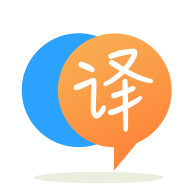
[英]How to enable and disable CheckBox in the GridView using JavaScript?
[英]How to disable/enable form elements using a checkbox with Javascript?
我不知所措 - 在這個問題上我的大腦被砸了幾個小時后,是時候把問題提到這里了。
我試圖讓一個復選框執行一些javascript代碼,當選中它們時,啟用一些表單元素,並且當取消選中時,再次禁用這些元素。
這是我的javascript:
function houseclean()
{
if (document.orderform.cleaning.checked == true)
{
document.orderform.rooms.disabled = false;
document.orderform.datetime.disabled = false;
document.orderform.howoften.disabled = false;
}
else
{
document.orderform.rooms.disabled = true;
document.orderform.datetime.disabled = true;
document.orderform.howoften.disabled = true;
}
}
這是我的HTML代碼:
<form id="orderform" style="margin-left: 6cm">
<input type="checkbox" name="cleaning" value="yes" onclick="houseclean()"/> Home cleaning <br />
Service: <select name="rooms" id="rooms" disabled >
<option value="1bedroom">One Bedroom Apt</option>
<option value="2bedroom">Two Bedroom Apt</option>
<option value="3bedroom">Three Bedroom Townhome or SF Home</option>
<option value="4bedroom">Four Bedroom Home</option>
<option value="5bedroom">Five Bedroom Home</option>
<option value="6bedroom">Six Bedroom Home</option>
</select> <br />
Date / Time: <input type="text" name="datetime" disabled /><br />
How often would you like a cleaning?: <select name="howoften" disabled>
<option value="once">One Time Only</option>
<option value="2bedroom">Every Week</option>
<option value="3bedroom">Every Other Week</option>
<option value="4bedroom">Once a Month</option>
</select> <br />
</form>
這段代碼可以解決問題。 您應該明確說明您的元素ID,並盡可能使用getElementById。
HTML:
<form id="orderform" style="margin-left: 6cm;">
<input type="checkbox" id="cleaning" name="cleaning" value="yes" onclick="javascript:houseclean();"/> Home cleaning <br />
Service: <select name="rooms" id="rooms" disabled >
<option value="1bedroom">One Bedroom Apt</option>
<option value="2bedroom">Two Bedroom Apt</option>
<option value="3bedroom">Three Bedroom Townhome or SF Home</option>
<option value="4bedroom">Four Bedroom Home</option>
<option value="5bedroom">Five Bedroom Home</option>
<option value="6bedroom">Six Bedroom Home</option>
</select> <br />
Date / Time: <input type="text" id="datetime" name="datetime" disabled /><br />
How often would you like a cleaning?: <select id="howoften" name="howoften" disabled>
<option value="once">One Time Only</option>
<option value="2bedroom">Every Week</option>
<option value="3bedroom">Every Other Week</option>
<option value="4bedroom">Once a Month</option>
</select> <br />
</form>
和javascript:
function houseclean()
{
if (document.getElementById('cleaning').checked == true)
{
document.getElementById('rooms').removeAttribute('disabled');
document.getElementById('datetime').removeAttribute('disabled');
document.getElementById('howoften').removeAttribute('disabled');
}
else
{
document.getElementById('rooms').setAttribute('disabled','disabled');
document.getElementById('datetime').setAttribute('disabled','disabled');
document.getElementById('howoften').setAttribute('disabled','disabled');
}
}
JSFiddle示例: http : //jsfiddle.net/HQQ4n/10/
我讓你的代碼工作。 我將id
屬性添加到表單元素中,並使用document.getElementById
來保證安全。
JFiddle示例: http : //jsfiddle.net/vxRjw/1/
不是超級健壯,而是一個動態的jquery解決方案......
$("#mycheckbox").click(function() {
enableFormElements("#myform", $(this).attr('checked'));
});
function enableFormElements(form, enable) {
var fields = ["checkbox","textarea","select"];
var selector = form+" input";
$.each(fields, function(i,e) { selector += ","+form+" "+e; });
$(selector).each(function(idx) {
if (enable) {
$(this).removeAttr("disabled");
$(this).removeAttr("readonly");
} else {
$(this).attr("disabled","disabled");
$(this).attr("readonly","readonly");
}
if ($(this).is("select") || $(this).is("textarea")) {
var id = $(this).attr("id");
var txt_equiv = id+"_text";
var val = $(this).find("option[value='"+$(this).val()+"']").text();
// dynamically add the span to this element...so you can switch back and forth...
if ($(this).parent().find("span").length == 0) {
$(this).parent().append("<span class='record_display_text' id='"+txt_equiv+"'>"+val+"</span>");
}
}
if ($("#"+txt_equiv).length != 0) {
if (enable) {
$("#"+id).show();
$("#"+txt_equiv).hide();
} else {
$("#"+txt_equiv).show();
$("#"+id).hide();
}
}
});
}
該函數基本上查找所提供的元素內的所有輸入,選擇,textareas,並禁用或啟用每個。 如果使用JQuery 1.9,您可能希望將它們更改為prop設置,但我發現並非所有瀏覽器都在使用它。 (的Bleh)。 在textareas或choose的情況下,它實際上創建了一個相關的span,其id與輸入匹配,除了后面帶有“_text”。 如果您的頁面上有其他可能存在沖突的ID,您可能需要對其進行編輯...但是將其置於適當的位置后,代碼可以隱藏/顯示文本或實際的select / textarea,具體取決於您是否要啟用所有內容或者禁用。 同樣,沒有在所有情況下進行測試,但可以隨意復制和編輯...
哦,並確保所有選擇或textareas是他們父母的唯一孩子...如果你必須在一個范圍內換行,因為它在同一個父母中動態添加相關的跨度......所以如果select或textarea是' t包裹在父級(如span,div或td或其他東西)中,然后它可能會將生成的文本跨度隨機拋出。 :P
然后你可以添加一些CSS來使表單元素看起來更像你的普通頁面或標准span元素...
input[readonly],
select[readonly],
textarea[readonly] {
cursor: default;
}
input[disabled] {
background-color:#F0F0F0;
border:none;
-moz-box-shadow: none;
-webkit-box-shadow:none;
box-shadow:none;
padding: 0;
}
您可能希望更改背景顏色以匹配您的頁面顏色...而“游標:默認”一個是如果bootstrap twitter正在添加惱人的“受限制”光標或其他任何殘障元素......但無論如何,這是我今晚提出的解決方案,它對我有用。 :)
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.