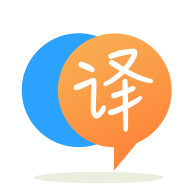
[英]Gesture Recognizer Pinch and Pan at same pic - loosing centre for pinching
[英]Is there a gesture recognizer that handles both pinch and pan together?
所以我正在使用iOS 4.2來為我的應用程序添加縮放和平移。 我已經實現了UIPinchGestureRecognizer和UIPanGestureRecognizer的實例。 在我看來,其中只有一個是一次識別一個手勢。 特別地,后者僅在一個手指向下時作出反應,而前者在第二手指存在時作出反應。 這沒關系,但它有一些副作用,我認為這會產生低劣的用戶體驗質量。
當您放下兩根手指然后移動其中一根手指時,圖像會像應該的那樣展開(放大),但手指下方的像素不再位於手指下方。 圖像從圖像中心縮放,而不是兩個手指之間的中點。 而這個中心點本身就在發展。 我希望中心點的運動能夠決定整體圖像的平移。
幾乎所有iOS應用程序都有相同的行為,圖像放大或縮小圖像中心周圍而不是手指跟蹤手指下方的像素?
在我看來,創建一個自定義手勢識別器是解決這個問題的正確設計方法,但在我看來,有人會創建這樣的識別器,以便商業免費下載和使用。 有沒有這樣的UIGestureRecognizer?
抱歉,這是我用於其中一個演示應用程序的代碼,它可以同時捏縮放和平移,而無需使用scrollview。
不要忘記遵守UIGestureRecognizerDelegate協議
如果你無法同時獲得捏和平移,也許是因為你錯過了這種方法:
-(BOOL)gestureRecognizer:(UIGestureRecognizer *)gestureRecognizer shouldRecognizeSimultaneouslyWithGestureRecognizer:(UIGestureRecognizer *)otherGestureRecognizer
{
return YES;
}
這是完整的源代碼:
#import "ViewController.h"
#import <QuartzCore/QuartzCore.h>
@interface ViewController ()
@end
@implementation ViewController
- (void)viewDidLoad
{
[super viewDidLoad];
// Do any additional setup after loading the view, typically from a nib.
isEditing = false;
photoView = [[UIImageView alloc] initWithFrame:CGRectMake(0, 0, 320, 460)];
[photoView setImage:[UIImage imageNamed:@"photo.png"]];
photoView.hidden = YES;
maskView = [[UIImageView alloc] initWithFrame:CGRectMake(0, 0, 320, 460)];
[maskView setImage:[UIImage imageNamed:@"maskguide.png"]];
maskView.hidden = YES;
displayImage = [[UIImageView alloc] initWithFrame:CGRectMake(0, 0, 320, 460)];
UIPanGestureRecognizer *panGesture = [[UIPanGestureRecognizer alloc] initWithTarget:self action:@selector(handlePan:)];
UIPinchGestureRecognizer *pinchGesture = [[UIPinchGestureRecognizer alloc] initWithTarget:self action:@selector(handlePinch:)];
[panGesture setDelegate:self];
[pinchGesture setDelegate:self];
[photoView addGestureRecognizer:panGesture];
[photoView addGestureRecognizer:pinchGesture];
[photoView setUserInteractionEnabled:YES];
[panGesture release];
[pinchGesture release];
btnEdit = [[UIButton alloc] initWithFrame:CGRectMake(60, 400, 200, 50)];
[btnEdit setBackgroundColor:[UIColor blackColor]];
[btnEdit setTitle:@"Start Editing" forState:UIControlStateNormal];
[btnEdit addTarget:self action:@selector(toggleEditing) forControlEvents:UIControlEventTouchUpInside];
[[self view] addSubview:displayImage];
[[self view] addSubview:photoView];
[[self view] addSubview:maskView];
[[self view] addSubview:btnEdit];
[self updateMaskedImage];
}
- (void)viewDidUnload
{
[super viewDidUnload];
// Release any retained subviews of the main view.
}
- (BOOL)shouldAutorotateToInterfaceOrientation:(UIInterfaceOrientation)interfaceOrientation
{
return (interfaceOrientation != UIInterfaceOrientationPortraitUpsideDown);
}
-(void)dealloc
{
[btnEdit release];
[super dealloc];
}
#pragma mark -
#pragma mark Update Masked Image Method
#pragma mark -
-(void)updateMaskedImage
{
maskView.hidden = YES;
UIImage *finalImage =
[self maskImage:[self captureView:self.view]
withMask:[UIImage imageNamed:@"mask.png"]];
maskView.hidden = NO;
//UIImage *finalImage = [self maskImage:photoView.image withMask:[UIImage imageNamed:@"mask.png"]];
[displayImage setImage:finalImage];
}
- (UIImage*) maskImage:(UIImage *)image withMask:(UIImage *)maskImage {
CGImageRef maskRef = maskImage.CGImage;
CGImageRef mask = CGImageMaskCreate(CGImageGetWidth(maskRef),
CGImageGetHeight(maskRef),
CGImageGetBitsPerComponent(maskRef),
CGImageGetBitsPerPixel(maskRef),
CGImageGetBytesPerRow(maskRef),
CGImageGetDataProvider(maskRef), NULL, false);
CGImageRef masked = CGImageCreateWithMask([image CGImage], mask);
return [UIImage imageWithCGImage:masked];
}
#pragma mark -
#pragma mark Touches Began
#pragma mark -
// adjusts the editing flag to make dragging and drop work
-(void)toggleEditing
{
if(!isEditing)
{
isEditing = true;
NSLog(@"editing...");
[btnEdit setTitle:@"Stop Editing" forState:UIControlStateNormal];
displayImage.hidden = YES;
photoView.hidden = NO;
maskView.hidden = NO;
}
else
{
isEditing = false;
[self updateMaskedImage];
NSLog(@"stopped editting");
[btnEdit setTitle:@"Start Editing" forState:UIControlStateNormal];
displayImage.hidden = NO;
photoView.hidden = YES;
maskView.hidden = YES;
}
}
/*
-(void)touchesMoved:(NSSet *)touches withEvent:(UIEvent *)event
{
if(isEditing)
{
UITouch *finger = [touches anyObject];
CGPoint currentPosition = [finger locationInView:self.view];
//[maskView setCenter:currentPosition];
//[photoView setCenter:currentPosition];
if([touches count] == 1)
{
[photoView setCenter:currentPosition];
}
else if([touches count] == 2)
{
}
}
}
*/
-(void)handlePan:(UIPanGestureRecognizer *)recognizer
{
CGPoint translation = [recognizer translationInView:self.view];
recognizer.view.center = CGPointMake(recognizer.view.center.x + translation.x,
recognizer.view.center.y + translation.y);
[recognizer setTranslation:CGPointMake(0, 0) inView:self.view];
}
-(void)handlePinch:(UIPinchGestureRecognizer *)recognizer
{
recognizer.view.transform = CGAffineTransformScale(recognizer.view.transform, recognizer.scale, recognizer.scale);
recognizer.scale = 1;
}
-(BOOL)gestureRecognizer:(UIGestureRecognizer *)gestureRecognizer shouldRecognizeSimultaneouslyWithGestureRecognizer:(UIGestureRecognizer *)otherGestureRecognizer
{
return YES;
}
#pragma mark -
#pragma mark Capture Screen Function
#pragma mark -
- (UIImage*)captureView:(UIView *)yourView
{
UIGraphicsBeginImageContextWithOptions(yourView.bounds.size, yourView.opaque, 0.0);
CGContextRef context = UIGraphicsGetCurrentContext();
[yourView.layer renderInContext:context];
UIImage *image = UIGraphicsGetImageFromCurrentImageContext();
UIGraphicsEndImageContext();
return image;
}
#pragma mark -
@end
所以我創建了自定義手勢識別器,因為沒有人給我一個更好的解決方案,達到了預期的效果。 下面是關鍵代碼片段,允許自定義識別器指示視圖應重新定位的位置以及新的比例應該是什么,以質心作為平移和縮放效果的中心,以便手指下方的像素保持在手指下方時間,除非手指看起來旋轉,這是不受支持的,我無法做任何事情來阻止他們這樣的手勢。 該手勢識別器用兩個手指同時平移和縮放。 我需要稍后為一個手指平移添加支持,即使兩個手指中的一個被抬起。
- (void)touchesMoved:(NSSet *)touches withEvent:(UIEvent *)event
{
// We can only process if we have two fingers down...
if ( FirstFinger == nil || SecondFinger == nil )
return;
// We do not attempt to determine if the first finger, second finger, or
// both fingers are the reason for this method call. For this reason, we
// do not know if either is stale or updated, and thus we cannot rely
// upon the UITouch's previousLocationInView method. Therefore, we need to
// cache the latest UITouch's locationInView information each pass.
// Break down the previous finger coordinates...
float A0x = PreviousFirstFinger.x;
float A0y = PreviousFirstFinger.y;
float A1x = PreviousSecondFinger.x;
float A1y = PreviousSecondFinger.y;
// Update our cache with the current fingers for next pass through here...
PreviousFirstFinger = [FirstFinger locationInView:nil];
PreviousSecondFinger = [SecondFinger locationInView:nil];
// Break down the current finger coordinates...
float B0x = PreviousFirstFinger.x;
float B0y = PreviousFirstFinger.y;
float B1x = PreviousSecondFinger.x;
float B1y = PreviousSecondFinger.y;
// Calculate the zoom resulting from the two fingers moving toward or away from each other...
float OldScale = Scale;
Scale *= sqrt((B0x-B1x)*(B0x-B1x) + (B0y-B1y)*(B0y-B1y))/sqrt((A0x-A1x)*(A0x-A1x) + (A0y-A1y)*(A0y-A1y));
// Calculate the old and new centroids so that we can compare the centroid's movement...
CGPoint OldCentroid = { (A0x + A1x)/2, (A0y + A1y)/2 };
CGPoint NewCentroid = { (B0x + B1x)/2, (B0y + B1y)/2 };
// Calculate the pan values to apply to the view so that the combination of zoom and pan
// appear to apply to the centroid rather than the center of the view...
Center.x = NewCentroid.x + (Scale/OldScale)*(self.view.center.x - OldCentroid.x);
Center.y = NewCentroid.y + (Scale/OldScale)*(self.view.center.y - OldCentroid.y);
}
視圖控制器通過將新比例和中心分配給相關視圖來處理事件。 我注意到其他手勢識別器傾向於允許控制器進行一些數學運算,但我試圖在識別器中進行所有數學運算。
-(void)handlePixelTrack:(PixelTrackGestureRecognizer*)sender
{
sender.view.center= sender.Center;
sender.view.transform = CGAffineTransformMakeScale(sender.Scale, sender.Scale);
}
更簡單的解決方案是將視圖放在滾動視圖中。 然后你可以免費獲得捏和平底鍋。 否則,您可以將pan和pinch手勢委托設置為self,並為shouldRecognizeSimultaneously返回YES。 至於縮放到用戶手指的中心,我從來沒有正確地解決過這個問題,但它涉及在改變其比例之前操縱視圖層的anchorPoint
(我認為)。
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.