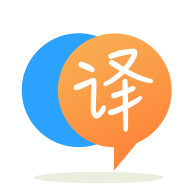
[英]Spring: link between WebApplicationContext and ApplicationContext?
[英]What is the difference between ApplicationContext and WebApplicationContext in Spring MVC?
應用程序上下文和Web應用程序上下文之間有什么區別?
我知道WebApplicationContext
用於面向Spring MVC體系結構的應用程序嗎?
我想知道MVC應用程序中ApplicationContext
的用途是什么? 在ApplicationContext
中定義了哪種bean?
Web應用程序上下文擴展了應用程序上下文,該上下文旨在與標准javax.servlet.ServletContext一起使用,因此能夠與容器進行通信。
public interface WebApplicationContext extends ApplicationContext {
ServletContext getServletContext();
}
如果WebBean中實現ServletContextAware接口,則在WebApplicationContext中實例化的Bean也將能夠使用ServletContext。
package org.springframework.web.context;
public interface ServletContextAware extends Aware {
void setServletContext(ServletContext servletContext);
}
ServletContext實例可以做很多事情,例如通過調用getResourceAsStream()方法來訪問WEB-INF資源(xml配置等)。 通常,在Servlet Spring應用程序的web.xml中定義的所有應用程序上下文都是Web應用程序上下文,這既適用於根Webapp上下文,也適用於Servlet的應用程序上下文。
此外,取決於Web應用程序上下文的功能,可能會使您的應用程序難以測試,並且可能需要使用MockServletContext類進行測試。
servlet和根上下文之間的區別 Spring允許您構建多級應用程序上下文層次結構,因此,如果當前應用程序上下文中不存在所需的bean,則會從父上下文中獲取所需的bean。 在Web應用程序中,默認情況下有兩個層次結構級別,即根和Servlet上下文: 。
這使您可以將某些服務作為整個應用程序的單例運行(Spring Security Bean和基本數據庫訪問服務通常位於此處),而另一項則作為相應服務中的單獨服務運行,以避免Bean之間發生名稱沖突。 例如,一個Servlet上下文將為網頁提供服務,而另一個將實現無狀態Web服務。
當使用spring servlet類時,這兩個級別的分離是開箱即用的:要配置根應用程序上下文,應在web.xml中使用context-param標記。
<context-param>
<param-name>contextConfigLocation</param-name>
<param-value>
/WEB-INF/root-context.xml
/WEB-INF/applicationContext-security.xml
</param-value>
</context-param>
(根應用程序上下文是由ContextLoaderListener創建的,它在web.xml中聲明
<listener>
<listener-class>org.springframework.web.context.ContextLoaderListener</listener-class>
</listener>
)和Servlet應用程序上下文的servlet標簽
<servlet>
<servlet-name>myservlet</servlet-name>
<servlet-class>org.springframework.web.servlet.DispatcherServlet</servlet-class>
<init-param>
<param-name>contextConfigLocation</param-name>
<param-value>app-servlet.xml</param-value>
</init-param>
</servlet>
請注意,如果將省略init-param,那么在此示例中spring將使用myservlet-servlet.xml。
另請參閱: Spring Framework中applicationContext.xml和spring-servlet.xml之間的區別
回到Servlet時代,web.xml只能有一個<context-param>
,因此,當服務器加載應用程序時,只有一個上下文對象被創建,並且該上下文中的數據在所有資源之間共享(例如:Servlet和JSP)。 它與在上下文中具有數據庫驅動程序名稱相同,但不會更改。 以類似的方式,當我們在<contex-param>
聲明contextConfigLocation參數時,Spring將創建一個Application Context對象。
<context-param>
<param-name>contextConfigLocation</param-name>
<param-value>com.myApp.ApplicationContext</param-value>
</context-param>
一個應用程序中可以有多個Servlet。 例如,您可能想以一種方式處理/ secure / *請求,而以另一種方式處理/ non-seucre / *。 對於每個這些Servlet,您都可以有一個上下文對象,即WebApplicationContext。
<servlet>
<servlet-name>SecureSpringDispatcher</servlet-name>
<servlet-class>org.springframework.web.servlet.DispatcherServlet</servlet-class>
<init-param>
<param-name>contextClass</param-name>
<param-value>com.myapp.secure.SecureContext</param-value>
</init-param>
</servlet>
<servlet-mapping>
<servlet-name>SecureSpringDispatcher</servlet-name>
<url-pattern>/secure/*</url-pattern>
</servlet-mapping>
<servlet>
<servlet-name>NonSecureSpringDispatcher</servlet-name>
<servlet-class>org.springframework.web.servlet.DispatcherServlet</servlet-class>
<init-param>
<param-name>contextClass</param-name>
<param-value>com.myapp.non-secure.NonSecureContext</param-value>
</init-param>
</servlet>
<servlet-mapping>
<servlet-name>NonSecureSpringDispatcher</servlet-name>
<url-pattern>/non-secure/*</url-patten>
</servlet-mapping>
可接受的答案是通過的,但對此有官方解釋:
WebApplicationContext是純ApplicationContext的擴展,具有Web應用程序必需的一些額外功能。 它與常規ApplicationContext的不同之處在於,它能夠解析主題(請參閱使用主題),並且知道它與哪個Servlet關聯(通過鏈接到ServletContext)。 WebApplicationContext綁定在ServletContext中,並且通過在RequestContextUtils類上使用靜態方法,可以隨時在需要訪問WebApplicationContext的情況下查找該WebApplicationContext。
順便說servlet和根上下文都是 web應用上下文:
ApplicationContext(根應用程序上下文):每個Spring MVC Web應用程序都有一個applicationContext.xml文件,該文件配置為上下文配置的根。 Spring加載此文件並為整個應用程序創建一個applicationContext。 該文件由ContextLoaderListener加載,該文件在web.xml文件中配置為上下文參數。 每個Web應用程序只有一個applicationContext。
WebApplicationContext:WebApplicationContext是可感知Web的應用程序上下文,即它具有servlet上下文信息。 一個Web應用程序可以具有多個WebApplicationContext,並且每個Dispatcher Servlet(它是Spring MVC體系結構的前端控制器)都與一個WebApplicationContext相關聯。 webApplicationContext配置文件* -servlet.xml特定於DispatcherServlet。 而且,由於一個Web應用程序可以將多個調度程序Servlet配置為服務多個請求,因此每個Web應用程序可以有多個webApplicationContext文件。
由WebApplicationContext
接口指定的Web應用程序上下文是Web應用程序的Spring應用程序上下文。 假定WebApplicationContext
接口擴展了ApplicationContext
接口,並具有為Web應用程序檢索標准Servlet API ServletContext
的方法,它具有常規Spring應用程序上下文的所有屬性。
除了標准的Spring bean范圍singleton
和prototype
之外,Web應用程序上下文中還有三個可用范圍:
request
-作用域一個bean定義到單個HTTP請求的生命周期; 也就是說,每個HTTP請求都有一個在單個bean定義后面創建的bean實例。 session
-作用域一個bean定義到一個HTTP會話的生命周期 application
-將單個bean定義的作用域限定為ServletContext
的生命周期
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.