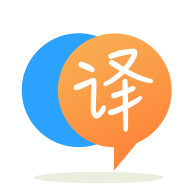
[英]How can I make a custom Pin It button to create the Pinterest overlay?
[英]How can i rerender Pinterest's Pin It button?
我正在嘗試在頁面加載后創建和操作 Pin It 按鈕。 當我使用 js 更改按鈕屬性時,應該重新渲染它以獲得固定動態加載圖像的功能。 那么,Pinterest 有沒有像 Facebook 的 B.XFBML.parse() 函數那樣的方法?
謝謝...
只需將data-pin-build
屬性添加到SCRIPT
標簽:
<script defer
src="//assets.pinterest.com/js/pinit.js"
data-pin-build="parsePinBtns"></script>
這導致pinit.js
將其內部build
函數作為parsePinBtns
函數公開給全局window
對象。
然后,您可以使用它來解析隱式元素中的鏈接或頁面上的所有鏈接:
// parse the whole page
window.parsePinBtns();
// parse links in #pin-it-buttons element only
window.parsePinBtns(document.getElementById('pin-it-buttons'));
提示:要顯示零計數,只需將data-pin-zero="1"
到SCRIPT
標簽。
最好的方法是:
重新編寫他們的腳本 - 即使用 jQuery:
$.ajax({ url: 'http://assets.pinterest.com/js/pinit.js', dataType: 'script', cache:true});
要在頁面加載后呈現 pin-it 按鈕,您可以使用:
<a href="..pin it link.." id="mybutton" class="pin-it-button" count-layout="none">
<img border="0" src="//assets.pinterest.com/images/PinExt.png" width="43" height="21" title="Pin It" />
</a>
<script>
var element = document.getElementById('mybutton');
(function(x){ for (var n in x) if (n.indexOf('PIN_')==0) return x[n]; return null; })(window).f.render.buttonPin(element);
</script>
當然,假設assets.pinterest.com/js/pinit.js已經加載到頁面上。 渲染對象還有一些其他有用的方法,如buttonBookmark 、 buttonFollow 、 ebmedBoard 、 embedPin 、 embedUser 。
我以 Derrek 的解決方案(並修復了未聲明的變量問題)為基礎,使動態加載 pinterest 按鈕成為可能,因此它不可能減慢加載時間。 僅與原始問題相關,但我想無論如何我都會分享。
在文件末尾:
<script type="text/javascript">
addPinterestButton = function (url, media, description) {
var js, href, html, pinJs;
pinJs = '//assets.pinterest.com/js/pinit.js';
//url = escape(url);
url = encodeURIComponent(url);
media = encodeURIComponent(media);
description = encodeURIComponent(description);
href = 'http://pinterest.com/pin/create/button/?url=' + url + '&media=' + media + '&description=' + description;
html = '<a href="' + href + '" class="pin-it-button" count-layout="vertical"><img border="0" src="http://assets.pinterest.com/images/PinExt.png" title="Pin It" /></a>';
$('#pinterestOption').html(html);
//add pinterest js
js = document.createElement('script');
js.src = pinJs;
js.type = 'text/javascript';
document.body.appendChild(js);
}
</script>
在文檔准備功能中:
addPinterestButton('pageURL', 'img', 'description');//replace with actual data
在您希望 pinterest 按鈕出現的文檔中,只需添加一個 id 為 pinterestOption 的元素,即
<div id="pinterestOption"></div>
希望對某人有所幫助!
我重寫了 Pinterest 按鈕代碼,支持加載 AJAX 內容后解析 Pinterest 標簽,類似於 FB.XFBML.parse() 或 gapi.plusone.go()。 作為獎勵,項目中的替代 JavaScript 文件支持 HTML5 有效語法。
這就是我所做的。
首先,我查看了 pinit.js,並確定它用 IFRAME 替換了特殊標記的錨標記。 我想我可以編寫 javascript 邏輯來獲取生成的 iframe 上的 src 屬性使用的主機名。
所以,我根據 pinterest 的正常建議插入了標記,但我將錨標簽放入了一個不可見的 div 中。
<div id='dummy' style='display:none;'>
<a href="http://pinterest.com/pin/create/button/?
url=http%3A%2F%2Fpage%2Furl
&media=http%3A%2F%2Fimage%2Furl"
class="pin-it-button" count-layout="horizontal"></a>
</div>
<script type="text/javascript" src="//assets.pinterest.com/js/pinit.js">
</script>
然后,緊接着,我插入了一個腳本,從注入的 iframe 中提取 pinterest CDN 的主機名。
//
// pint-reverse.js
//
// logic to reverse-engineer pinterest buttons.
//
// The standard javascript module from pinterest replaces links to
// http://pinterest.com/create/button with links to some odd-looking
// url based at cloudfront.net. It also normalizes the URLs.
//
// Not sure why they went through all the trouble. It does not work for
// a dynamic page where new links get inserted. The pint.js code
// assumes a static page, and is designed to run "once" at page creation
// time.
//
// This module spelunks the changes made by that script and
// attempts to replicate it for dynamically-generated buttons.
//
pinterestOptions = {};
(function(obj){
function spelunkPinterestIframe() {
var iframes = document.getElementsByTagName('iframe'),
k = [], iframe, i, L1 = iframes.length, src, split, L2;
for (i=0; i<L1; i++) {
k.push(iframes[i]);
}
do {
iframe = k.pop();
src = iframe.attributes.getNamedItem('src');
if (src !== null) {
split = src.value.split('/');
L2 = split.length;
obj.host = split[L2 - 2];
obj.script = split[L2 - 1].split('?')[0];
//iframe.parentNode.removeChild(iframe);
}
} while (k.length>0);
}
spelunkPinterestIframe();
}(pinterestOptions));
然后,
function getPinMarkup(photoName, description) {
var loc = document.location,
pathParts = loc.pathname.split('/'),
pageUri = loc.protocol + '//' + loc.hostname + loc.pathname,
href = '/' + pathToImages + photoName,
basePath = (pathParts.length == 3)?'/'+pathParts[1]:'',
mediaUri = loc.protocol+'//'+loc.hostname+basePath+href,
pinMarkup;
description = description || null;
pinMarkup = '<iframe class="pin-it-button" ' + 'scrolling="no" ' +
'src="//' + pinterestOptions.host + '/' + pinterestOptions.script +
'?url=' + encodeURIComponent(pageUri) +
'&media=' + encodeURIComponent(mediaUri);
if (description === null) {
description = 'Insert standard description here';
}
else {
description = 'My site - ' + description;
}
pinMarkup += '&description=' + encodeURIComponent(description);
pinMarkup += '&title=' + encodeURIComponent("Pin this " + tagType);
pinMarkup += '&layout=horizontal&count=1">';
pinMarkup += '</iframe>';
return pinMarkup;
}
然后像這樣從 jQuery 使用它:
var pinMarkup = getPinMarkup("snap1.jpg", "Something clever here");
$('#pagePin').empty(); // a div...
$('#pagePin').append(pinMarkup);
嘗試閱讀這篇文章http://dgrigg.com/blog/2012/04/04/dynamic-pinterest-button/它使用一個小 javascript 用一個新按鈕替換 pinterest iframe,然后重新加載 pinit.js 文件。 下面是做這個伎倆的javascript
refreshPinterestButton = function (url, media, description) {
var js, href, html, pinJs;
url = escape(url);
media = escape(media);
description = escape(description);
href = 'http://pinterest.com/pin/create/button/?url=' + url + '&media=' + media + '&description=' + description;
html = '<a href="' + href + '" class="pin-it-button" count-layout="horizontal"><img border="0" src="http://assets.pinterest.com/images/PinExt.png" title="Pin It" /></a>';
$('div.pin-it').html(html);
//remove and add pinterest js
pinJs = $('script[src*="assets.pinterest.com/js/pinit.js"]');
pinJs.remove();
js = document.createElement('script');
js.src = pinJs.attr('src');
js.type = 'text/javascript';
document.body.appendChild(js);
}
這是我所做的.. 對@Derrick Grigg 稍作修改,使其在 AJAX 重新加載后在頁面上的多個 pinterest 按鈕上工作。
refreshPinterestButton = function () {
var url, media, description, pinJs, href, html, newJS, js;
var pin_url;
var pin_buttons = $('div.pin-it a');
pin_buttons.each(function( index ) {
pin_url = index.attr('href');
url = escape(getUrlVars(pin_URL)["url"]);
media = escape(getUrlVars(pin_URL)["media"]);
description = escape(getUrlVars(pin_URL)["description"]);
href = 'http://pinterest.com/pin/create/button/?url=' + url + '&media=' + media + '&description=' + description;
html = '<a href="' + href + '" class="pin-it-button" count-layout="horizontal"><img border="0" src="http://assets.pinterest.com/images/PinExt.png" title="Pin It" /></a>';
index.parent().html(html);
});
//remove and add pinterest js
pinJs = '//assets.pinterest.com/js/pinit.js';
js = $('script[src*="assets.pinterest.com/js/pinit.js"]');
js.remove();
js = document.createElement('script');
js.src = pinJs;
js.type = 'text/javascript';
document.body.appendChild(js);
}
});
function getUrlVars(pin_URL)
{
var vars = [], hash;
var hashes = pin_URL.slice(pin_URL.indexOf('?') + 1).split('&');
for(var i = 0; i < hashes.length; i++)
{
hash = hashes[i].split('=');
vars.push(hash[0]);
vars[hash[0]] = hash[1];
}
return vars;
}
官方的方法是在加載腳本時設置“data-pin-build”屬性:
<script defer="defer" src="//assets.pinterest.com/js/pinit.js" data-pin-build="parsePins"></script>
然后你可以像這樣動態渲染你的按鈕:
// render buttons inside a scoped DOM element
window.parsePins(buttonDomElement);
// render the whole page
window.parsePins();
該站點上還有另一種方法,可以讓您在沒有腳本標記的情況下在 JavaScript 中呈現它們。
他們的pinit.js文件,在他們的“Pin it”按鈕文檔中引用,沒有公開任何全局變量。 它運行一次,除了它創建的 iframe 之外不會留下任何痕跡。
您可以再次注入該文件以“解析”新按鈕。 他們的 JS 在運行時會查看所有錨標記,並將class="pin-it-button"
替換為 iframe 的按鈕。
我試圖調整他們的代碼以相同的方式工作(插入並忘記它),此外您可以調用 Pinterest.init() 以在頁面上添加任何“新”按鈕(例如 ajax 'd in,動態創建等)變成了正確的按鈕。
項目: https : //github.com/onassar/JS-Pinterest原始:https ://raw.github.com/onassar/JS-Pinterest/master/Pinterest.js
截至 2020 年 6 月,Pinterest 將 pin js 代碼更新為 v2。 這就是為什么 data-pin-build 可能不適用於<script defer="defer" src="//assets.pinterest.com/js/pinit.js" data-pin-build="parsePins"></script>
現在它適用於 pinit_v2.js <script async defer src="//assets.pinterest.com/js/pinit_v2.js" data-pin-build="parsePins"></script>
這對我來說很好用: http : //www.mediadevelopment.no/projects/pinit/它獲取點擊事件的所有數據
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.