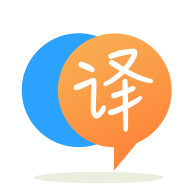
[英]A NullPointerException is being thrown but I can't figure out how to fix it.
[英]I can't figure out how to fix error NullPointerException
我認為smallIndex
, index
和temp
都有值,所以我不確定為什么會收到錯誤。 誰能向我解釋為什么會這樣? 錯誤消息是:
線程“主”中的異常java.lang.NullPointerException
public class LinkedList
{
public class LinkedListNode
{
public int info;
public LinkedListNode next;
public LinkedListNode back;
public LinkedListNode()
{
info = 0;
next = null;
back = null;
}
public LinkedListNode(int item)
{
info = item;
next = null;
back = null;
}
public void displayInfo()
{
System.out.print(info + " ");
}
}
protected int count;
protected LinkedListNode first;
protected LinkedListNode last;
public LinkedList()
{
first = null;
last = null;
count = 0;
}
public void initializeList()
{
first = null;
last = null;
count = 0;
}
public boolean isEmpty()
{
return (first == null);
}
public int length()
{
return count;
}
public void print()
{
LinkedListNode current = first;
while (current != null)
{
current.displayInfo();
current = current.next;
}
}
public void insertNode(int insertItem)
{
LinkedListNode newNode = new LinkedListNode(insertItem);
if (isEmpty())
{
first = newNode;
last = newNode;
count++;
}
else
{
last.next = newNode;
newNode.back = last;
}
last = newNode;
}
public LinkedListNode partition(LinkedList list,
LinkedListNode first, LinkedListNode last)
{
LinkedListNode smallIndex = first;
LinkedListNode index = smallIndex.next;
LinkedListNode temp = new LinkedListNode();
int pivot = first.info;
while (index != last.next)
{
if((index.info) < pivot)
{
smallIndex = smallIndex.next;
temp.info = index.info;
index.info = smallIndex.info;
smallIndex.info = temp.info;
}
index = index.next;
}
temp.info = first.info;
first.info = smallIndex.info;
smallIndex.info = temp.info;
System.out.print("The list after QuickSort is: ");
list.print();
System.out.print("\n");
return smallIndex;
}
public void recQuickSort(LinkedList list, LinkedListNode first,
LinkedListNode last)
{
while(first != last)
{
LinkedListNode pivotLocation = partition(list, first, last);
recQuickSort(list, first, pivotLocation.back);
recQuickSort(list, pivotLocation.next, last);
}
}
public void quickSortLinkedList(LinkedList list)
{
recQuickSort(list, list.first, list.last);
}
}
import java.util.*;
public class testLinkedListQuickSort
{
static Scanner console = new Scanner(System.in);
public static void main(String[] args)
{
LinkedList linkedlist = new LinkedList();
int num;
System.out.println("Enter numbers to add to linked list:");
num = console.nextInt();
while (num != 0)
{
linkedlist.insertNode(num);
num = console.nextInt();
}
linkedlist.quickSortLinkedList(linkedlist);
linkedlist.print();
}
}
關於您的評論:您調用分區與
recQuickSort(list, first, pivotLocation.back);
如果pivotLocation.back
為null
則使用last == null
調用分區方法,這將導致您的NPE。
在您的partition()
方法中,您沒有為null
值做太多檢查。 例如,如果smallIndex
, index
, temp
或last
為null
,那么它將smallIndex
。 還有first
,如果為null
,則會導致NPE。
確保在遞歸調用中傳遞的內容不是一個空節點是一個好主意。 我沒有看到很多檢查。
當您嘗試訪問沒有值的引用時,會發生NullPointerException
。
或在調用空對象的實例方法並訪問或修改空對象的字段時。
或者,當您將null傳遞給需要實際值的方法時。 意思是說空對象被非法使用。
我沒有仔細查看您的代碼,但要解決此問題,請確定哪個Object實例為null並引起問題。 您將需要修改代碼以添加適當的空檢查驗證
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.