work with any array.
$input =& $_POST;
/* Basic validation: check that required fields are non-empty. More than is
necessary for the example problem, but this will work more generally for an
arbitrary number of required fields. In production code, validation should be
handled by a separate method/class/module.
*/
foreach ($required as $key => $field) {
# allows for input name to be different from column name, or not
if (is_int($key)) {
$key = $field;
}
if (empty($input[$key])) {
# error: input field is required
$errors[$key] = "empty";
}
}
if ($errors) {
# present errors to user.
...
} else {
# Build the statement and argument array.
$toSet = array();
$args = array();
foreach ($optional as $key => $field) {
# allows for input name to be different from column name, or not
if (is_int($key)) {
$key = $field;
}
if (! empty($input[$key])) {
$toSet[] = "$key = ?";
$args[] = $input[$key];
}
}
if ($toSet) {
$updateContactsStmt = "UPDATE contacts SET " . join(', ', $toSet) . " WHERE cust_name = ?";
$args[] = $input['custname'];
try {
$updateContacts = $db->prepare($updateContactsStmt);
if (! $updateContacts->execute($args)) {
# update failed
...
}
} catch (PDOException $exc) {
# DB error. Don't reveal exact error message to non-admins.
...
}
} else {
# error: no fields to update. Inform user.
...
}
}
這應該在數據庫和程序對象之間設計為 map 的數據訪問層中處理。 如果你很聰明,你可以編寫一個適用於任意模型的單一方法(相關的 forms、表和類)。
mysql_query("
UPDATE contacts
SET
TEL = ".(is_null($a)?'TEL':"'$a'").",
FAX = ".(is_null($b)?'FAX':"'$b'").",
EMAIL = ".(is_null($c)?'EMAIL':"'$c'")."
WHERE Cust_Name = '$cst'
");
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.