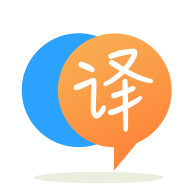
[英]OOP PHP two database accessing by two different class, how to access if two tables are in different database
[英]How to access the database class in OOP?
所以我知道不應該問標題中帶有“什么是最好的”的問題,但真的......你應該怎么做?
我們有一個數據庫 class,例如,用戶 class。 用戶 class 將獲得諸如 create() 和 update() 之類的方法,這將需要執行數據庫操作。
據我所知,有兩個主要選項,在每個 __construct() 中傳遞數據庫 object 或創建數據庫 class static。
(關於 OOP + 數據庫驅動網站的任何其他提示也值得贊賞)
這里一個非常常見的模式是使數據庫 class 成為 singleton 構造,然后將其傳遞給每個 object 構造函數(稱為依賴注入)。
使數據庫 object 成為 singleton 的目的是確保每次頁面加載只建立一個連接。 如果您出於某種原因需要多個連接,則需要以不同的方式進行操作。 不過,通過構造函數傳遞它很重要,而不是在不相關的 class 中創建數據庫 object ,這樣您就可以更輕松地測試和調試代碼。
// Basic singleton pattern for DB class
class DB
{
// Connection is a static property
private static $connection;
// Constructor is a private method so the class can't be directly instantiated with `new`
private function __construct() {}
// A private connect() method connects to your database and returns the connection object/resource
private static function connect() {
// use PDO, or MySQLi
$conn = new mysqli(...);
// Error checking, etc
return $conn;
}
// Static method retrieves existing connection or creates a new one if it doesn't exist
// via the connect() method
public static function get_connection() {
if (!self::$connection) {
self::$connection = self::connect();
// This could even call new mysqli() or new PDO() directly and skip the connect() method
// self::$connection = new mysqli(...);
}
return self::$connection;
}
}
class Other_Class
{
// accepts a DB in constructor
public function __construct($database) {
//stuff
}
}
// Database is created by calling the static method get_connetion()
$db = DB::get_connection();
$otherclass = new Other_Class($db);
// Later, to retrieve the connection again, if you don't use the variable $db
// calling DB::get_connection() returns the same connection as before since it already exists
$otherclass2 = new Other_Class(DB::get_connection());
另一種方法是創建數據庫 class直接擴展mysqli
或PDO
。 在這種情況下, __construct()
方法將 object 提供給getConnect()
,如
public static function get_connection() {
if (!self::$connection) {
self::$connection = new self(/* params to constructor */);
}
return self::$connection;
}
好吧,您可以做的是在一個 object 中擁有數據庫訪問層,然后將其傳遞給您的對象,尊重控制模式的反轉。
如果您想深入了解這個方向,請查看依賴注入 (DI): http://en.wikipedia.org/wiki/Dependency_injection
擁有 singleton 通常是個壞主意,因為在測試代碼時最終會遇到問題。
在 model class 中包含數據庫訪問邏輯,例如 User 違反了關注點分離原則。 通常 DAO(數據訪問對象)處理與數據庫相關的問題。
有 ORM 框架,例如 Hibernate,可以很好地處理 OO 和關系模型之間的不匹配,可能會節省大量的手動工作。
我真的很驚訝沒有人這么說,但這里是: ORM 。
如果您選擇的武器是 PHP,那么主要選項是Propel和Doctrine 。 他們都有很多優點和一些缺點,但毫無疑問,他們是強大的。 只是一個例子,來自 Propel(我個人最喜歡的)用戶手冊:
// retrieve a record from a database
$book = BookQuery::create()->findPK(123);
// modify. Don't worry about escaping
$book->setName('Don\'t be Hax0red!');
// persist the modification to the database
$book->save();
$books = BookQuery::create() // retrieve all books...
->filterByPublishYear(2009) // ... published in 2009
->orderByTitle() // ... ordered by title
->joinWith('Book.Author') // ... with their author
->find();
foreach($books as $book) {
echo $book->getAuthor()->getFullName();
}
你不會得到比這更多的OO!
他們會為你處理很多事情,從數據庫供應商那里抽象出你的數據。 也就是說,您應該能夠(相對輕松地)從 MySQL 移動到 SQL 服務器,如果您正在為 web 應用程序構建自己的工具,那么能夠適應不同的環境非常重要。
希望我能幫上忙!
嘿,看看ORM的。 讓他們為你做艱苦的工作? fluent nhibernate 或微軟實體框架。
我可能誤解了你的問題。 對不起,如果是這樣
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.