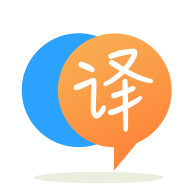
[英]Why do I get an EXC_BAD_ACCESS error when I try to create a two suffix arrays?
[英]Why am I getting a EXC BAD ACCESS error when I try to impliment this dynamic array?
如果我提供的內容還不夠,請告訴我是否需要提供我的程序的更多/不同部分。
我還在使用Airline課程開展我的課程。 Airline類包含一系列動態Flight對象。
在我擁有的航空公司課程中(無法編輯或更改,這些是為我分配的頭文件):
//Airline.h
class Airline {
public:
Airline(); // default constructor
Airline(int capacity); // construct with capacity n
void cancel(int flightNum);
void add(Flight* flight);
Flight* find(int flightNum);
Flight* find(string dest);
int getSize();
private:
Flight **array; // dynamic array of pointers to flights
int capacity; // maximum number of flights
int size; // actual number of flights
void resize();
};
//Flight.h
class Flight {
public:
Flight();
// Default constructor
Flight(int fnum, string destination);
void reserveWindow();
void reserveAisle();
void reserveSeat(int row, char seat);
void setFlightNum(int fnum);
void setDestination(string dest);
int getFlightNum();
string getDest();
protected:
int flightNumber;
bool available[20][4]; //only four seat types per row; only 20 rows
string destination;
};
//Airline.cpp
#include "Airline.h"
/* Flight **array; // dynamic array of pointers to flights
int capacity; // maximum number of flights
int size; // actual number of flights
*/
Airline::Airline(){
capacity = 1;
size = 0;
array = new Flight*[1];
}
Airline::Airline(int cap){
capacity = cap;
size = 0;
array = new Flight*[capacity];
//array = &arr;
}
void Airline::cancel(int flightNum){
for(int i = 0; i < size; i++){
if(array[i]->getFlightNum() == flightNum){
array[i] = NULL;
}
}
}
// Delete the flight with the given flight number.
// Print "Flight cancelled" if successful, or print
// "No such flight", if the flight cannot be found.
void Airline::add(Flight* flight){
if(size >= capacity){
resize();
}
array[size] = flight;
size++;
cout << array[size]->getFlightNum(); //This is where the EXC BAD ACCESS is. I set the index of the array to point to the address of the flight I sent in, but I can't access it after that...
}
// Add the given flight to the array. If there is no room,
// double the array capacity and copy the existing flights.
// When increasing the capacity, print "Capacity increased to N",
// where N is the new capacity.
Flight* Airline::find(int flightNum){
for(int i = 0; i < size; i++){
cout << array[i]->getFlightNum();
if(array[i]->getFlightNum() == flightNum){
return array[i];
}
}
return 0;
}
// Return pointer to flight with given number if present, otherwise
// return 0.
Flight* Airline::find(string dest){
for(int i = 0; i < size; i++){
if(array[i]->getDest() == dest){
return array[i];
}
}
return 0;
}
// Return pointer to flight with given destination if present,
// otherwise return 0.
int Airline::getSize(){
return size;
}
// Returns the number of Flights in the array.
void Airline::resize(){
Flight **temp = new Flight*[capacity * 2];
Flight **swapper;
for(int i = 0; i < capacity; i++){
temp[i] = array[i];
}
swapper = temp;
temp = array;
array = swapper;
delete [] temp;
capacity *= 2;
cout << "Capacity increased to " << capacity << endl;
}
// resizes the array if it exceeds capacity. New array is double size of old array.
//Flight.cpp
#include "Flight.h"
/* int flightNumber;
bool available[20][4]; //only four seat types per row; only 20 rows
string destination;
*/
Flight::Flight(){
flightNumber = 0;
destination = "X";
for(int i = 0; i < 20; i++){
for(int j = 0; j < 4; j++)
available[i][j] = false;
}
}
// Default constructor
Flight::Flight(int fnum, string dest){
flightNumber = fnum;
destination = dest;
for(int i = 0; i < 20; i++){
for(int j = 0; j < 4; j++)
available[i][j] = false;
}
}
// initialize flight with flight number and destination
void Flight::reserveWindow(){
int i, j;
char seat;
bool reserved = false;
for(i = 0; i < 20; i++){
for(j = 0; j < 4; j++){
if (j == 0 || j == 3){
if(!available[i][j] && !reserved){
available[i][j] = true;
reserved = true;
break;
}
}
}
if(reserved)
break;
}
if (!reserved) {
cout << "Not available" << endl;
}else
switch (j) {
case 0:
seat = 'A';
break;
case 4:
seat = 'D';
break;
default:
break;
}
cout << "Reserved " << i << seat << endl;
}
// If a window seat if available, reserve the first available
// seat, and print it. The first available seat is the
// lowest-numbered one in the lowest numbered row. Seat 0, is
// A, since 1 is B, and so on.
// If the seat reserved is 7D, for example, print "7D".
// If no such seat is available, print "Not available".
void Flight::reserveAisle(){
bool reserved = false;
int i, j;
char seat;
for(i = 0; i < 20; i++){
for(j = 0; j < 4; j++){
if (j == 1 || j == 2){
if(!available[i][j] && !reserved){
available[i][j] = true;
reserved = true;
break;
}
}
}
if(reserved)
break;
}
if (!reserved) {
cout << "Not available" << endl;
}else
switch (j) {
case 1:
seat = 'B';
break;
case 2:
seat = 'C';
break;
default:
break;
}
cout << "Reserved " << i << seat << endl;
}
// Just like reserveWindow, but for aisle seats.
void Flight::reserveSeat(int row, char seat){
bool reserved = false;
if (row > 20 || row < 1 || seat > 'D' || seat < 'A') {
cout << "No such seat." << endl;
return;
}
for(int i = 0; i < 20; i++){
for(int j = 0; j < 4; j++){
if(!available[i][j] && !reserved){
available[i][j] = true;
reserved = true;
break;
}
}
if(reserved)
break;
}
if (!reserved) {
cout << "Not available" << endl;
}else
cout << "Reserved " << row << seat << endl;
}
// Reserve the seat specified if available. If it is available,
// print it as in reserveWindow.
// If it does not exist, print "No such seat".
// If it exists, but is unavailable, print, "Not available".
void Flight::setFlightNum(int fnum){
flightNumber = fnum;
}
//assign the flight number for this flight
void Flight::setDestination(string dest){
destination = dest;
}
//assign the destination (airline code) for this flight
int Flight::getFlightNum(){
return flightNumber;
}
// Return the flight number
string Flight::getDest(){
return destination;
}
// Return the destination
//main.cpp
#include "Airline.h"
#include "Flight.h"
#include <fstream>
#include <iomanip>
using namespace std;
int main(){
ifstream in;
Airline airline(10);
Flight flight;
Flight* flightTemp;
string transaction;
string transType;
int flightNum;
string flightDest;
string seatType;
int seatRow;
char seatCol;
string dummy;
in.open("input4.txt");
if(!in){
cout << "File not found. Ending program." << endl;
return 1;
}
while(in >> transaction){
if(transaction == "ADD"){
in >> dummy >> flightNum >> dummy >> flightDest;
//flight(flightNum,flightDest);
flight.setFlightNum(flightNum);
flight.setDestination(flightDest);
airline.add(&flight);
cout << flight.getFlightNum(); //It works here...
}
else if(transaction == "CANCEL"){
in >> dummy >> flightNum;
airline.cancel(flightNum);
}else{
in >> transType;
if(transType == "Flight"){
in >> flightNum;
cout << flightNum;
flightTemp = airline.find(flightNum);
}else{
in >> flightDest;
flightTemp = airline.find(flightDest);
}
in >> seatType;
if(seatType == "Seat"){
in >> seatRow >> seatCol;
flightTemp->reserveSeat(seatRow - 1, seatCol - 1);
}else if(seatType == "Aisle"){
flightTemp->reserveAisle();
}else
flightTemp->reserveWindow();
}
}
in.close();
cin.get();
return 0;
}
編輯我添加了所有代碼只是為了讓它更容易。
我現在遇到的問題是當我運行嘗試訪問我的數組中的Flight中的flightNum(或任何getter方法)時。 我將數組的索引設置為指向我在add()方法中發送的航班的地址,但之后我無法訪問它...
使用您的構造函數:
Airline::Airline(){
capacity = 1;
size = 0;
Flight* arr = new Flight[capacity];
array = &arr;
}
這是什么?
Flight* arr = new Flight[capacity];
array = &arr;
以與resize
相同的方式分配array
:
Airline::Airline(){
capacity = 1;
size = 0;
array = new Flight*[1];
}
因為array
是Flight*
的數組 ,而不是指向 Flight
數組的指針
編輯:編輯后,這是您的代碼中的另一個問題:
void Airline::add(Flight* flight){
if(size >= capacity){
resize();
}
array[size] = flight;
size++;
cout << array[size]->getFlightNum();
}
你在做array[size]->getFlightNum();
在打印航班號后增加size
后。 交換size++
和cout << array[size]->getFlightNum();
:
void Airline::add(Flight* flight){
if(size >= capacity){
resize();
}
array[size] = flight;
cout << array[size]->getFlightNum();
size++;
}
EDIT2:你的Airline::cancel
功能也有問題:
void Airline::cancel(int flightNum){
for(int i = 0; i < size; i++){
if(array[i]->getFlightNum() == flightNum){
array[i] = NULL;
}
}
}
您不能只將數組中的位置設置為NULL以從數組中刪除Flight
。 一種常見的方法是用最后一個元素替換數組中的那個位置,然后減小大小:
void Airline::cancel(int flightNum){
for(int i = 0; i < size; i++){
if(array[i]->getFlightNum() == flightNum){
array[i] = array[--size];
}
}
}
Flight::reserveWindow
也會導致seg錯誤,並且代碼中仍然存在更多錯誤。 因為它是家庭作業所以我會讓你離開這里。
聽起來你正在寫超出陣列界限。 我有一種感覺問題在這里:
if(size == capacity)
{
resize();
}
看看你的病情。 如果尺寸大於容量會發生什么? 在這種情況下,它不會調整大小!
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.