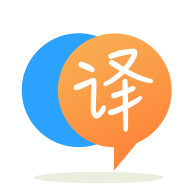
[英]c++ & OpenGl: How do you create a mesh object instance from within a class
[英]How do you create an instance of a class in C++
我是C ++初學者。 我有一堂課,當我嘗試編譯時說它缺少“主”。
我需要怎么做才能創建此類的實例並訪問其方法?
#include <string>
#include <iostream>
using namespace std;
class Account
{
string name;
double balance;
public:
Account(string n);
Account(string n, double initial_balance);
string get_name() const;
double get_balance() const;
void deposit(double amount);
void widthdraw(double amount);
};
編輯:
我將主要方法放在哪里?
我嘗試將其放在其他文件中,如下所示
#include <Account>
int main(){
Account:: Account(string n) : name(n), balance(0){}
return 0 ;
}
但這會導致錯誤,指出目錄中沒有帳戶。 我猜這是因為它沒有編譯
編輯:
兩個文件都在同一目錄中
account_main.cc
#include<string>
#include <iostream>
#include "Account.cc"
Account:: Account(string n) : name(n), balance(0){}
int main()
{
Account account("Account Name"); // A variable called "account"
account.deposit(100.00); // Calls the deposit() function on account
return 0 ;
}
Account.cc
#include<string>
#include <iostream>
using namespace std;
class Account
{
string name;
double balance;
public:
Account(string n);
Account(string n, double initial_balance);
string get_name() const;
double get_balance() const;
void deposit(double amount);
void widthdraw(double amount);
};
所有C ++程序都需要所謂的入口點 。 main()
函數始終是標准C ++程序的入口點。 您需要提供main()
函數,否則鏈接器會抱怨。 您可以通過以下兩種方式之一編寫main()
函數:
int main()
{
return 0;
}
或者,如果您期望使用命令行參數:
int main(int argc, char ** argv)
{
return 0;
}
請注意, void main()
在C ++中無效 。 還要注意,對於main()
函數來說,並非必須要使用return
語句,但是為了保持一致性,您仍然應該明確地編寫一個return
語句。
C ++標准3.6.1主要功能[basic.start.main]
5. main中的return語句的作用是保留main函數(使用自動存儲持續時間銷毀所有對象)並使用返回值作為參數調用exit。 如果控制在沒有遇到return語句的情況下到達了main的末尾,則其結果是執行
return 0;
要回答有關最新編輯的問題,請執行以下操作:
#include <Account>
int main(){
Account:: Account(string n) : name(n), balance(0){}
return 0 ;
}
main()
的形式是正確的,但這不是您提供類成員定義的方式。 構造函數必須在main函數之外。
#include <Account>
// Outside of main()
Account::Account(string n) : name(n), balance(0)
{
}
int main()
{
return 0 ;
}
要創建Account
的實例,您需要聲明一個變量並傳遞所有必需的構造函數參數,如下所示:
int main()
{
Account account("Account Name"); // A variable called "account"
account.deposit(100.00); // Calls the deposit() function on account
// Make sure you provide a function
// definition for Account::deposit().
return 0;
}
此外,檢查class Account
所在的確切文件路徑。 如果Account
類位於一個名為Account.h
的文件中,並且與包含main()
函數的文件位於同一文件夾中,則需要在該文件中使用#include "Account.h"
而不是#include <Account>
。 例如:
#include "Account.h" // Note .h and quotes
Account::Account(string n) : name(n), balance(0)
{
}
int main()
{
// ...
return 0;
}
這實際上是C ++編程語言的一個相當基本的方面。 您肯定有一本涵蓋此書的書嗎? 實際上, main()
函數和#include
語句通常是使用C ++編程時要學習的第一件事。 我強烈建議您拿起一本不錯的C ++書,並通讀它們並進行練習。
對於最新的編輯: not Account in the directory
嘗試這個:
#include "Account.h" //quotes, and .h
Account:: Account(string n) //this must be outside of main, it's its own function
: name(n), balance(0) //I put these on three seperate lines but
{} //that's a personal choice
int main(){ //this is what the code should do when it starts
Account person1("George"); //create a Account called person1 with the name George
cout << person1.get_name(); //c-output person1's name.
return 0 ; //return success (success=0)
}
正如已經指出的,您需要一本像樣的書。 要回答您的問題,您應該了解以下內容:類通常在.h文件中定義,並在.cpp或.cc文件中實現。 您應該具有三個文件:Account.h,Account.cc和main.cc。 您僅編譯.cc文件,而.h文件包含在您需要了解有關類的知識的代碼部分中(即,當您實際上在對類進行某些操作時)。 如果使用的是g ++(我認為是Linux,unix或mingw),則可以使用以下命令來編譯程序:g ++ main.cc Account.cc -o program_name
main.cc:
#include <iostream>
using namespace std;
#include "Account.h"
int main() {
Account a("first account");
cout << a.get_balance() << endl;
a.deposit(100.0);
cout << a.get_balance() << endl;
return 0;
}
您的Account.h文件應如下所示:
#include<string>
//#include <iostream> -- not needed here
// using namespace std; -- don't use 'using' in header files
class Account
{
std::string name;
double balance;
public:
Account(std::string n);
Account(std::string n, double initial_balance);
std::string get_name() const;
double get_balance() const;
void deposit(double amount);
void widthdraw(double amount);
};
最后,您的Account.cc文件應實現該類。
#include "Account.h"
using namespace std;
Account::Account(string n) : name(n), balance(0.0) {}
Account::Account(string n, double initial_balance) :
name(n), balance(initial_balance) {}
string Account::get_name() const {
return name;
}
double Account::get_balance() const {
return balance;
}
void Account::deposit(double amount) {
balance += amount;
}
void Account::widthdraw(double amount) {
balance -= amount; // generously allow overdraft...
}
希望能有所幫助。
羅曼
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.