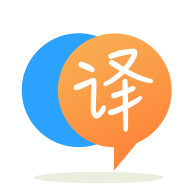
[英]unable to convert return value of std::bind to void function pointer
[英]convert std::bind to function pointer
我有一個第三方庫,它有一個方法,將函數指針作為第一個參數:
int third_party_method(void (*func)(double*, double*, int, int, double*), ...);
我想傳遞一個指向類聲明如下的方法的指針:
class TestClass
{
public:
void myFunction (double*, double*, int, int, void*);
我試圖傳遞這個函數如下:
TestClass* tc = new TestClass();
using namespace std::placeholders;
third_party_method(std::bind(&TestClass::myFunction, tc, _1, _2, _3, _4, _5), ...);
但是,這不編譯:
Conversion of parameter 1 from 'std::tr1::_Bind<_Result_type,_Ret,_BindN>' to 'void (__cdecl *)(double *,double *,int,int,void *)' is not possible
with
[
_Result_type=void,
_Ret=void,
_BindN=std::tr1::_Bind6<std::tr1::_Callable_pmf<void (__thiscall TestClass::* const )(double *,double *,int,int,void *),TestClass,false>,TestClass *,std::tr1::_Ph<1>,std::tr1::_Ph<2>,std::tr1::_Ph<3>,std::tr1::_Ph<4>,std::tr1::_Ph<5>>
]
有什么方法可以將成員傳遞給函數嗎?
有什么方法可以將成員傳遞給函數嗎?
除非你的類對象是某種全局對象 - 否則它是不可能的。 因為對象可能包含一些數據,而函數指針只是指向函數的指針 - 它不包含任何運行時上下文,只包含編譯時的上下文。
如果您接受每個回調傳遞的編譯時唯一ID,那么您可以使用以下通用方法。
用法:
void test(void (*fptr)())
{
fptr();
}
struct SomeStruct
{
int data;
void some_method()
{
cout << data << endl;
}
void another_method()
{
cout << -data << endl;
}
};
int main()
{
SomeStruct local[] = { {11}, {22}, {33} };
test(get_wrapper<0>( boost::bind(&SomeStruct::some_method,local[0]) ));
test(get_wrapper<1>( boost::bind(&SomeStruct::another_method,local[0]) ));
test(get_wrapper<2>( boost::bind(&SomeStruct::some_method,local[1]) ));
test(get_wrapper<3>( boost::bind(&SomeStruct::another_method,local[1]) ));
test(get_wrapper<4>( boost::bind(&SomeStruct::some_method,local[2]) ));
test(get_wrapper<5>( boost::bind(&SomeStruct::another_method,local[2]) ));
}
每次調用可能不需要唯一ID,例如,因為Functors可能已經有不同的類型,或者它們的運行時范圍不重疊。 但每次使用唯一ID更安全。
執行:
#include <boost/optional.hpp>
#include <boost/bind.hpp>
#include <iostream>
#include <ostream>
using namespace std;
template<unsigned ID,typename Functor>
boost::optional<Functor> &get_local()
{
static boost::optional<Functor> local;
return local;
}
template<unsigned ID,typename Functor>
typename Functor::result_type wrapper()
{
return get_local<ID,Functor>().get()();
}
template<typename ReturnType>
struct Func
{
typedef ReturnType (*type)();
};
template<unsigned ID,typename Functor>
typename Func<typename Functor::result_type>::type get_wrapper(Functor f)
{
(get_local<ID,Functor>()) = f;
return wrapper<ID,Functor>;
}
// ----------------------------------------------------------------------
void test(void (*fptr)())
{
fptr();
}
struct SomeStruct
{
int data;
void some_method()
{
cout << data << endl;
}
void another_method()
{
cout << -data << endl;
}
};
int main()
{
SomeStruct local[] = { {11}, {22}, {33} };
test(get_wrapper<0>( boost::bind(&SomeStruct::some_method,local[0]) ));
test(get_wrapper<1>( boost::bind(&SomeStruct::another_method,local[0]) ));
test(get_wrapper<2>( boost::bind(&SomeStruct::some_method,local[1]) ));
test(get_wrapper<3>( boost::bind(&SomeStruct::another_method,local[1]) ));
test(get_wrapper<4>( boost::bind(&SomeStruct::some_method,local[2]) ));
test(get_wrapper<5>( boost::bind(&SomeStruct::another_method,local[2]) ));
}
PS要注意多線程訪問 - 在這種情況下,您應該使用某種線程本地存儲數據。
它沒有編譯,因為第三方函數期望指向函數的指針,但是你試圖傳遞一個指向成員函數的指針。 這兩種類型根本不同 ,不能互換。 實際上,指向成員函數的指針通常是奇怪的動物 。
這是一個SSCCE,說明您遇到的問題:
#include <iostream>
#include <iomanip>
using namespace std;
typedef void(*SpeakFn)(void);
void Bark()
{
cout << "WOOF" << endl;
}
void Meow()
{
cout << "meeow" << endl;
}
void SpeakUsing(SpeakFn fn)
{
fn();
}
class Alligator
{
public:
void Speak()
{
cout << "YAWWW" << endl;
}
typedef void(Alligator::*AlligatorSpeakFn)(void);
void SpeakUsing(AlligatorSpeakFn fn)
{
(this->*fn)();
}
};
int main()
{
SpeakUsing(&Bark); // OK
Alligator a;
Alligator::AlligatorSpeakFn mem_fn = &Alligator::Speak;
a.SpeakUsing(mem_fn); // OK
SpeakUsing(mem_fn); // NOT OK -- can't cvt from fn-ptr to mem-fn-ptr
}
您不能使用指向成員函數的指針調用SpeakUsing
,因為它不能轉換為指向函數的指針。
請改用靜態成員函數,例如:
class Alligator
{
public:
static void Speak()
{
cout << "YAWWW" << endl;
}
typedef void(*AlligatorSpeakFn)(void);
void SpeakUsing(AlligatorSpeakFn fn)
{
fn();
}
};
正如其他人所提到的,你別無選擇,只能使用全局或靜態數據來提供綁定調用上下文作為原始函數。 但是如果提供的解決方案不是通用的,它就會遇到仿函數的空參數列表。 您需要為要綁定的每個不同的函數簽名手動編寫wrapper
, get_wrapper
和Func
,並為它們指定不同的名稱。
我想提出更廣泛的原始綁定解決方案:
#include <iostream>
#include <memory>
#include <functional>
#include <cassert>
// Raw Bind - simulating auto storage behavior for static storage data
template <typename BindFunctor, typename FuncWrapper> class scoped_raw_bind
{
public:
typedef scoped_raw_bind<BindFunctor, FuncWrapper> this_type;
// Make it Move-Constructible only
scoped_raw_bind(const this_type&) = delete;
this_type& operator=(const this_type&) = delete;
this_type& operator=(this_type&& rhs) = delete;
scoped_raw_bind(this_type&& rhs): m_owning(rhs.m_owning)
{
rhs.m_owning = false;
}
scoped_raw_bind(BindFunctor b): m_owning(false)
{
// Precondition - check that we don't override static data for another raw bind instance
if(get_bind_ptr() != nullptr)
{
assert(false);
return;
}
// Smart pointer is required because bind expression is copy-constructible but not copy-assignable
get_bind_ptr().reset(new BindFunctor(b));
m_owning = true;
}
~scoped_raw_bind()
{
if(m_owning)
{
assert(get_bind_ptr() != nullptr);
get_bind_ptr().reset();
}
}
decltype(&FuncWrapper::call) get_raw_ptr()
{
return &FuncWrapper::call;
}
static BindFunctor& get_bind()
{
return *get_bind_ptr();
}
private:
bool m_owning;
static std::unique_ptr<BindFunctor>& get_bind_ptr()
{
static std::unique_ptr<BindFunctor> s_funcPtr;
return s_funcPtr;
}
};
// Handy macro for creating raw bind object
// W is target function wrapper, B is source bind expression
#define RAW_BIND(W,B) std::move(scoped_raw_bind<decltype(B), W<decltype(B), __COUNTER__>>(B));
// Usage
///////////////////////////////////////////////////////////////////////////
// Target raw function signature
typedef void (*TargetFuncPtr)(double, int, const char*);
// Function that need to be called via bind
void f(double d, int i, const char* s1, const char* s2)
{
std::cout << "f(" << d << ", " << i << ", " << s1 << ", " << s2 << ")" << std::endl;
}
// Wrapper for bound function
// id is required to generate unique type with static data for
// each raw bind instantiation.
// THE ONLY THING THAT YOU NEED TO WRITE MANUALLY!
template <typename BindFunc, int id = 0> struct fWrapper
{
static void call(double d, int i, const char* s)
{
scoped_raw_bind<BindFunc, fWrapper<BindFunc, id>>::get_bind()(d, i, s);
}
};
int main()
{
using namespace std::placeholders;
auto rf1 = RAW_BIND(fWrapper, std::bind(&f, _1, _2, _3, "This is f trail - 1"));
TargetFuncPtr f1 = rf1.get_raw_ptr();
f1(1.2345, 42, "f1: Bind! Bind!");
auto rf2 = RAW_BIND(fWrapper, std::bind(&f, _1, _2, _3, "This is f trail - 2"));
TargetFuncPtr f2 = rf2.get_raw_ptr();
f2(10.2345, 420, "f2: Bind! Bind!");
auto rf3 = RAW_BIND(fWrapper, std::bind(&f, _1, _2, _3, "This is f trail - 3"));
TargetFuncPtr f3 = rf3.get_raw_ptr();
f3(100.2345, 4200, "f3: Bind! Bind!");
}
它經過測試 - 參見Live Action Here
不,不容易。 問題是函數指針有一個信息塊 - 函數的地址。 方法既需要對象的地址,也需要對象的地址,或者可以將對象地址作為參數傳遞。
有非常hackey的方法來做到這一點,但它們將是特定於平台的。 非常hackey。 這么多,我將推薦全局變量而不是使用它們。
如果有一個類的全局實例,你知道如何做到這一點,對吧?
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.