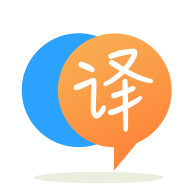
[英]Best practices or patterns for writing unit test results/reports to text file as well as to console
[英]Writing Out to Console as Well as to File
我試圖讓我的java程序的輸出寫入文件。
用戶輸入一些不應包含在文件中的數據。 當程序響應它應該輸出信息給用戶,並承擔全部輸出寫入到文件中。
從示例開始,我在驅動程序類的頂部開始:
static BufferedReader in = new BufferedReader(new InputStreamReader(System.in));
static String lineFromOutput;
這段代碼在我可能從程序接收輸出的每個地方:
try {
lineFromInput = in.readLine();
FileWrite.write(lineFromInput);
} catch (IOException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
而它的主叫是:
public class FileWrite {
public static void write(String message) {
PrintWriter out = null;
try {
out = new PrintWriter(new FileWriter("output.txt"), true);
} catch (IOException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
out.write(message);
out.close();
}
}
它會創建輸出文件,但就是這樣。 程序的輸出都沒有寫入。 我已經查看了很多例子,這似乎是最簡單的方法,盡管我對任何其他建議持開放態度。
謝謝!
每次寫入調用都會打開和關閉文本文件。 每次打開它都會被覆蓋,所以我希望只寫下最后一件事就會出現在文件中。
我建議從構造函數中打開輸出文件,然后從close方法關閉它。
我認為它應該是InputStremReader
,下面是單個t
語句:
static BufferedReader in= new BufferedReader(new OutputtStreamReader(System.in));
static String lineFromOutput;
如
static BufferedReader in = new BufferedReader(new InputStreamReader(System.in));
static String lineFromOutput;
編輯:這很好。 請確保通過輸入控制台提供輸入。 另請注意,它只讀取和寫入(覆蓋)單行。
public class FileWrite {
public static void write(String message) {
PrintWriter out = null;
try {
out = new PrintWriter(new FileWriter("output.txt"), true);
} catch (IOException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
out.write(message);
out.close();
}
public static void main(String[] args){
String lineFromInput;
try {
BufferedReader in = new BufferedReader(
new InputStreamReader(System.in));
lineFromInput = in.readLine();
FileWrite.write(lineFromInput);
in.close();
} catch (IOException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
}
}
編輯2:更新了多線輸入程序。 它不是每次寫入時打開和關閉文件的最佳方式,但我只是試圖讓您的程序稍作修改。 請告訴我,如果您需要建議以避免重復打開/關閉輸出文件。
更改亮點:
以append
模式打開文件。
public class FileWrite { public static void write(String message) { PrintWriter out = null; try { out = new PrintWriter(new FileWriter("output.txt", true), true); } catch (IOException e) { // TODO Auto-generated catch block e.printStackTrace(); } out.write(message); out.close(); } public static void main(String[] args){ String lineFromInput = ""; try { System.out.println("Provide the inputs in any number of lines"); System.out.println("Type \\"exit\\" in new line when done"); BufferedReader in = new BufferedReader( new InputStreamReader(System.in)); while(!"exit".equals(lineFromInput)){ lineFromInput = in.readLine(); FileWrite.write(lineFromInput+System.lineSeparator()); } in.close(); } catch (IOException e) { // TODO Auto-generated catch block e.printStackTrace(); } } }
EDIT3:使用Scanner
讀取輸入的更新程序:
private static HashMap<Integer, Object> shapes =
new HashMap<Integer, Object>();
static int i = 0;
public static void main(String[] args) {
PrintWriter output = null;
Scanner scanner = new Scanner(System.in);
try {
output = new PrintWriter(new FileWriter("output.txt"), true);
} catch (IOException e1) {
System.err.println("You don't have accress to this file");
System.exit(1);
}
String command = "";
while(!"quit".equalsIgnoreCase(command)){
System.out.println("Enter your Command: ");
command = scanner.next();
if (command.equalsIgnoreCase("create")) {
String type = scanner.next();
if (type.equalsIgnoreCase("line")) {
double length = scanner.nextDouble();
Line l = new Line(length);
scanner.nextLine();//flush the previous line
String line = scanner.nextLine();
output.format("%s", line);
shapes.put(i, l);
i++;
}else if (type.equalsIgnoreCase("circle")) {
double radius = scanner.nextDouble();
String color = scanner.next();
Circle c = new Circle(radius, Colors.valueOf(color));
scanner.nextLine();//flush the previous line
String line = scanner.nextLine();
output.format("%s", line);
shapes.put(i, c);
i++;
}else if (type.equals("rectangle")) {
double length = scanner.nextDouble();
double width = scanner.nextDouble();
String color = scanner.next();
Rectangle r = new Rectangle(length, width,
Colors.valueOf(color));
scanner.nextLine();//flush the previous line
String line = scanner.nextLine();
output.format("%s", line);
shapes.put(i, r);
i++;
}else if (type.equals("square")) {
double length = scanner.nextDouble();
String color = scanner.next();
Square s = new Square(length, Colors.valueOf(color));
scanner.nextLine();//flush the previous line
String line = scanner.nextLine();
output.format("%s", line);
shapes.put(i, s);
i++;
}
}else if (command.equals("printbyperimeter")) {
Shape[] shapeArray = shapes.values().toArray(new Shape[0]);
Arrays.sort(shapeArray);
System.out.println("Print in ascending order...");
for (int j = 0; j < shapeArray.length; j++) {
Shape temp = shapeArray[j];
if (temp.getClass().getName().equals("Line")) {
System.out.println("Shape: "
+ temp.getClass().getName() + ", Perimeter: "
+ temp.getPerimeter());
} else {
System.out.println("Shape: "
+ temp.getClass().getName() + ", Color: "
+ ((Colorable) temp).getColor()
+ ", Perimeter: " + temp.getPerimeter());
}
}
}else if (command.equals("printbyarea")) {
Shape[] shapeArray = shapes.values().toArray(new Shape[0]);
System.out.println("Print in random order...");
for (int j = 0; j < shapeArray.length; j++) {
Shape temp = shapeArray[j];
if (!temp.getClass().getName().equals("Line")) {
System.out.println("Shape: "
+ temp.getClass().getName() + ", Color: "
+ ((Colorable) temp).getColor() + ", Area: "
+ ((Areable) temp).getArea());
}
}
}else if (command.equals("quit")) {
scanner.close();
System.exit(0);
}
}
output.close();
}
嘗試使用代碼。 這個對我有用。 您只需要更改文件路徑以匹配您希望輸出的位置。 我在這里使用BufferedWriter,我認為是首選。
public static void main(String[] args) {
BufferedReader in = new BufferedReader(new InputStreamReader(System.in));
String lineFromOutput;
try {
lineFromOutput = in.readLine();
FileWrite.write(lineFromOutput);
} catch (IOException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
}
public static class FileWrite {
private static void write(String message) throws IOException {
BufferedWriter out = null;
try {
out = new BufferedWriter(new FileWriter(new File("C:\\Users\\Teresa\\Dropbox\\output.txt")));
//Replace the above line with your path.
out.write(message);
} catch (IOException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
out.close();
}
}
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.