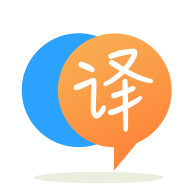
[英]How to separate one python list into 3 different lists according to the criteria
[英]How to separate a Python list into two lists, according to some aspect of the elements
我有一個這樣的列表:
[[8, "Plot", "Sunday"], [1, "unPlot", "Monday"], [12, "Plot", "Monday"], [10, "Plot", "Tuesday"], [4, "unPlot", "Tuesday"], [14, "Plot", "Wednesday"], [6, "unPlot", "Wednesday"], [1, "unPlot", "Thursday"], [19, "Plot", "Thursday"], [28, "Plot", "Friday"], [10, "unPlot", "Friday"], [3, "unPlot", "Saturday"]]
我想根據Plot
和unPlot
值將它分成兩個列表,結果如下:
list1=[[8, "Plot", "Sunday"], [12, "Plot", "Monday"], ...]
list2=[[1, "unPlot", "Monday"], [4, "unPlot", "Tuesday"], ...]
嘗試基本列表理解:
>>> [ x for x in l if x[1] == "Plot" ]
[[8, 'Plot', 'Sunday'], [12, 'Plot', 'Monday'], [10, 'Plot', 'Tuesday'], [14, 'Plot', 'Wednesday'], [19, 'Plot', 'Thursday'], [28, 'Plot', 'Friday']]
>>> [ x for x in l if x[1] == "unPlot" ]
[[1, 'unPlot', 'Monday'], [4, 'unPlot', 'Tuesday'], [6, 'unPlot', 'Wednesday'], [1, 'unPlot', 'Thursday'], [10, 'unPlot', 'Friday'], [3, 'unPlot', 'Saturday']]
如果您喜歡函數式編程,請使用filter
:
>>> filter(lambda x: x[1] == "Plot", l)
[[8, 'Plot', 'Sunday'], [12, 'Plot', 'Monday'], [10, 'Plot', 'Tuesday'], [14, 'Plot', 'Wednesday'], [19, 'Plot', 'Thursday'], [28, 'Plot', 'Friday']]
>>> filter(lambda x: x[1] == "unPlot", l)
[[1, 'unPlot', 'Monday'], [4, 'unPlot', 'Tuesday'], [6, 'unPlot', 'Wednesday'], [1, 'unPlot', 'Thursday'], [10, 'unPlot', 'Friday'], [3, 'unPlot', 'Saturday']]
我個人覺得列表理解更加清晰。 它肯定是最“pythonic”的方式。
data = [[8, "Plot", "Sunday"], [1, "unPlot", "Monday"], [12, "Plot", "Monday"], [10, "Plot", "Tuesday"], [4, "unPlot", "Tuesday"], [14, "Plot", "Wednesday"], [6, "unPlot", "Wednesday"], [1, "unPlot", "Thursday"], [19, "Plot", "Thursday"], [28, "Plot", "Friday"], [10, "unPlot", "Friday"], [3, "unPlot", "Saturday"]]
res = {'Plot':[],'unPlot':[]}
for i in data: res[i[1]].append(i)
這樣您可以迭代列表一次
嘗試:
yourList=[[8, "Plot", "Sunday"], [1, "unPlot", "Monday"], [12, "Plot", "Monday"], [10, "Plot", "Tuesday"], [4, "unPlot", "Tuesday"], [14, "Plot", "Wednesday"], [6, "unPlot", "Wednesday"], [1, "unPlot", "Thursday"], [19, "Plot", "Thursday"], [28, "Plot", "Friday"], [10, "unPlot", "Friday"], [3, "unPlot", "Saturday"]]
plotList=[]
unPlotList=[]
for i in yourList:
if "Plot" in i:
plotList.append(i)
else:
unPlotList.append(i)
理解時更短或更短:
plotList = [i for i in yourList if "Plot" in i]
unPlotList = [i for i in yourList if "unPlot" in i]
您可以使用列表推導,例如
# old_list elements should be tuples if they're fixed-size, BTW
list1 = [(X, Y, Z) for X, Y, Z in old_list if Y == 'Plot']
list2 = [(X, Y, Z) for X, Y, Z in old_list if Y == 'unPlot']
如果只想遍歷輸入列表一次,那么可能:
def split_list(old_list):
list1 = []
list2 = []
for X, Y, Z in old_list:
if Y == 'Plot':
list1.append((X, Y, Z))
else:
list2.append((X, Y, Z))
return list1, list2
您可以直接瀏覽列表,並檢查值是否為“Plot”,如下所示:
for i in List:
if i[1]=="Plot":
list1.append(i)
else:
list2.append(i)
我有一個輔助函數,用於將列表分成兩部分的一般情況:
def partition(iterable, condition):
def partition_element(partitions, element):
(partitions[0] if condition(element) else partitions[1]).append(element)
return partitions
return reduce(partition_element, iterable, ([], []))
例如:
>>> partition([1, 2, 3, 4], lambda d: d % 2 == 0)
([2, 4], [1, 3])
或者在你的情況下:
>>> partition(your_list, lambda i: i[1] == "Plot")
使用列表理解:
l = [[8, "Plot", "Sunday"], [1, "unPlot", "Monday"], [12, "Plot", "Monday"], [10, "Plot", "Tuesday"], [4, "unPlot", "Tuesday"], [14, "Plot", "Wednesday"], [6, "unPlot", "Wednesday"], [1, "unPlot", "Thursday"], [19, "Plot", "Thursday"], [28, "Plot", "Friday"], [10, "unPlot", "Friday"], [3, "unPlot", "Saturday"]]
list1 = [x for x in l if x[1] == "Plot"]
list2 = [x for x in l if x[1] == "unPlot"]
您也可以使用filter命令執行此操作:
list1 = filter(lambda x: x[1] == "Plot", list)
list2 = filter(lambda x: x[1] == "unPlot", list)
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.