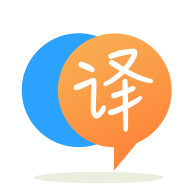
[英]Object deserializtion in Redisson for a class with only parameterized constructor
[英]how to create object for a class with only parameterized constructor?
public final class City
{
public final long id;
public final String name;
public final double latitude;
public final double longitude;
public City(long id, String name, double lat, double lng)
{
this.id = id;
this.name = name;
this.latitude = lat;
this.longitude = lng;
}
public static City fromJsonObject(JSONObject json) throws JSONException
{
return new City(json.getLong(WebApi.Params.CITY_ID),
json.getString(WebApi.Params.CITY_NAME),
json.getDouble(WebApi.Params.LATITUDE),
json.getDouble(WebApi.Params.LONGITUDE));
}
}
這是我的班級。 我想在另一個類中訪問這個類的值; 我怎么做? (緯度,經度,城市名稱和城市名稱的值)
這可以使用instance.member
表示法來完成:(這就像調用方法一樣,只是沒有括號;-)
City c = City.fromJsonObject(...);
system.out.println("City name: " + c.name); // etc.
因為成員被標記為final,所以必須在聲明時或在構造函數中設置它們。 在這種情況下,它們是在工廠(靜態)方法調用的構造函數中設置的。 但是,因為它們是最終的,所以這將是無效的:
City c = ...;
c.name = "Paulville"; // oops (won't compile)! can't assign to final member
盡管有人可能會爭辯說[總是]使用“ getters”,但是由於這是一個[簡單] 不變的對象 ,因此我發現它(直接成員訪問)是一種有效的方法。 然而, 關於“getters”的一個非常好的事情是它們可以在接口中定義,因為它們實際上只是方法。 這個方便的功能對於以后避免ABI重大更改,啟用DI或允許模擬... 可能有用,但不要過度設計:)
(除非絕對必要,否則我通常會避免使用“設定者”……無需引入隨意的可變狀態,並且,如果需要更改狀態,我喜歡通過“操作”進行更改。)
快樂的編碼。
現在,假設這個問題是關於刪除靜態工廠方法同時仍保留最終成員的,那么仍然可以輕松完成(但是,這會引入一個“可能引發異常的構造函數”),這完全是蠕蟲的一種。 ..無論如何,請注意,分配給構造函數中最終成員的要求仍在滿足。
// constructors can (ickickly) throw exceptions ...
public City(JSONObject json) throws JSONException
{
id = json.getLong(WebApi.Params.CITY_ID),
name = json.getString(WebApi.Params.CITY_NAME),
latitude = json.getDouble(WebApi.Params.LATITUDE),
longitude = json.getDouble(WebApi.Params.LONGITUDE));
}
或者也許需要保留/利用工廠方法,同時還提供構造函數重載,但這真的開始變得愚蠢 ......
public City(JSONObject json) throws JSONException
: this(City.fromJsonObject(json)) {
// we do everything in the next constructor, it would be neat if it
// was possible to do static/local stuff before calling the base constructor
// (so that we could pass in multiple arguments, but .. not possible in Java)
}
City(City other) {
// a "copy constructor"
// just copy over the member values, note no exception from here :)
// also note that this fulfills the contract of assigning to the final
// members when used as the base constructor for City(JSONObject)
id = other.id;
name = other.name;
latitude = other.latitude;
longitude = other.longitude;
}
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.