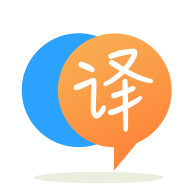
[英]How to show a user profile in a model from the list of users fetched using fetch api with javascript
[英]How to fetch on /users/profile and /users/friends with BackboneJS
我有很多網址的API,例如:
/users/profile -> GET the currently connected user /users/bestfriend -> GET the currently connected users' best friend (most bestfriend of bestfriends) /users/wife -> GET the currently connected users' wife /users/friends -> GET the currently connected users' friends /users/bestfriends -> GET the currently connected users' best friends /users/childrens -> GET the currently connected users' childrens /users/neighbours -> GET the currently connected users' neighbours
我是Backbone的新手,我真的不知道是否應該將當前連接的用戶作為集合或簡單模型來獲取。
我知道當前用戶可能會像Backbone doc提到的那樣受到提振,但這不是重點,只是假設我無法提升當前用戶模型。
那么,如何將提取的內容重定向到API URL? 我應該每次提供諸如{"operation":"profile"}
或{"operation":"friends"}
,還是在執行提取之前修改集合網址?
謝謝
如果要使用集合的“外部”模型,請使用model.urlRoot
屬性指定模型的URL。 如果是新模型,它將自動在URL末尾附加一個id(即id==null
),並在創建時發送POST。 否則,它將在指定的網址獲取(GET)。
如果不想在末尾附加ID:只需覆蓋model.url
即可生成要獲取/放入/發布到的實際端點URL。
集合還具有一個稱為url
的屬性-如果未指定模型的url或urlRoot,則將集合的url作為根(如果該模型是該集合的一部分)。
因此,您可以執行以下任一操作:
model.urlRoot = '/users/profile'; //appends id on put/delete requests
model.url = '/users/profile'; //direct endpoint. Can also be a function
collection.url = '/users/friends'; //fetch endpoint for collection. Could also be used by models to put to with appended id.
骨干網的文檔非常全面,可以自我解釋。 我建議您閱讀這些部分,例如Model.urlRoot
更新 :根據您的新URL
我認為造成混淆的原因是URL的設計方式。 端點為復數/單數不是一個好習慣:
例如: /user/friends
很好地獲得user的所有朋友。 但是哪個用戶呢? 更妙的是: /users/{id}/friends
獲取特定用戶的朋友。
為用戶獲取特定的朋友: /users/{id}/friends/{id}
我建議在您的URL中添加ID,以充分利用骨干網內置的REST功能。 否則,只需定義您自己的屬性和邏輯並具有單獨的功能(如“保存”)即可觸發$.ajax
調用您自己並在成功/錯誤回調上執行某些操作。
無論是模型還是收藏,完全取決於您。 幸運的是,在Backbone中有多種方法可以做到這一點。 如果您遵循BB的方法,它將提供一種更簡潔的處理方式,因為該框架期望並遵循某些約定。 如果沒有,您可以隨時自定義創建。 我做過兩種方式。 自定義創建似乎更容易,遵循約定需要一些計划,但是可以使其更加整潔,盡管“隱藏”隱藏/抽象了程序員的內容,而顯式ajax調用使其變得顯式。
這是一個權衡。 我建議您重組端點並相應地使用url屬性。 否則,請盡一切可能對您和您的團隊有意義,並堅持下去。
您必須創建幾個與您的一個URLS相關的模型和集合。
var Profile = Backbone.Model.extend({
url: "/users/profile"
});
var BestFriend = Backbone.Model.extend({
url: "/users/bestfriend"
});
// ...
var Friend = Backbone.Model.extend({});
var Friends = Backbone.Collection.extend({
model: Friend,
url: "/users/friends"
});
您還可以將Friend
用作BestFriend
的父類:
var BestFriend = Friend.extend({
url: "/users/bestfriend"
});
甚至創建一個Person
類作為每個人的父母:
var Person = Backbone.Model.extend({});
var Friends = Backbone.Collection.extend({
model: Person,
url: "/users/friends"
});
var Profile = Person.extend({
url: "/users/profile"
});
var BestFriend = Person.extend({
url: "/users/bestfriend"
});
由於您有單獨的URL來檢索每個元素的數據,因此應進行幾次訪存:
var profile = new Profile();
var bestFriend = new BestFriend();
var friends = new Friends();
profile.fetch();
bestfriend.fetch();
friends.fetch();
您可以使用以下內容將所有元素引用到您的Profile
實例中:
var Profile = Person.extend({
url: "/users/profile",
initialize: function( opts ){
this.bestFriend = opt.bestfriend;
// ...
}
});
var bestFriend = new BestFriend();
var friends = new Friends();
var profile = new Profile({
bestfriend: bestfriend
});
有幾種方法可以組織元素,也可以聽取有關事件的信息,但這只是品味的問題,您更喜歡哪種架構。
從本質上講,我同意第一個答案,但為簡單起見,您可以開始為用戶使用id並分別獲取它們。 這可能會產生一些額外的負載,但是在您需要進行優化之前,它應該使代碼更整潔。
當前的API設計可能會遇到問題。 如果將來您想要妻子的最好的朋友怎么辦? 您將擁有一個URL為“ / user / wife”的對象,然后說“ / user / wife / bestfriend”。 接下來,某人想要擴展它並使用“ /用戶/妻子/最好的朋友/孩子/”。 在后端解析非常復雜。
本質上,讓配置文件調用返回關聯用戶的ID而不是分別呼叫妻子和孩子:
/profile
{
id: 165735,
name: "Peter",
age: 45,
wifeId: 246247,
childrenIds: [352356, 134098]
}
然后拿到妻子將是:
/users/246247
這將使妻子成為真正的用戶,而不僅僅是某人的妻子。 檢索用戶時,您可以在此處檢查安全性,因為無論如何您都已將關系存儲在數據庫中。
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.