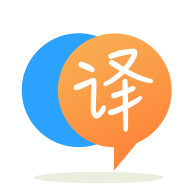
[英]How to return an array, without allocating it, if size is unknown to caller?
[英]Allocating an array of an unknown size
上下文:我正在嘗試做一個程序,將文本作為輸入並將其存儲在字符數組中。 然后我將數組的每個元素打印為小數。 例如,“ Hello World”將轉換為72、101等。我將其用作快速ASCII2DEC轉換器。 我知道有在線轉換器,但我正在嘗試自己制作一個。
問題:如何分配在編譯時大小未知的數組,並使其與輸入的文本大小完全相同? 因此,當我輸入“ Hello World”時,它將動態創建一個具有僅存儲“ Hello World”所需大小的數組。 我已經在網上搜索了,但找不到任何可以利用的東西。
我看到您正在使用C。您可以執行以下操作:
#define INC_SIZE 10
char *buf = (char*) malloc(INC_SIZE),*temp;
int size = INC_SIZE,len = 0;
char c;
while ((c = getchar()) != '\n') { // I assume you want to read a line of input
if (len == size) {
size += INC_SIZE;
temp = (char*) realloc(buf,size);
if (temp == NULL) {
// not enough memory probably, handle it yourself
}
buf = temp;
}
buf[len++] = c;
}
// done, note that the character array has no '\0' terminator and the length is represented by `len` variable
通常,在沒有很大內存限制的PC之類的環境中,我將動態分配(取決於語言)數組/字符串/任何值(例如64K),並保持索引/指針/任何值到當前端點加一-即。 下一個索引/位置以放置任何新數據。
如果使用cpp語言,則可以使用字符串存儲輸入字符,並通過operator []訪問字符,如以下代碼所示:
std::string input;
cin >> input;
我猜你的意思是C,因為這是您遇到此問題的最常見的編譯語言之一。
您在函數中聲明的變量存儲在堆棧中 。 這是一種很好且有效的方法,可以在函數退出時進行清理等。唯一的問題是每個函數的堆棧插槽大小都是固定的,並且在函數運行時無法更改。
可以分配內存的第二個位置是堆 。 這是萬能的,您可以在運行時從中分配和取消分配內存。 您使用malloc()進行分配,完成后,您對其調用free()(這對於避免內存泄漏很重要)。
使用堆分配時,您必須在分配時知道大小,但這比將其存儲在固定的堆棧空間中要好得多,如果需要,該空間不能增長。
這是一個簡單而愚蠢的函數,它使用動態分配的緩沖區將字符串解碼為其ASCII碼:
char* str_to_ascii_codes(char* str)
{
size_t i;
size_t str_length = strlen(str);
char* ascii_codes = malloc(str_length*4+1);
for(i = 0; i<str_length; i++)
snprintf(ascii_codes+i*4, 5, "%03d ", str[i]);
return ascii_codes;
}
編輯:您在評論中提到想要使緩沖區恰到好處。 通過使字符串中的每個條目具有已知的長度,而不修剪結果的多余空格字符,我與上述示例截然不同。 這是一個更智能的版本,可以解決以下兩個問題:
char* str_to_ascii_codes(char* str)
{
size_t i;
int written;
size_t str_length = strlen(str), ascii_codes_length = 0;
char* ascii_codes = malloc(str_length*4+1);
for(i = 0; i<str_length; i++)
{
snprintf(ascii_codes+ascii_codes_length, 5, "%d %n", str[i], &written);
ascii_codes_length = ascii_codes_length + written;
}
/* This is intentionally one byte short, to trim the trailing space char */
ascii_codes = realloc(ascii_codes, ascii_codes_length);
/* Add new end-of-string marker */
ascii_codes[ascii_codes_length-1] = '\0';
return ascii_codes;
}
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.