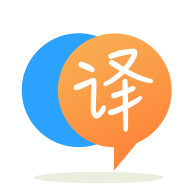
[英]Python error when playing music with Pyglet: UserWarning: exception: access violation reading 0x00000014
[英]Playing music with Pyglet and Tkinter in Python
我想用播放和停止按鈕創建一個簡單的GUI,以python播放mp3文件。 我使用Tkinter創建了一個非常簡單的GUI,它由2個按鈕(停止和播放)組成。
我創建了一個執行以下操作的函數:
def playsound () :
sound = pyglet.media.load('music.mp3')
sound.play()
pyglet.app.run()
我將該功能作為命令添加到按鈕播放中。 我還做了其他功能來停止音樂:
def stopsound ():
pyglet.app.exit
我將此功能作為命令添加到第二個按鈕。 但是問題是,當我打play時,python和gui凍結了。 我可以嘗試關閉窗口,但它不會關閉,並且停止按鈕沒有響應。 我知道這是因為pyglet.app.run()一直執行到歌曲結束,但是我如何才能防止這種情況發生? 我想讓gui在單擊按鈕時停止播放音樂。 關於在哪里可以找到解決方案的任何想法?
您正在將兩個UI庫混合在一起-本質上不是很糟糕,但是存在一些問題。 值得注意的是,它們兩個都需要自己的主循環來處理事件。 TKinter使用它與桌面事件和用戶生成的事件進行通信,在這種情況下,pyglet使用它來播放音樂。
這些循環中的每一個都會阻止正常的“自上而下”的程序流,就像我們在學習非GUI編程時所習慣的那樣,該程序應基本上從主循環進行回調。 在這種情況下,在Tkinter回調的中間,將pyglet主循環(調用pyglet.app.run
)置於運動中,控件將永遠不會返回到Tkinter庫。
有時,不同庫的循環可以在同一進程中共存,而不會發生沖突-但是,您當然可以運行其中一個,也可以運行另一個。 如果是這樣,則有可能在不同的Python線程中運行每個庫的mainloop。
如果它們不能一起存在,則必須以不同的過程處理每個庫。
因此,使音樂播放器從另一個線程啟動的一種方法可能是:
from threading import Thread
def real_playsound () :
sound = pyglet.media.load('music.mp3')
sound.play()
pyglet.app.run()
def playsound():
global player_thread
player_thread = Thread(target=real_playsound)
player_thread.start()
如果Tkinter和pyglet可以共存,那么足以讓您的音樂開始。 為了能夠控制它,您將需要實現更多的功能。 我的建議是在pyglet每秒調用一次pyglet線程上進行一次回調-此回調檢查某些全局變量的狀態,並根據它們選擇停止音樂,更改正在播放的文件等等。上。
我會做類似的事情:
import pyglet
from pyglet.gl import *
class main (pyglet.window.Window):
def __init__ (self):
super(main, self).__init__(800, 600, fullscreen = False)
self.button_texture = pyglet.image.load('button.png')
self.button = pyglet.sprite.Sprite(self.button_texture)
self.sound = pyglet.media.load('music.mp3')
self.sound.play()
self.alive = 1
def on_draw(self):
self.render()
def on_close(self):
self.alive = 0
def on_mouse_press(self, x, y, button, modifiers):
if x > self.button.x and x < (self.button.x + self.button_texture.width):
if y > self.button.y and y < (self.button.y + self.button_texture.height):
self.alive = 0
def on_key_press(self, symbol, modifiers):
if symbol == 65307: # [ESC]
self.alive = 0
def render(self):
self.clear()
self.button.draw()
self.flip()
def run(self):
while self.alive == 1:
self.render()
# -----------> This is key <----------
# This is what replaces pyglet.app.run()
# but is required for the GUI to not freeze
#
event = self.dispatch_events()
x = main()
x.run()
此解決方案是最簡單的一種:
import pyglet
foo=pyglet.media.load("/data/Me/Music/Goo Goo Dolls/[1998] Dizzy Up The Girl/11 - Iris.mp3")
foo.play()
def exiter(dt):
pyglet.app.exit()
print "Song length is: %f" % foo.duration
# foo.duration is the song length
pyglet.clock.schedule_once(exiter, foo.duration)
pyglet.app.run()
pyglet文檔中有一個媒體播放器實現:
http://www.pyglet.org/doc/programming_guide/playing_sounds_and_music.html
您應該查看的腳本是media_player.py
希望這會幫助您入門
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.