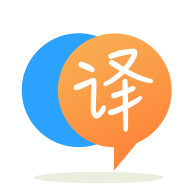
[英]How to write a byte array to OutputStream of process builder (Java)
[英]How to write array to OutputStream in Java?
我想通過Socket
發送多個隨機值。 我認為數組是發送它們的最佳方式。 但我不知道如何將數組寫入 Socket OutputStream
?
我的Java類:
導入 java.io.ByteArrayOutputStream; 導入 java.io.IOException; 導入 java.io.InputStream; 導入 java.net.Socket; 導入 java.io.*; 導入 java.util.Random;
public class NodeCommunicator {
public static void main(String[] args) {
try {
Socket nodejs = new Socket("localhost", 8181);
Random randomGenerator = new Random();
for (int idx = 1; idx <= 1000; ++idx){
Thread.sleep(500);
int randomInt = randomGenerator.nextInt(35);
sendMessage(nodejs, randomInt + " ");
System.out.println(randomInt);
}
while(true){
Thread.sleep(1000);
}
} catch (Exception e) {
System.out.println("Connection terminated..Closing Java Client");
System.out.println("Error :- "+e);
}
}
public static void sendMessage(Socket s, String message) throws IOException {
s.getOutputStream().write(message.getBytes("UTF-8"));
s.getOutputStream().flush();
}
}
使用 java.io.DataOutputStream / DataInputStream 對,他們知道如何讀取整數。 將信息作為長度+隨機數的數據包發送。
發件人
Socket sock = new Socket("localhost", 8181);
DataOutputStream out = new DataOutputStream(sock.getOutputStream());
out.writeInt(len);
for(int i = 0; i < len; i++) {
out.writeInt(randomGenerator.nextInt(35))
...
接收者
DataInputStream in = new DataInputStream(sock.getInputStream());
int len = in.readInt();
for(int i = 0; i < len; i++) {
int next = in.readInt();
...
Java 數組實際上是Object
,而且它們實現了Serializable
接口。 因此,您可以序列化您的數組,獲取字節並通過套接字發送這些字節。 這應該這樣做:
public static void sendMessage(Socket s, int[] myMessageArray)
throws IOException {
ByteArrayOutputStream bs = new ByteArrayOutputStream();
ObjectOutputStream os = new ObjectOutputStream(bs);
os.writeObject(myMessageArray);
byte[] messageArrayBytes = bs.toByteArray();
s.getOutputStream().write(messageArrayBytes);
s.getOutputStream().flush();
}
真正巧妙的是它不僅適用於int[]
還適用於任何Serializable
對象。
編輯:再想一想,這更簡單:
發件人:
public static void sendMessage(Socket s, int[] myMessageArray)
throws IOException {
OutputStream os = s.getOutputStream();
ObjectOutputStream oos = new ObjectOutputStream(os);
oos.writeObject(myMessageArray);
}
接收器:
public static int[] getMessage(Socket s)
throws IOException {
InputStream is = s.getInputStream();
ObjectInputStream ois = new ObjectInputStream(is);
int[] myMessageArray = (int[])ois.readObject();
return myMessageArray;
}
我將留下我的第一個答案,因為(它也有效並且)它對於將對象寫入沒有流的 UDP DatagramSockets
和DatagramPackets
很有用。
我建議使用一些分隔符(例如@@
簡單地連接字符串中的int
值,然后立即傳輸最終連接的字符串。 在接收端,只需使用相同的分隔符拆分字符串即可返回int[]
例如:
Random randomGenerator = new Random();
StringBuilder numToSend = new StringBuilder("");
numToSend.append(randomGenerator.nextInt(35));
for (int idx = 2; idx <= 1000; ++idx){
Thread.sleep(500);
numToSend.append("@@").append(randomGenerator.nextInt(35));
}
String numsString = numToSend.toString();
System.out.println(numsString);
sendMessage(nodejs, numsString);
在接收方,獲取您的字符串並拆分為:
Socket remotejs = new Socket("remotehost", 8181);
BufferedReader in = new BufferedReader(
new InputStreamReader(remotejs.getInputStream()));
String receivedNumString = in.readLine();
String[] numstrings = receivedNumString.split("@@");
int[] nums = new int[numstrings.length];
int indx = 0;
for(String numStr: numstrings){
nums[indx++] = Integer.parseInt(numStr);
}
好吧,流是字節流,因此您必須將數據編碼為字節序列,並在接收端對其進行解碼。 如何寫入數據取決於您希望如何對其進行編碼。
由於從 0 到 34 的數字適合單個字節,因此這很簡單:
outputStream.write(randomNumber);
另一方面:
int randomNumber = inputStream.read();
當然,在每個字節之后刷新流並不是很有效(因為它會為每個字節生成一個網絡數據包,並且每個網絡數據包都包含數十個字節的標頭和路由信息......)。 如果性能很重要,您可能也想使用 BufferedOutputStream。
因此,您可以比較我編寫的模板的替代格式,該模板允許您使用您希望的任何格式,或者比較替代格式。
abstract class DataSocket implements Closeable {
private final Socket socket;
protected final DataOutputStream out;
protected final DataInputStream in;
DataSocket(Socket socket) throws IOException {
this.socket = socket;
out = new DataOutputStream(new BufferedOutputStream(socket.getOutputStream()));
in = new DataInputStream(new BufferedInputStream(socket.getInputStream()));
}
public void writeInts(int[] ints) throws IOException {
writeInt(ints.length);
for (int i : ints)
writeInt(i);
endOfBlock();
}
protected abstract void writeInt(int i) throws IOException;
protected abstract void endOfBlock() throws IOException;
public int[] readInts() throws IOException {
nextBlock();
int len = readInt();
int[] ret = new int[len];
for (int i = 0; i < len; i++)
ret[i] = readInt();
return ret;
}
protected abstract void nextBlock() throws IOException;
protected abstract int readInt() throws IOException;
public void close() throws IOException {
out.close();
in.close();
socket.close();
}
}
二進制格式,4 字節整數
class BinaryDataSocket extends DataSocket {
BinaryDataSocket(Socket socket) throws IOException {
super(socket);
}
@Override
protected void writeInt(int i) throws IOException {
out.writeInt(i);
}
@Override
protected void endOfBlock() throws IOException {
out.flush();
}
@Override
protected void nextBlock() {
// nothing
}
@Override
protected int readInt() throws IOException {
return in.readInt();
}
}
停止位編碼二進制,每 7 位一個字節。
class CompactBinaryDataSocket extends DataSocket {
CompactBinaryDataSocket(Socket socket) throws IOException {
super(socket);
}
@Override
protected void writeInt(int i) throws IOException {
// uses one byte per 7 bit set.
long l = i & 0xFFFFFFFFL;
while (l >= 0x80) {
out.write((int) (l | 0x80));
l >>>= 7;
}
out.write((int) l);
}
@Override
protected void endOfBlock() throws IOException {
out.flush();
}
@Override
protected void nextBlock() {
// nothing
}
@Override
protected int readInt() throws IOException {
long l = 0;
int b, count = 0;
while ((b = in.read()) >= 0x80) {
l |= (b & 0x7f) << 7 * count++;
}
if (b < 0) throw new EOFException();
l |= b << 7 * count;
return (int) l;
}
}
以新行結尾的文本編碼。
class TextDataSocket extends DataSocket {
TextDataSocket(Socket socket) throws IOException {
super(socket);
}
private boolean outBlock = false;
@Override
protected void writeInt(int i) throws IOException {
if (outBlock) out.write(' ');
out.write(Integer.toString(i).getBytes());
outBlock = true;
}
@Override
protected void endOfBlock() throws IOException {
out.write('\n');
out.flush();
outBlock = false;
}
private Scanner inLine = null;
@Override
protected void nextBlock() throws IOException {
inLine = new Scanner(in.readLine());
}
@Override
protected int readInt() throws IOException {
return inLine.nextInt();
}
}
如果您想向套接字發送多個隨機值,請選擇一種簡單的格式並讓雙方(發送方和接收方)都同意,例如您可以簡單地選擇一個分隔符;
並使用該分隔符創建一個包含所有值的字符串,然后發送
Android Socket SERVER 示例修改為接收整數數組而不是字符串:
...
class CommunicationThread implements Runnable {
private ObjectInputStream input;
public CommunicationThread(Socket clientSocket) {
try {
this.input = new ObjectInputStream(clientSocket.getInputStream());
} catch (IOException e) {
e.printStackTrace();
}
}
public void run() {
while (!Thread.currentThread().isInterrupted()) {
try {
int[] myMessageArray = (int[]) input.readObject();
String read = null;
read = String.format("%05X", myMessageArray[0] & 0x0FFFFF);
int i = 1;
do {
read = read + ", " + String.format("%05X", myMessageArray[i] & 0x0FFFFF);
i++;
} while (i < myMessageArray.length);
read = read + " (" + myMessageArray.length + " bytes)";
updateConversationHandler.post(new updateUIThread(read));
} catch (IOException e) {
e.printStackTrace();
} catch (ClassNotFoundException e) {
e.printStackTrace();
}
}
}
}
...
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.