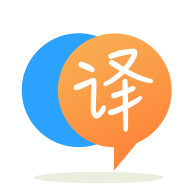
[英]Is there any way to put a variable inside telnet command in python?
[英]Is there any way to do HTTP PUT in python
我需要在 python 中使用 HTTP PUT
將一些數據上傳到服務器。 從我對 urllib2 文檔的簡要閱讀來看,它只執行 HTTP POST
。 有什么辦法可以在 python 中執行 HTTP PUT
?
我過去使用過各種 python HTTP 庫,我最喜歡的是requests 。 現有的庫有非常有用的接口,但代碼最終可能會變得太長而無法進行簡單的操作。 請求中的基本 PUT 如下所示:
payload = {'username': 'bob', 'email': 'bob@bob.com'}
>>> r = requests.put("http://somedomain.org/endpoint", data=payload)
然后您可以檢查響應狀態代碼:
r.status_code
或響應:
r.content
Requests 有很多語法糖和快捷方式,可以讓您的生活更輕松。
import urllib2
opener = urllib2.build_opener(urllib2.HTTPHandler)
request = urllib2.Request('http://example.org', data='your_put_data')
request.add_header('Content-Type', 'your/contenttype')
request.get_method = lambda: 'PUT'
url = opener.open(request)
Httplib 似乎是一個更簡潔的選擇。
import httplib
connection = httplib.HTTPConnection('1.2.3.4:1234')
body_content = 'BODY CONTENT GOES HERE'
connection.request('PUT', '/url/path/to/put/to', body_content)
result = connection.getresponse()
# Now result.status and result.reason contains interesting stuff
您可以使用請求庫,與采用 urllib2 方法相比,它簡化了很多事情。 首先從 pip 安裝它:
pip install requests
有關安裝請求的更多信息。
然后設置放置請求:
import requests
import json
url = 'https://api.github.com/some/endpoint'
payload = {'some': 'data'}
# Create your header as required
headers = {"content-type": "application/json", "Authorization": "<auth-key>" }
r = requests.put(url, data=json.dumps(payload), headers=headers)
請參閱請求庫的快速入門。 我認為這比 urllib2 簡單得多,但確實需要安裝和導入這個額外的包。
這在 python3 中做得更好,並記錄在stdlib 文檔中
urllib.request.Request
類在 python3 中獲得了一個method=...
參數。
一些示例用法:
req = urllib.request.Request('https://example.com/', data=b'DATA!', method='PUT')
urllib.request.urlopen(req)
您應該看看httplib 模塊。 它應該允許您發出任何類型的 HTTP 請求。
不久前我也需要解決這個問題,這樣我才能充當 RESTful API 的客戶端。 我選擇了 httplib2,因為除了 GET 和 POST 之外,它還允許我發送 PUT 和 DELETE。 Httplib2 不是標准庫的一部分,但您可以從奶酪店輕松獲得它。
我還推薦 Joe Gregario 的httplib2 。 我經常使用它而不是標准庫中的 httplib。
你看過put.py了嗎? 我過去用過它。 您也可以使用 urllib 破解您自己的請求。
您當然可以在從套接字到調整 urllib 的任何級別上使用現有的標准庫來滾動自己的庫。
http://pycurl.sourceforge.net/
“PyCurl 是 libcurl 的 Python 接口。”
“libcurl 是一個免費且易於使用的客戶端 URL 傳輸庫,...支持...HTTP PUT”
“PycURL 的主要缺點是它是 libcurl 之上的一個相對較薄的層,沒有任何那些漂亮的 Pythonic 類層次結構。這意味着它的學習曲線有點陡峭,除非您已經熟悉 libcurl 的 C API。”
如果你想留在標准庫中,你可以urllib2.Request
:
import urllib2
class RequestWithMethod(urllib2.Request):
def __init__(self, *args, **kwargs):
self._method = kwargs.pop('method', None)
urllib2.Request.__init__(self, *args, **kwargs)
def get_method(self):
return self._method if self._method else super(RequestWithMethod, self).get_method()
def put_request(url, data):
opener = urllib2.build_opener(urllib2.HTTPHandler)
request = RequestWithMethod(url, method='PUT', data=data)
return opener.open(request)
你可以使用requests.request
import requests
url = "https://www.example/com/some/url/"
payload="{\"param1\": 1, \"param1\": 2}"
headers = {
'Authorization': '....',
'Content-Type': 'application/json'
}
response = requests.request("PUT", url, headers=headers, data=payload)
print(response.text)
對requests
執行此操作的更正確方法是:
import requests
payload = {'username': 'bob', 'email': 'bob@bob.com'}
try:
response = requests.put(url="http://somedomain.org/endpoint", data=payload)
response.raise_for_status()
except requests.exceptions.RequestException as e:
print(e)
raise
如果 HTTP PUT 請求中存在錯誤,則會引發異常。
urllib3
為此,您需要手動對 URL 中的查詢參數進行編碼。
>>> import urllib3
>>> http = urllib3.PoolManager()
>>> from urllib.parse import urlencode
>>> encoded_args = urlencode({"name":"Zion","salary":"1123","age":"23"})
>>> url = 'http://dummy.restapiexample.com/api/v1/update/15410' + encoded_args
>>> r = http.request('PUT', url)
>>> import json
>>> json.loads(r.data.decode('utf-8'))
{'status': 'success', 'data': [], 'message': 'Successfully! Record has been updated.'}
requests
>>> import requests
>>> r = requests.put('https://httpbin.org/put', data = {'key':'value'})
>>> r.status_code
200
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.