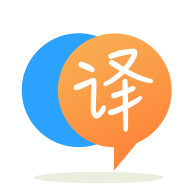
[英]What possible exceptions are there if User enters String instead of Int?
[英]Possible exception if User enters String instead of Int
我只是在玩Java,我試圖強迫我的程序只接受3位數字。 我相信我已經使用while循環成功完成了此操作(如果我輸入錯了,請糾正我)。 但是,如果用戶輸入字符串,我該如何打印一條錯誤語句。 例如:“ abc”。
我的代碼:
import java.util.Scanner;
public class DigitSum {
public static void main(String[] args) {
Scanner newScan = new Scanner(System.in);
System.out.println("Enter a 3 digit number: ");
int digit = newScan.nextInt();
while(digit > 1000 || digit < 100)
{
System.out.println("Error! Please enter a 3 digit number: ");
digit = newScan.nextInt();
}
System.out.println(digit);
}
}
這個怎么樣?
public class Sample {
public static void main (String[] args) {
Scanner newScan = new Scanner (System.in);
System.out.println ("Enter a 3 digit number: ");
String line = newScan.nextLine ();
int digit;
while (true) {
if (line.length () == 3) {
try {
digit = Integer.parseInt (line);
break;
}
catch (NumberFormatException e) {
// do nothing.
}
}
System.out.println ("Error!(" + line + ") Please enter a 3 digit number: ");
line = newScan.nextLine ();
}
System.out.println (digit);
}
}
regexp版本:
public class Sample {
public static void main (String[] args) {
Scanner newScan = new Scanner (System.in);
System.out.println ("Enter a 3 digit number: ");
String line = newScan.nextLine ();
int digit;
while (true) {
if (Pattern.matches ("\\d{3}+", line)) {
digit = Integer.parseInt (line);
break;
}
System.out.println ("Error!(" + line + ") Please enter a 3 digit number: ");
line = newScan.nextLine ();
}
System.out.println (digit);
}
}
將用於讀取int的代碼嵌入到try catch塊中,每當輸入錯誤的輸入時,它將生成一個異常,然后在catch塊中顯示所需的任何消息
如果輸入錯誤,則nextInt
方法本身會引發InputMismatchException
。
try {
digit = newScan.nextInt()
} catch (InputMismatchException e) {
e.printStackTrace();
System.err.println("Entered value is not an integer");
}
這應該做。
當您抓住輸入或拉動輸入字符串時,請通過parseInt
運行。 如果yourString
不是Integer
這實際上將引發異常:
Integer.parseInt(yourString)
而且,如果它引發異常,您就知道它不是有效的輸入,因此此時您可以顯示錯誤消息。 這是parseInt
上的文檔:
http://docs.oracle.com/javase/1.4.2/docs/api/java/lang/Integer.html#parseInt(java.lang.String )
您可以通過以下方式檢查字符串是否為數字值:
1)使用try / Catch塊
try
{
double d = Double.parseDouble(str);
}catch(NumberFormatException nfe) {
System.out.println("error");
}
2)使用正則表達式
if (!str.matches("-?\\d+(\\.\\d+)?")){
System.out.println("error");
}
3)使用NumberFormat類
NumberFormat formatter = NumberFormat.getInstance();
ParsePosition pos = new ParsePosition(0);
formatter.parse(str, pos);
if(str.length() != pos.getIndex()){
System.out.println("error");
}
4)使用Char.isDigit()
for (char c : str.toCharArray())
{
if (!Character.isDigit(c)){
System.out.println("error");
}
}
您可以查看如何檢查Java中的字符串是否為數字以獲取更多信息。
我將如何使用if語句。 if語句應該是這樣的:
if(input.hasNextInt()){
// code that you want executed if the input is an integer goes in here
} else {
System.out.println ("Error message goes here. Here you can tell them that you want them to enter an integer and not a string.");
}
注意:如果要讓他們輸入字符串而不是整數,請將if語句的條件更改為input.hasNextLine()
而不是input.hasNextInt()
。
第二點注意: input
就是我命名的掃描儀。 如果您命名煎餅,則應輸入pancakes.hasNextInt()
或pancakes.hasNextLine()
。
希望我有所幫助,祝你好運!
如果用戶輸入諸如“ abc”之類的字符串而不是整數值,則希望發生異常 ,那么InputMismatchException
適合您。
讓我給你一個基本的例子。
public static void main(String[] args)
{
Scanner ip = new Scanner(System.in);
int a;
System.out.println("Enter Some Input");
try{
a = ip.nextInt();
}
catch(InputMismatchException msg){
System.out.println("Input Mismatch Exception has occured " + msg.getMessage());
}
}
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.