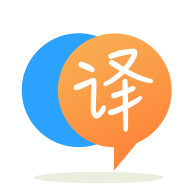
[英]Using STL algorithms (specifically std::sort) from within a templated class
[英]Sort std::vector<myclass> in one line using sort function from STL
問題是關於使用STL的算法類中的函數sort
對std::vector<myclass>
進行sort
。
標准方式是: sort(v.begin(), v.end(), &myfunct)
myfunct
在哪里:
bool myfunct( myclass first, myclass second ) {
if (first.value < second.value)
return true;
else return false;
}
上面的方法需要多於一行。 我很好奇如何做到這一點。 是否有可能在sort函數中比較myclass對象的define函數? 可能以某種方式使用(a < b) ? a : b
(a < b) ? a : b
。 我記得在C#中有這樣的事情,但是我忘記了如何調用它。 是否可以在C ++中執行。
首先,您可以只返回first.value < second.value
但這並不能擺脫該函數。 在C ++ 2011中,您可以使用lambda函數:
std::sort(begin, end, [](myclass const& f, myclass const& s){ return f.value < s.value; });
沒有C ++ 2011,我認為您將需要一個函數對象,因為沒有任何東西可以將您的類投影到您實際想要比較的值。
順便說一句,您絕對希望通過引用比較函數來傳遞除最瑣碎的對象之外的所有對象。
您可以使用boost::lambda
和boost::lambda::bind
(帶有boost lambda占位符)
std::sort(vec.begin(), vec.end(),
boost::lambda::bind(&A::a, boost::lambda::_1)
<
boost::lambda::bind(&A::a, boost::lambda::_2));
sort
將2個值傳遞給比較函數,因此您需要比較這2個值。 代碼的綁定部分僅從要比較的每個結構(由_1
和_2
引用)中從struct A
選擇變量a
。
示例代碼:
#include <iostream>
#include <algorithm>
#include <boost/lambda/lambda.hpp>
#include <boost/lambda/bind.hpp>
#include <boost/array.hpp>
struct A
{
A() : a(0), b(0) {}
int a;
int b;
};
std::ostream & operator<<(std::ostream & os, A & a)
{ return os << a.a << ":" << a.b; }
int main()
{
boost::array<A,5> vec;
std::fill(vec.begin(),vec.end(),A());
vec[0].a = 1;
vec[1].a = 3;
vec[2].a = 4;
vec[3].a = 0;
vec[4].a = 2;
std::for_each(vec.begin(),vec.end(), std::cout << boost::lambda::_1 << ' ');
std::cout << std::endl;
std::sort(vec.begin(), vec.end(),
boost::lambda::bind(&A::a, boost::lambda::_1)
<
boost::lambda::bind(&A::a, boost::lambda::_2));
std::for_each(vec.begin(),vec.end(), std::cout << boost::lambda::_1 << ' ');
std::cout << std::endl;
}
輸出:
1:0 3:0 4:0 0:0 2:0
0:0 1:0 2:0 3:0 4:0
為什么不將向量復制到集合中:
std::copy(v.begin(),v.end(),std::inserter(s,s.end()));
現在,集合中的元素將按升序排序並立即使用集合。
一個對sort()的線性調用: sort(my_vector_of_class_object.begin(),my_vector_of_class_object.end(),compare);
下面提供了“ 類對象的排序向量 ”的工作演示代碼:
#include <iostream>
#include <vector>
#include <string>
#include <algorithm>
using namespace std;
class my_Class
{
public:
my_Class(int r,int n, int s):rollno(r),name(n),status(s) { }
int getRollno() const { return rollno;}
int getName() const { return name;}
int getStatus() const { return status;}
private:
int rollno;
int name;
int status;
};
bool compare(const my_Class& x, const my_Class& y) {
return x.getRollno() < y.getRollno();
}
int main()
{
vector<my_Class> my_vector_of_class_object;
vector<my_Class>::const_iterator iter;
my_Class s1(10,20,30);
my_Class s2(40,50,60);
my_Class s3(25,85,9);
my_Class s4(1,50,2);
my_Class s5(90,70,90);
my_Class s6(85,85,3);
my_Class s7(20,6,89);
my_Class s8(70,54,22);
my_Class s9(65,22,77);
my_vector_of_class_object.push_back(s1);
my_vector_of_class_object.push_back(s2);
my_vector_of_class_object.push_back(s3);
my_vector_of_class_object.push_back(s4);
my_vector_of_class_object.push_back(s5);
my_vector_of_class_object.push_back(s6);
my_vector_of_class_object.push_back(s7);
my_vector_of_class_object.push_back(s8);
my_vector_of_class_object.push_back(s9);
cout <<"Before vector sort \n";
for(iter=my_vector_of_class_object.begin(); iter!=my_vector_of_class_object.end();++iter)
std::cout << (*iter).getRollno() << '\t' << (*iter).getName() << '\t' << (*iter).getStatus() << '\n';
cout <<" \n\n";
sort(my_vector_of_class_object.begin(),my_vector_of_class_object.end(),compare);
cout <<"After vector sort \n";
for(iter=my_vector_of_class_object.begin(); iter!=my_vector_of_class_object.end();++iter)
std::cout << (*iter).getRollno() << '\t' << (*iter).getName() << '\t' << (*iter).getStatus() << '\n';
cout <<" \n\n";
return 0;
}
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.