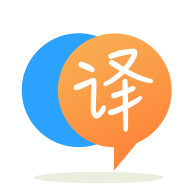
[英]ExecutorService and accepting new task after shutdown when waiting for other task to finish
[英]scheduling a periodic task after other threads finish
我想讓3個線程同時執行一個任務,並且我想讓一個線程在一段時間內執行計划的任務(每10秒運行一次)。 但是我希望當3個線程完成運行時僅調度一次任務,然后我希望該線程終止。 實現這些線程的最佳方法是什么? ExecutorService接口是否適合於此。
這是我剛剛編寫的示例:
import java.util.concurrent.ExecutorService;
import java.util.concurrent.Executors;
import java.util.concurrent.ScheduledFuture;
import java.util.concurrent.TimeUnit;
public class Example {
public static void main(String[] args) throws InterruptedException {
ScheduledFuture future = Executors.newScheduledThreadPool(1).scheduleAtFixedRate(new MyScheduledTask(), 0, 10, TimeUnit.SECONDS);
ExecutorService executor = Executors.newFixedThreadPool(3); // pool composed of 3 threads
for (int i = 0; i < 3; i++) {
// for the example assuming that the 3 threads execute the same task.
executor.execute(new AnyTask());
}
// This will make the executor accept no new threads
// and finish all existing threads in the queue
executor.shutdown();
// expect current thread to wait for the ending of the 3 threads
executor.awaitTermination(Long.MAX_VALUE, TimeUnit.TimeUnit.NANOSECONDS);
future.cancel(false); // to exit properly the scheduled task
// reprocessing the scheduled task only one time as expected
new Thread(new ScheduledTask()).start();
}
}
class MyScheduledTask implements Runnable {
@Override
public void run() {
//Process scheduled task here
}
}
class AnyTask implements Runnable {
@Override
public void run() {
//Process job of threads here
}
}
這是定期任務計划程序的一個示例,它一個接一個地執行任務。 任務計划程序接受一系列任務,並在指定的時間間隔后在單獨的線程中終止每個任務。 20秒后,主線程關閉調度程序並停止所有等待的任務。
import java.util.Arrays;
import java.util.Iterator;
import java.util.List;
import java.util.concurrent.CancellationException;
import java.util.concurrent.ExecutionException;
import java.util.concurrent.ExecutorService;
import java.util.concurrent.Executors;
import java.util.concurrent.ScheduledExecutorService;
import java.util.concurrent.ScheduledFuture;
import java.util.concurrent.TimeUnit;
public class Example {
class ScheduledRepeatingTask implements Runnable {
final ScheduledExecutorService scheduler = Executors
.newScheduledThreadPool(1);
List<Runnable> taskCollection;
Iterator<Runnable> taskIterator;
Runnable getCancelRunnable(final ScheduledFuture<?> future) {
Runnable cancelFuture = new Runnable() {
public void run() {
future.cancel(true);
}
};
return cancelFuture;
}
public ScheduledRepeatingTask(Runnable[] tasks) {
super();
taskCollection = Arrays.asList(tasks);
taskIterator = taskCollection.iterator();
}
public void shutdownScheduler() throws InterruptedException {
scheduler.shutdown();
boolean execTerminated = scheduler.awaitTermination(5,
TimeUnit.SECONDS);
if (!execTerminated) {
scheduler.shutdownNow();
}
}
public boolean isShutdown(){
return scheduler.isShutdown();
}
public void scheduleRepeatingTask(ScheduledFuture<?> future,
ScheduledFuture<?> futureCancel) {
try {
futureCancel.get();
future.get();
} catch (CancellationException e) {
System.out.println("cancelled.");
} catch (ExecutionException e) {
Throwable exp = e.getCause();
if (exp instanceof RuntimeException) {
System.out.println("failed.");
RuntimeException rt = (RuntimeException) exp;
throw rt;
}
} catch (InterruptedException e) {
Thread.currentThread().interrupt();
} finally {
if (!scheduler.isShutdown() && taskIterator.hasNext()) {
future = scheduler.scheduleAtFixedRate(taskIterator.next(),
2, 2, TimeUnit.SECONDS);
futureCancel = scheduler.schedule(
getCancelRunnable(future), 5, TimeUnit.SECONDS);
scheduleRepeatingTask(future, futureCancel);
}
}
}
@Override
public void run() {
if (!scheduler.isShutdown() && taskIterator.hasNext()) {
ScheduledFuture<?> future = scheduler.scheduleAtFixedRate(
taskIterator.next(), 2, 2, TimeUnit.SECONDS);
ScheduledFuture<?> futureCancel = scheduler.schedule(
getCancelRunnable(future), 5, TimeUnit.SECONDS);
scheduleRepeatingTask(future, futureCancel);
}
}
}
public static void main(String[] args) throws InterruptedException {
Example example = new Example();
ExecutorService executor = Executors.newCachedThreadPool();
Runnable[] tasks = { new Task1(), new Task2(), new Task1() };
ScheduledRepeatingTask scheduledRepeatingTask = example.new ScheduledRepeatingTask(tasks);
executor.execute(scheduledRepeatingTask);
Thread.sleep(20000);
scheduledRepeatingTask.shutdownScheduler();
executor.shutdown();
}
}
class Task1 implements Runnable {
@Override
public void run() {
System.out.println("Task 1");
}
}
class Task2 implements Runnable {
@Override
public void run() {
System.out.println("Task 2");
}
}
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.