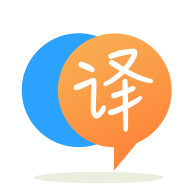
[英]Is it necessary to use @Configuration while working with spring annotations
[英]Spring, working with @Configuration and @Bean annotations
我有一個代碼:
@Configuration
public class BeanSample {
@Bean(destroyMethod = "stop")
public SomeBean someBean() throws Exception {
return new SomeBean("somebean name1");
}
class SomeBean {
String name;
public SomeBean(String name) {
this.name = name;
}
public void stop() {
System.out.println("stop");
}
}
public static void main(String[] args) throws Exception {
BeanSample beanSample = new BeanSample();
SomeBean someBean1 = beanSample.someBean();
ClassPathXmlApplicationContext appContext = new ClassPathXmlApplicationContext(
new String[] {"appContext.xml"});
SomeBean someBean2 = (SomeBean) appContext.getBean("someBean");
if (someBean1 == someBean2) System.out.println("OK");
}
}
我期望一旦啟動應用程序BeanSample.getSomeBean(),SomeBean就可以通過“ someBean”開始使用。
我現在有一個錯誤: 沒有定義名為“ someBean”的bean
實際上,我有點不明白應該使用哪個應用程序上下文來提取我的豆子?
關於@Configuration :
有什么原因,為什么我應該在這里使用@Configuration注釋? (通過這一步,我的IDE突出了我的類,因為那時它是與Spring相關的,所以應該很有意義)
- 好的:得到答案后,我的代碼如下:
public static void main(String[] args) throws Exception {
AnnotationConfigApplicationContext appContext = new AnnotationConfigApplicationContext(BeanSample.class);
SomeBean someBean2 = (SomeBean) appContext.getBean("someBean");
if (someBean2 != null) System.out.println("OK");
}
首先,如果使用Java配置,則必須實例化上下文,如下所示:
new AnnotationConfigApplicationContext(BeanSample.class)
其次, @Configuration
批注不會從被批注的類中生成bean。 僅@Bean
方法用於創建bean。
如果您也想擁有BeanSample
bean,則必須創建另一個@Bean
方法來創建一個。 但是話又說回來,你為什么要那樣? 我認為@Configuration
類只能用作配置容器,不能用於其他任何東西。
三,默認bean名字@Bean
不遵循屬性獲取的約定。 方法名稱直接使用,這意味着在您的示例中,bean將被命名為getSomeBean
而不是someBean
。 將方法更改為此:
@Bean(destroyMethod = "stop")
public SomeBean someBean() throws Exception {
return new SomeBean("somebean name1");
}
最后,不應實例化@Configuration
類。 它的方法僅用於創建bean。 然后,Spring將處理其生命周期,注入屬性等。 相反,如果您實例化該類並直接調用方法,則返回的對象將只是與Spring無關的普通對象。
由生產的豆
@Bean
public SomeBean getSomeBean()
將具有默認名稱-這是生產者方法getSomeBean
的名稱
所以你可以做兩件事
@Bean
public SomeBean getSomeBean() {...}
...
SomeBean bean = (SomeBean) appContext.getBean("getSomeBean");
if (bean != null) System.out.println("OK");
要么
@Bean(name="someBean")
public SomeBean getSomeBean() {...}
...
SomeBean bean = (SomeBean) appContext.getBean("someBean");
if (bean != null) System.out.println("OK");
我使用AnnotationConfigApplicationContext
而不是ClassPathXmlApplicationContext
一些完整示例
import java.util.Arrays;
import org.springframework.context.annotation.AnnotationConfigApplicationContext;
import org.springframework.context.annotation.Bean;
import org.springframework.context.annotation.Configuration;
@Configuration
public class BeanSample {
@Bean(name="someBean")
public SomeBean getSomeBean() throws Exception {
return new SomeBean("somebean name1");
}
class SomeBean {
String name;
public SomeBean(final String name) {
this.name = name;
}
public void stop() {
System.out.println("stop");
}
}
public static void main(final String[] args) throws Exception {
AnnotationConfigApplicationContext appContext = new AnnotationConfigApplicationContext(BeanSample.class);
BeanSample beanSample = (BeanSample) appContext.getBean("beanSample");
//next time use this to have a look at the beans in the context!
System.out.println(Arrays.toString(appContext.getBeanDefinitionNames()));
SomeBean bean = (SomeBean) appContext.getBean("someBean");
if (bean != null) System.out.println("OK");
}
}
輸出:
[org.springframework.context.annotation.internalConfigurationAnnotationProcessor,org.springframework.context.annotation.internalAutowiredAnnotationProcessor,org.springframework.context.annotation.internalRequiredAnnotationProcessor,org.springframework.context.annotation.internalCommonAnnotationProcessor, ,beanSample,org.springframework.context.annotation.ConfigurationClassPostProcessor $ ImportAwareBeanPostProcessor#0,someBean]確定
使用@Configuration
bean配置,您的測試應該看起來像這樣(以前使用上下文xml文件定義的內容現在已經使用@Configuration Java代碼定義了)
這將使用BeanSample創建一個提供bean配置的應用程序上下文:
AnnotationConfigApplicationContext ctx = new AnnotationConfigApplicationContext(BeanSample.class);
現在,在您的@Configuration
:
@Bean
public SomeBean someBean() throws Exception {
return new SomeBean("somebean name1");
}
Bean名稱是方法名稱。.因此,方法名稱上方是“ someBean”,在本例中,您將Bean名稱命名為“ getSomeBean”
因此,要查找bean,您必須執行以下操作:
SomeBean bean = appContext.getBean("someBean", SomeBean.class);
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.