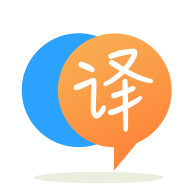
[英]How can I find max and min number without using lists in Python?(user is going to write the numbers)
[英]Python - find greatest 2 of 3 numbers WITHOUT using max()
我正在尋找此處找到的相同問題的答案:
但是,我想構建自己的程序,而不是使用內置函數max()
。 這是我現在所擁有的,但是由於范圍可變,我想拋出一個錯誤。
def two_of_three(a, b, c):
if a>=b:
x=a
else:
x=b
if b>=c:
y=b
else:
y=c
if a>=c:
x=a
else:
x=c
return x**x+y**y
assert two_of_three(3,4,5)==41
assert two_of_three(0,1,2)==5
assert two_of_three(9,21,89)==8362
這是我得到的錯誤:
Traceback (most recent call last):
File "python_hw1.py", line 32, in <module>
assert two_of_three(3,4,5)==41
AssertionError
在Python中, **
表示to the power of
。 您可能正在尋找return x**2 + y**2
Sol.1
def two_biggest(a, b, c):
if a>=b>=c:
print a, b, 'are the biggest two'
elif b>=c>=a:
print b, c, 'are the biggest two'
else:
print c, a, 'are the biggest two'
Sol.2
def two_biggest(a, b, c):
nums = set([a, b, c])
smallest = min(nums) # not max (trollface :P)
nums.remove(smallest)
print "the two largest numbers are", ' and '.join(map(str, nums))
您的代碼中存在一個錯誤,該錯誤允許兩次返回相同的值:
def two_of_three(a, b, c):
if a>=b:
x=a
else:
x=b
if b>=c:
y=b
else:
y=c
if a>=c:
x=a
else:
x=c
print x, y
>>> two_of_three(3,4,5)
#5 5
更新
我尚未測試此內容,因為我正在從手機中進行更新,但是這樣的事情呢?
vals = [3,4,5]
twoLargest = sorted(vals)[-2:]
讓sort函數自然地將最大的末尾放在最后兩個?
def biggestTwo(*args):
return sorted(args)[-2:]
我的解決方案如下:
def two_of_three(a, b, c):
"""Return x**2 + y**2, where x and y are the two largest of a, b, c."""
return sum( map( lambda x: x**2, ( (a, b) if (a>=b>=c) else ((b, c) if (b>= c>=a) else (a, c))) ) )
l=[2,3,8,5,4]
l.sort()
l[-1]
8
如果您需要的是最大數字(而不是變量名),則可以嘗試使用列表推導:
In : (a,b,c)
Out: (7, 3, 8)
In : [x for x in (a,b,c) if (x > a) | (x > b) | ( x > c)]
Out: [7, 8]
您將不得不對此進行調整,具體取決於兩個或多個數字相等時所需的結果(例如7,7,9)
如果問題是避免使用bultin max函數,請編寫自己的函數:
def mymax(l):
x=float("-inf")
for i in l:
if i>x: x=i
return x
如果要在列表中包含n
個最大值的列表,則也可以編寫自己的一個:
def nmax(pl,n=1):
r,l=[],[]
for e in pl:
l.append(e)
for x in range(0,n):
max_found=float("-inf")
for i, v in enumerate(l):
if v>max_found:
max_found=v
max_index=i
r.append(max_found)
del l[max_index]
return r
測試一下:
>>> import random
>>> rl=random.sample(range(1000),10)
>>> print rl
[183, 456, 688, 263, 452, 613, 789, 682, 589, 493]
>>> print nmax(rl,2)
[789, 688]
或者,如果允許使用內置sorted
,則可以在一行中執行以下操作:
>>> sorted(rl)[-2:]
[789, 688]
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.