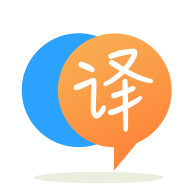
[英]Android:How to get time or timezone according to current location(geolocation/geocode)
[英]Geocode results based on current location
在我的一項活動中,我需要能夠進行地理編碼(通過地址字符串搜索找到位置)。 問題是我的結果過於廣泛。 當我搜索“ mcdonalds”時,得到的結果在美國不同地區。 我該如何做,以便用戶可以搜索附近的餐館(或任何位置),並且結果將在一定距離之內? 我的應用程序基本上需要更精確的結果。 這是發生的情況的屏幕截圖:
public class MainActivity extends MapActivity {
HelloItemizedOverlay itemizedOverlay;
List<Overlay> mapOverlays;
Drawable drawable;
@Override
public void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
MapView myMap = (MapView) findViewById(R.id.mapview);
myMap.setBuiltInZoomControls(true);
MapController mc = myMap.getController();
mapOverlays = myMap.getOverlays();
drawable = this.getResources().getDrawable(R.drawable.androidmarker);
itemizedOverlay = new HelloItemizedOverlay(drawable, this);
//geopoints are cordinates in microdegrees or degrees * E6
GeoPoint point = new GeoPoint(34730300, -86586100);
//GeoPoint point2 = locatePlace("texas", mc); //HelloItemizedOverlay.locatePlace("Texas", mc, myMap);
//overlayitems are items that show the point of location to the user
OverlayItem overlayitem = new OverlayItem(point, "Hola, Mundo!", "im in huntsville");
//OverlayItem overlayitem2 = new OverlayItem(point2, "Texas", "hi");
//itemizedoverlay is used here to add a drawable to each of the points
itemizedOverlay.addOverlay(overlayitem);
//itemizedOverlay.addOverlay(overlayitem2);
//this adds the drawable to the map
//this method converts the search address to locations on the map and then finds however many you wish to see.
locatePlace("mcdonalds", mc, 5);
mapOverlays.add(itemizedOverlay);
//this animates to the point desired (i plan on having "point" = current location of the user)
mc.animateTo(point);
}
@Override
public boolean onCreateOptionsMenu(Menu menu) {
getMenuInflater().inflate(R.menu.activity_main, menu);
return true;
}
@Override
protected boolean isRouteDisplayed(){
return false;
}
public void locatePlace(String locName, MapController mc, int numberToDisplay)
{ // code to make the google search via string work
// i use the Geocoder class is used to handle geocoding and reverse-geocoding. So make an instance of this class to work with the methods included
Geocoder geoCoder1 = new Geocoder(this, Locale.getDefault());
try {
List<Address> searchAddresses = geoCoder1.getFromLocationName(locName, numberToDisplay); // gets a max of 5 locations
if (searchAddresses.size() > 0)
{
//iterate through using an iterator loop (for loop would have been fine too)
//Iterator<Address> iterator1 = searchAddresses.iterator();
for (int i=0; i < searchAddresses.size(); i++){
//while (iterator1.hasNext()){
//step1 get a geopoint
GeoPoint tempGeoP = new GeoPoint( (int) (searchAddresses.get(i).getLatitude()*1E6), (int) (searchAddresses.get(i).getLongitude()*1E6) );
//step2 add the geopoint to the Overlay item
OverlayItem tempOverlayItm = new OverlayItem(tempGeoP, locName, "this is " + locName);
//step3 add the overlay item to the itemized overlay
HelloItemizedOverlay tempItemizedOverlay = new HelloItemizedOverlay(drawable, this); // its breakking here.........
tempItemizedOverlay.addOverlay(tempOverlayItm);
//the itemized overlay is added to the map Overlay
mapOverlays.add(tempItemizedOverlay);
}
}
} catch (IOException e)
{
e.printStackTrace();
// Log.e("the error", "something went wrong: "+ e);
}//finally {}
}
}
//這是Itemized覆蓋類中的重要代碼
public HelloItemizedOverlay(Drawable defaultMarker, Context context)
{
super(boundCenter(defaultMarker));
myContext = context;
}
public void addOverlay(OverlayItem overlay){
mOverlays.add(overlay);
populate();
}
謝謝亞當
這是根據Vishwa的注釋調整后的一些代碼:
// these 2 variables are my current location
latitudeCurrent = 34730300; // make this dynamic later
longitudeCurrent = -86586100; // make this dynamic later
LLlatitude = (latitudeCurrent - 100000)/(1E6); //lowerleft latitude = original lat - (1)*degree/10
LLlongitude = (longitudeCurrent+ 100000)/(1E6);//lowerleft longitude = original longitude - (1)*degree/10
URlatitude = (latitudeCurrent + 100000)/(1E6); //upperright latitude = original + (1)*degree/10
URlongitude = (longitudeCurrent+ 100000)/(1E6); //upperright longitude = original longitude + (1)*degree/10
try {
List<Address> searchAddresses = geoCoder1.getFromLocationName(locName, numberToDisplay, LLlatitude, LLlongitude, URlatitude, URlongitude);
這是您需要做的:
1.)使用GPS獲取用戶的當前位置,這是可以幫助您完成此操作的答案。
2)接下來,找出您希望顯示結果的半徑,即您需要的結果距離用戶位置2英里或5英里左右。
3.)找出此值(指點2)在緯度和經度值方面的含義,因此基本上,您必須定義一個具有LowerLeftLatitude,lowerLeftLongitude,lowerRightLatitude,lowerRightLongitude的邊界框。 這些計算可以通過計算一個經度換算成英里數來完成(再次,此計算可能是近似的,因為由於經度的工作原理,經地之間的距離因地而異(請閱讀: http://en.wikipedia.org/wiki/經度(如果您希望根據每個人的位置進行調整)
4.)使用以下方法(這是getFromLocationName的變體),而不是使用當前使用的版本: http : //developer.android.com/reference/android/location/Geocoder.html#getFromLocationName(java。 lang.String ,int,double,double,double,double)。 閱讀此方法的工作原理,基本上,除了名稱(即McDonalds)外,您現在還將指定邊界框值。 因此,現在,您將獲得更多特定於用戶位置的結果。
讓我知道如何/如果您需要更多幫助
這是從Places API獲取JSON響應的代碼:再次不要忘記用Places API密鑰替換“ AddYourOwnKeyHere”
try
{
HttpPost httppost = new HttpPost("https://maps.googleapis.com/maps/api/place/search/json?location=34.730300,-86.586100&radius=19308&types=food&name=mcdonalds&sensor=false&key=AddYourOwnKeyHere");
HttpClient httpclient = new DefaultHttpClient();
response = httpclient.execute(httppost);
String data = EntityUtils.toString(response.getEntity());
JSONObject json = new JSONObject(data);
//Parse the JSONObject now
} catch (Exception e) {
e.printStackTrace();
}
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.