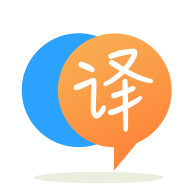
[英]How can I repeatedly change the background style of a div using a click event in Javascript?
[英]How can I create and style a div using JavaScript?
如何使用 JavaScript 創建和設置樣式(並附加到頁面)帶有內容的 div? 我知道這是可能的,但如何?
var div = document.createElement("div"); div.style.width = "100px"; div.style.height = "100px"; div.style.background = "red"; div.style.color = "white"; div.innerHTML = "Hello"; document.getElementById("main").appendChild(div);
<body> <div id="main"></div> </body>
var div = document.createElement("div");
div.style.width = "100px";
div.style.height = "100px";
div.style.background = "red";
div.style.color = "white";
div.innerHTML = "Hello";
document.getElementById("main").appendChild(div);
OR
document.body.appendChild(div);
使用父引用而不是document.body
。
取決於你是怎么做的。 純JavaScript:
var div = document.createElement('div');
div.innerHTML = "my <b>new</b> skill - <large>DOM maniuplation!</large>";
// set style
div.style.color = 'red';
// better to use CSS though - just set class
div.setAttribute('class', 'myclass'); // and make sure myclass has some styles in css
document.body.appendChild(div);
使用 jquery 做同樣的事情非常容易:
$('body')
.append('my DOM manupulation skills dont seem like a big deal when using jquery')
.css('color', 'red').addClass('myclass');
干杯!
雖然這里的其他答案有效,但我注意到您要求一個包含內容的 div。 所以這是我的帶有額外內容的版本。 底部的 JSFiddle 鏈接。
JavaScript (帶注釋) :
// Creating a div element
var divElement = document.createElement("Div");
divElement.id = "divID";
// Styling it
divElement.style.textAlign = "center";
divElement.style.fontWeight = "bold";
divElement.style.fontSize = "smaller";
divElement.style.paddingTop = "15px";
// Adding a paragraph to it
var paragraph = document.createElement("P");
var text = document.createTextNode("Another paragraph, yay! This one will be styled different from the rest since we styled the DIV we specifically created.");
paragraph.appendChild(text);
divElement.appendChild(paragraph);
// Adding a button, cause why not!
var button = document.createElement("Button");
var textForButton = document.createTextNode("Release the alert");
button.appendChild(textForButton);
button.addEventListener("click", function(){
alert("Hi!");
});
divElement.appendChild(button);
// Appending the div element to body
document.getElementsByTagName("body")[0].appendChild(divElement);
HTML:
<body>
<h1>Title</h1>
<p>This is a paragraph. Well, kind of.</p>
</body>
CSS:
h1 { color: #333333; font-family: 'Bitter', serif; font-size: 50px; font-weight: normal; line-height: 54px; margin: 0 0 54px; }
p { color: #333333; font-family: Georgia, serif; font-size: 18px; line-height: 28px; margin: 0 0 28px; }
注意:從 Ratal Tomal 借來的 CSS 行
JSFiddle: https ://jsfiddle.net/Rani_Kheir/erL7aowz/
此解決方案使用 jquery 庫
$('#elementId').append("<div class='classname'>content</div>");
我喜歡做的另一件事是創建一個對象,然后循環遍歷該對象並設置這樣的樣式,因為逐個編寫每個樣式可能很乏味。
var bookStyles = {
color: "red",
backgroundColor: "blue",
height: "300px",
width: "200px"
};
let div = document.createElement("div");
for (let style in bookStyles) {
div.style[style] = bookStyles[style];
}
body.appendChild(div);
這是我會使用的一種解決方案:
var div = '<div id="yourId" class="yourClass" yourAttribute="yourAttributeValue">blah</div>';
如果您希望屬性和/或屬性值基於變量:
var id = "hello";
var classAttr = "class";
var div = '<div id='+id+' '+classAttr+'="world" >Blah</div>';
然后,附加到正文:
document.getElementsByTagName("body").innerHTML = div;
非常簡單。
這將在具有自定義 CSS 的函數或腳本標記內,類名為自定義
var board = document.createElement('div');
board.className = "Custom";
board.innerHTML = "your data";
console.log(count);
document.getElementById('notification').appendChild(board);
使用 id 名稱創建 div
var divCreator=function (id){
newElement=document.createElement("div");
newNode=document.body.appendChild(newElement);
newNode.setAttribute("id",id);
}
將文本添加到 div
var textAdder = function(id, text) {
target = document.getElementById(id)
target.appendChild(document.createTextNode(text));
}
測試代碼
divCreator("div1");
textAdder("div1", "this is paragraph 1");
輸出
this is paragraph 1
你可以這樣創建
board.style.cssText = "position:fixed;height:100px;width:100px;background:#ddd;"
document.getElementById("main").appendChild(board);
完整的可運行片段:
var board; board= document.createElement("div"); board.id = "mainBoard"; board.style.cssText = "position:fixed;height:100px;width:100px;background:#ddd;" document.getElementById("main").appendChild(board);
<body> <div id="main"></div> </body>
這是一個使用一些漂亮的可重用 DOM 實用程序函數的小示例:
// DOM utility functions: const ELNew = (tag, prop) => Object.assign(document.createElement(tag), prop), ELS = (sel, par) => (par ?? document).querySelectorAll(sel), EL = (sel, par) => (par ?? document).querySelector(sel); // Task: const EL_new = ELNew("div", { className: "item", textContent: "Hello, World!", onclick() { console.log(this.textContent); }, style: ` font-size: 2em; color: brown; background: gold; ` }); // Append it EL("body").append(EL_new);
此外,您還可以使用Object.assign() 設置元素的樣式,例如:
// Add additional styles later:
Object.assign(EL_new.style, {
color: "red",
background: "yellow",
fontSize: "3rem",
});
您可以使用以下方法:
document.write()
這很簡單,在下面的文檔中我會解釋
document.write("<div class='div'>Some content inside the div (It is styled!)</div>")
.div { background-color: red; padding: 5px; color: #fff; font-family: Arial; cursor: pointer; } .div:hover { background-color: blue; padding: 10px; } .div:hover:before { content: 'Hover! '; } .div:active { background-color: green; padding: 15px; } .div:active:after { content: ' Active! or clicked...'; }
<p>Below or above well show the div</p> <p>Try pointing hover it and clicking on it. Those are tha styles aplayed. The text and background color changes.</p>
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.