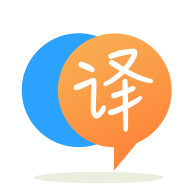
[英]java.lang.outofmemoryerror bitmap size exceeds vm budget on bitmap
[英]ViewPager with ImageView gives “java.lang.OutOfMemoryError: bitmap size exceeds VM budget”
我做了一個ViewPager
以顯示圖像。 當我前進某些頁面時,我得到一個java.lang.OutOfMemoryError: bitmap size exceeds VM budget
錯誤。
關於此問題還有更多問題,但我沒有找到解決方案( BitMap.Recycle
, System.gc()
等)。 如果您有任何建議或解決方案,請告訴我!
PNG是628KB,478KB,587KB,132KB,139KB,149KB,585KB(崩潰)。
如果您還有其他解決方案(滾動類似頁面的圖像),請告訴我!
我的代碼:
package nl.ipear.vngmagazine;
import android.content.Context;
import android.graphics.BitmapFactory;
import android.os.Bundle;
import android.os.Environment;
import android.os.Parcelable;
import android.support.v4.app.FragmentActivity;
import android.support.v4.view.PagerAdapter;
import android.support.v4.view.ViewPager;
import android.util.Log;
import android.view.LayoutInflater;
import android.view.View;
import android.widget.ImageView;
public class ShowMagazine2 extends FragmentActivity {
private ViewPager myPager;
private MyPagerAdapter myPagerAdapter;
private static int NUM_PAGES = 15;
/** Called when the activity is first created. */
@Override
public void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.showmagazine);
myPager = (ViewPager)findViewById(R.id.viewpager1);
myPagerAdapter = new MyPagerAdapter();
myPager.setAdapter(myPagerAdapter);
return;
}
private class MyPagerAdapter extends PagerAdapter{
@Override
public int getCount() {
return NUM_PAGES;
}
@Override
public Object instantiateItem(View collection, int position) {
String location = Environment.getExternalStorageDirectory() + "/MYData/" + "2012-02/";
// Inflate and create the view
LayoutInflater layoutInflater = (LayoutInflater) collection.getContext().getSystemService(Context.LAYOUT_INFLATER_SERVICE);
View view = layoutInflater.inflate(R.layout.magazinepageview, null);
ImageView imageView = (ImageView) view.findViewById(R.id.magazinePageImage);
String fileName = String.format("%s%s%02d%s", location, "2012-02_Page_", position + 1, ".png");
Log.v("PNG", fileName);
imageView.setImageBitmap(BitmapFactory.decodeFile(fileName));
((ViewPager) collection).addView(view,0);
return view;
}
@Override
public void destroyItem(View collection, int position, Object view) {
((ViewPager) collection).removeView((View) view);
}
@Override
public boolean isViewFromObject(View view, Object object) {
return view==((View)object);
}
@Override
public void finishUpdate(View arg0) {}
@Override
public void restoreState(Parcelable arg0, ClassLoader arg1) {}
@Override
public Parcelable saveState() {
return null;
}
@Override
public void startUpdate(View arg0) {}
}
}
在這里找到答案: Android:java.lang.OutOfMemoryError:無法分配20970152可用字節和2MB的23970828字節分配,直到OOM
只需在AndroidManifest內的Application標簽中添加android:hardwareAccelerated="false"
和android:largeHeap="true"
:
<application
android:allowBackup="true"
android:icon="@mipmap/ic_launcher"
android:label="@string/app_name"
android:supportsRtl="true"
android:theme="@style/AppTheme"
android:hardwareAccelerated="false"
android:largeHeap="true">
看來您的ViewPager正在加載在位圖中,但在滾動瀏覽時未釋放任何內容。
我建議您限制當前可見頁面兩邊可用的頁面數量,這將使系統能夠清除其他頁面中的位圖。
確保銷毀“活動/片段”以解決OOM問題時,您正在回收位圖。
是的,跟隨Mimminito。 我建議2頁,因為3頁的來回渲染速度很快。
現在,如果您需要無延遲的圖像並且圖像已在Internet上。 確保下載它並將其放入internalStorageDevice內,然后重新使用(如果存在)。
對於內存泄漏問題,我的最高答案可能是錯誤的。 這個新答案可能是內存泄漏的原因,原因是圖像的高度和寬度。
private byte[] resizeImage( byte[] input ) {
if ( input == null ) {
return null;
}
Bitmap bitmapOrg = BitmapFactory.decodeByteArray(input, 0, input.length);
if ( bitmapOrg == null ) {
return null;
}
int height = bitmapOrg.getHeight();
int width = bitmapOrg.getWidth();
int newHeight = 250;
float scaleHeight = ((float) newHeight) / height;
// creates matrix for the manipulation
Matrix matrix = new Matrix();
// resize the bit map
matrix.postScale(scaleHeight, scaleHeight);
// recreate the new Bitmap
Bitmap resizedBitmap = Bitmap.createBitmap(bitmapOrg, 0, 0,
width, height, matrix, true);
bitmapOrg.recycle();
ByteArrayOutputStream bos = new ByteArrayOutputStream();
resizedBitmap.compress(CompressFormat.PNG, 0 /*ignored for PNG*/, bos);
resizedBitmap.recycle();
return bos.toByteArray();
}
此頂部代碼用於重新縮放位圖,“ newHeight”是賦予位圖的新高度。
現在,如果這不起作用,我現在100%確定OP必須使用Android內存可以處理的更多視圖來重載ViewPager。 解決方案是使用ViewFlipper並使用我上面所述的答案。
嘗試縮小您的位圖,它確實解決了問題,這是您的操作方法。
BitmapFactory.Options options = new BitmapFactory.Options();
options.inJustDecodeBounds = true;
BitmapFactory.decodeFile( filename, options );
options.inJustDecodeBounds = false;
options.inSampleSize = 2;
bitmap = BitmapFactory.decodeFile( filename, options );
if ( bitmap != null && exact ) {
bitmap = Bitmap.createScaledBitmap( bitmap, width, height, false );
}
將樣本大小調整為任意大小,例如4、8等
在oncreate()中調用System.gc()
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.