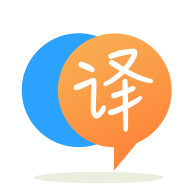
[英]Open a shared link in facebook to open in native app if installled in iphone
[英]Open a facebook link by native Facebook app on iOS
如果本機 Facebook 應用程序安裝在 iPhone 上。 如何從我的應用程序打開 Facebook 鏈接到本機 Facebook 應用程序。 在Safari打開的情況下,鏈接與:
謝謝你。
以下是 Facebook 應用程序使用的一些方案,源鏈接上還有更多內容:
NSURL *url = [NSURL URLWithString:@"fb://profile/<id>"];
[[UIApplication sharedApplication] openURL:url];
fb://profile – 打開 Facebook 應用到用戶的個人資料
fb://friends – 打開 Facebook 應用到好友列表
fb://notifications – 打開 Facebook 應用到通知列表(注意:此 URL 似乎存在錯誤。通知頁面打開。但是,無法導航到 Facebook 應用中的其他任何位置)
fb://feed – 打開 Facebook 應用到新聞提要
fb://events – 打開 Facebook 應用到活動頁面
fb://requests – 打開 Facebook 應用到請求列表
fb://notes – 打開 Facebook 應用到 Notes 頁面
fb://albums – 打開 Facebook 應用到相冊列表
如果在打開此 url 之前您想檢查用戶是否使用 facebook 應用程序,您可以執行以下操作(如下面的另一個答案所述):
if ([[UIApplication sharedApplication] canOpenURL:nsurl]){
[[UIApplication sharedApplication] openURL:nsurl];
}
else {
//Open the url as usual
}
只需使用https://graph.facebook.com/(your_username or page name) 獲取您的頁面 ID,然后您就可以看到所有的拘留和您的 ID
在您的 IOS 應用程序中使用后:
NSURL *url = [NSURL URLWithString:@"fb://profile/[your ID]"];
[[UIApplication sharedApplication] openURL:url];
要將 yonix 的評論添加為答案,舊的fb://page/…
URL 不再有效。 顯然它被fb://profile/…
取代,即使頁面不是個人資料。
我用下面的方法在 MonoTouch 中做到了這一點,我確信基於純 Obj-C 的方法不會有太大的不同。 我在一個有時會更改 URL 的類中使用它,這就是為什么我沒有將它放在 if/elseif 語句中。
NSString *myUrls = @"fb://profile/100000369031300|http://www.facebook.com/ytn3rd";
NSArray *urls = [myUrls componentsSeparatedByString:@"|"];
for (NSString *url in urls){
NSURL *nsurl = [NSURL URLWithString:url];
if ([[UIApplication sharedApplication] canOpenURL:nsurl]){
[[UIApplication sharedApplication] openURL:nsurl];
break;
}
}
在您的應用程序更改為 Safari/Facebook 之前,並不總是調用中斷。 我假設您的程序將在那里停止並在您返回時調用它。
我知道這個問題很老,但為了支持上述答案,我想分享我的工作代碼。 只需將這兩個方法添加到任何類。代碼檢查是否安裝了 facebook 應用程序,如果未安裝,則在瀏覽器中打開 url。如果在嘗試查找 profileId 時發生任何錯誤,頁面將在瀏覽器中打開。 只需將 url(例如, http://www.facebook.com/AlibabaUS )傳遞給 openUrl: 它將發揮所有作用。 希望它可以幫助某人!
注意: UrlUtils 是為我提供代碼的類,您可能需要將其更改為其他內容以滿足您的需要。
+(void) openUrlInBrowser:(NSString *) url
{
if (url.length > 0) {
NSURL *linkUrl = [NSURL URLWithString:url];
[[UIApplication sharedApplication] openURL:linkUrl];
}
}
+(void) openUrl:(NSString *) urlString
{
//check if facebook app exists
if ([[UIApplication sharedApplication] canOpenURL:[NSURL URLWithString:@"fb://"]]) {
// Facebook app installed
NSArray *tokens = [urlString componentsSeparatedByString:@"/"];
NSString *profileName = [tokens lastObject];
//call graph api
NSURL *apiUrl = [NSURL URLWithString:[NSString stringWithFormat:@"https://graph.facebook.com/%@",profileName]];
NSData *apiResponse = [NSData dataWithContentsOfURL:apiUrl];
NSError *error = nil;
NSDictionary *jsonDict = [NSJSONSerialization JSONObjectWithData:apiResponse options:NSJSONReadingMutableContainers error:&error];
//check for parse error
if (error == nil) {
NSString *profileId = [jsonDict objectForKey:@"id"];
if (profileId.length > 0) {//make sure id key is actually available
NSURL *fbUrl = [NSURL URLWithString:[NSString stringWithFormat:@"fb://profile/%@",profileId]];
[[UIApplication sharedApplication] openURL:fbUrl];
}
else{
[UrlUtils openUrlInBrowser:urlString];
}
}
else{//parse error occured
[UrlUtils openUrlInBrowser:urlString];
}
}
else{//facebook app not installed
[UrlUtils openUrlInBrowser:urlString];
}
}
迅捷 3
if let url = URL(string: "fb://profile/<id>") {
if #available(iOS 10, *) {
UIApplication.shared.open(url, options: [:],completionHandler: { (success) in
print("Open fb://profile/<id>: \(success)")
})
} else {
let success = UIApplication.shared.openURL(url)
print("Open fb://profile/<id>: \(success)")
}
}
如果已登錄 Facebook 應用程序,則執行以下代碼時將打開該頁面。 如果在執行代碼時 Facebook 應用程序沒有登錄,那么用戶將被重定向到 Facebook 應用程序登錄,然后在連接 Facebook 后不會重定向到頁面!
NSURL *fbNativeAppURL = [NSURL URLWithString:@"fb://page/yourPageIDHere"] [[UIApplication sharedApplication] openURL:fbNativeAppURL]
今天剛剛驗證了這一點,但是如果您嘗試打開 Facebook頁面,則可以使用“fb://page/{Page ID}”
頁面 ID 可以在頁面下方靠近底部的關於部分中找到。
具體到我的用例,在 Xamarin.Forms 中,您可以使用此代碼段在應用程序中打開(如果可用),否則在瀏覽器中打開。
Device.OpenUri(new Uri("fb://page/{id}"));
以前的方法fb://profile/[ID]
不起作用。
試試fb://profile?id=[ID]
用法示例:
// Open Profile or page
// To open in Application
if UIApplication.shared.canOpenURL(URL(string: "fb://profile?id=vikramchaudhary.ios")!) {
UIApplication.shared.open(URL(string: "fb://profile?id=vikramchaudhary.ios")!, options: [:], completionHandler: nil)
} else {
// To open in safari
UIApplication.shared.open(URL(string: "https://facebook.com/vikramchaudhary.ios/")!, options: [:], completionHandler: nil)
}
這將在 Safari 中打開 URL:
[[UIApplication sharedApplication] openURL:[NSURL URLWithString:@"http://www.facebook.com/comments.php?href=http://wombeta.jiffysoftware.com/ViewWOMPrint.aspx?WPID=317"]];
對於 iPhone,如果使用以 fb:// 開頭的 url 安裝,您可以啟動 Facebook 應用程序
更多信息可以在這里找到: http : //iphonedevtools.com/?p=302也在這里: http : //wiki.akosma.com/IPhone_URL_Schemes#Facebook
從上述網站竊取:
fb://profile – Open Facebook app to the user’s profile
fb://friends – Open Facebook app to the friends list
fb://notifications – Open Facebook app to the notifications list (NOTE: there appears to be a bug with this URL. The Notifications page opens. However, it’s not possible to navigate to anywhere else in the Facebook app)
fb://feed – Open Facebook app to the News Feed
fb://events – Open Facebook app to the Events page
fb://requests – Open Facebook app to the Requests list
fb://notes - Open Facebook app to the Notes page
fb://albums – Open Facebook app to Photo Albums list
要啟動上述 URL:
NSURL *theURL = [NSURL URLWithString:@"fb://<insert function here>"];
[[UIApplication sharedApplication] openURL:theURL];
2020 答案
直接打開網址。 Facebook 會自動注冊深層鏈接。
let url = URL(string:"https://www.facebook.com/TheGoodLordAbove")!
UIApplication.shared.open(url,completionHandler:nil)
如果已安裝,這將在 Facebook 應用程序中打開,否則將在您的默認瀏覽器中打開
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.