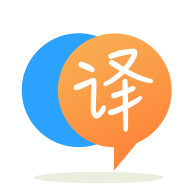
[英]How to check in JSP if user is logged (using JSTL <if> statement), and display username?
[英]JSP: How to check if a user is logged in?
我正在學習Java Web,但我遇到了一些問題,需要幫助。 我使用了template.jsp,其中包括header.jsp,footer.jsp,login.jsp(模板的左側)和${param.content}.jsp
。 對於名為X.jsp的每個頁面,我創建了另一個具有以下內容的jsp,因為我希望每個頁面具有相同的布局:
<jsp:include page="template.jsp">
<jsp:param name="content" value="X"/>
<jsp:param name="title" value="Start navigate"/>`enter code here`
</jsp:include>
例如,當我點擊Review鏈接時,我想重定向到Review.jsp,但是我遇到了一些問題。 在footer.jsp我有這樣的事情:
(...)
< a href =" Review.jsp "> Review </a>
(...)
登錄后,我嘗試單擊Review,它將我發送到Review.jsp,但它告訴我我沒有登錄。我使用Spring Framework,Tomcat 7,Eclipse,MySQL。 當我登錄時,我創建了一個cookie:
String timestamp = new Date().toString();
String sessionId = DigestUtils.md5Hex(timestamp);
db.addSession(sessionId, username, timestamp);
Cookie sid = new Cookie("sid", sessionId);
response.addCookie(sid);
對於每種方法,我都有類似的東西(我在教程中看到過):
@RequestMapping(value = "/writeReview", method = RequestMethod.GET)
public String nameMethod(@CookieValue("sid") String sid,...)
使用sid,我可以找出誰是用戶。 我有一個包含用戶帳戶和其他一些表的數據庫。 問題是當我點擊評論或其他任何內容時,它會告訴我我沒有登錄。我該怎么辦?
更新 :
我將JavaBean用於user和login.jsp。 具有登錄文本框的JSP,當我單擊按鈕登錄時,我有一個GET方法。
@RequestMapping(value = "/login", method = RequestMethod.GET)
public ModelAndView createCookie(
@RequestParam("username") String username, @RequestParam("password") String password,
HttpServletRequest request, HttpServletResponse response) throws SQLException {
//code for creating the cookie here, after testing if the user is in my database
}
對於每個頁面,我發送sid,我有一個模型的屬性,用戶名:
public String methodName(@CookieValue(required = false) String sid, Model model) {
if (sid != null) {
User user = getUserBySid(sid);
model.addAttribute("user", user);
}
(..other data.)
return "nameJSP.jsp";
}
我在每個JSP中檢查用戶名是否為空,這樣我就可以看到用戶是否已登錄。應用程序運行良好,如果我沒有點擊頁眉或頁腳中的鏈接,它會傳遞參數。 問題是我必須傳遞一個JSP中的參數,該參數是布局的實際內容到頁腳引用的JSP,這個JSP將是我布局的下一個內容。 布局僅識別內容和標題:
<title>${param.title}</title>
(I didn't paste all the code, I use a table <table>....)
<%@ include file="header.jsp"%>
<%@ include file="login.jsp"%>
<jsp:include page="${param.content}.jsp"/>
<%@ include file="footer.jsp"%>
那么如何在這個JSP中包含一個參數,該參數將從另一個JSP接收? 此外,它必須由layout.jsp訪問並發送到頁腳或標題?
<jsp:include page="layout.jsp">
<jsp:param name="content" value="X"/>
<jsp:param name="title" value="Start navigate"/>
</jsp:include>
要將一些參數傳遞給包含的JSP:
<jsp:include page="somePage.jsp" >
<jsp:param name="param1" value="someValue" />
<jsp:param name="param2" value="anotherParam"/>
....
</jsp:include>
你已經在做了。
好的,問題的細節不是很清楚,但我明白了。
其中一個解決方案可能如下。
在“ 登錄”操作中, 如果身份驗證成功,請創建HttpSession並為經過身份驗證的用戶設置屬性:
if (/* authentication was successfull */) {
request.getSession().setAttribute("loggedInUser", user);
...
}
在您控制的代碼中,如果用戶已登錄,只需檢查是否存在相應的HttpSession屬性:
HttpSession session = request.getSession(false);
if (session == null || session.getAttribute("loggedInUser") == null) {
// user is not logged in, do something about it
} else {
// user IS logged in, do something: set model or do whatever you need
}
或在你的JSP頁面,您可以檢查用戶是否使用登錄JSTL標簽作為例子說明了通過BalusC 這里 :
...
<c:if test="${not empty loggedInUser}">
<p>You're still logged in.</p>
</c:if>
<c:if test="${empty loggedInUser}">
<p>You're not logged in!</p>
</c:if>
...
但通常,您檢查用戶是否已登錄以限制對某些頁面的訪問(因此只有經過身份驗證的用戶才能訪問它們)。 你編寫了一些實現javax.servlet.Filter的類。
以下是我的一個項目的LoginFilter示例:
package com.example.webapp.filter;
import java.io.IOException;
import javax.servlet.Filter;
import javax.servlet.FilterChain;
import javax.servlet.FilterConfig;
import javax.servlet.ServletException;
import javax.servlet.ServletRequest;
import javax.servlet.ServletResponse;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import javax.servlet.http.HttpSession;
/**
* The purpose of this filter is to prevent users who are not logged in
* from accessing confidential website areas.
*/
public class LoginFilter implements Filter {
/**
* @see Filter#init(FilterConfig)
*/
@Override
public void init(FilterConfig filterConfig) throws ServletException {}
/**
* @see Filter#doFilter(ServletRequest, ServletResponse, FilterChain)
*/
@Override
public void doFilter(ServletRequest req, ServletResponse res, FilterChain chain) throws IOException, ServletException {
HttpServletRequest request = (HttpServletRequest) req;
HttpServletResponse response = (HttpServletResponse) res;
HttpSession session = request.getSession(false);
if (session == null || session.getAttribute("loggedInUser") == null) {
response.sendRedirect(request.getContextPath() + "/login.jsp");
} else {
chain.doFilter(request, response);
}
}
/**
* @see Filter#destroy()
*/
@Override
public void destroy() {}
}
在這個項目中,我使用普通的servlet和沒有Spring的JSP,但你會明白這個想法。
當然,您必須配置web.xml才能使用此過濾器:
<?xml version="1.0" encoding="UTF-8"?>
<web-app xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xmlns="http://java.sun.com/xml/ns/javaee"
xmlns:web="http://java.sun.com/xml/ns/javaee/web-app_3_0.xsd"
xsi:schemaLocation="http://java.sun.com/xml/ns/javaee http://java.sun.com/xml/ns/javaee/web-app_3_0.xsd"
version="3.0">
...
<filter>
<filter-name>Login Filter</filter-name>
<filter-class>com.example.webapp.filter.LoginFilter</filter-class>
</filter>
<filter-mapping>
<filter-name>Login Filter</filter-name>
<url-pattern>/*</url-pattern>
</filter-mapping>
...
</web-app>
注意 :
如果您使用的是支持Servlet 3.0及更高版本的Web容器或Application Server ,則可以使用@WebFilter批注對過濾器類進行批注,在這種情況下,無需在部署描述符 ( web.xml )中配置過濾器。 請參閱此處使用@WebFilter注釋和更多過濾器相關信息的示例。
希望這會幫助你。
或者只是使用:
String username = request.getRemoteUser();
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.