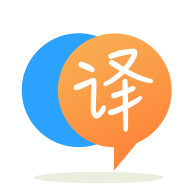
[英]JavaScript: Form onSubmit does not work when function is called 'submit'
[英]Javascript closure function not called on form submit
這令我感到困惑。 我有一個文本框。 輸入內容,然后按Enter鍵。 Javascript以單獨的形式創建只讀輸入元素。 文本輸入框旁邊是用於刪除它們的按鈕。 該表單底部還有一個提交按鈕,用於提交所有只讀文本輸入。
由於單擊表單內的按鈕將提交表單(我只想刪除包含按鈕及其相應文本輸入的父div),因此在表單提交時會調用一個函數。 此功能確定按下了哪種類型的按鈕(刪除或提交)並采取相應措施。
這就是問題所在。 按下刪除按鈕時,永遠不會調用函數destinations.enter
。 我所做的解決,這是創建一個名為全局函數submitDestinations
可復制的功能destinations.enter
。 如果調用此函數,一切都會順利進行。
有沒有人知道為什么destinations.enter
不會在提交時運行,但submitDestinations
會? 我想相信它與閉包有關,因為函數作用域似乎是兩個函數之間的唯一區別。 但是,這是我第一次使用閉包,我對它們的了解有限。
使用Javascript:
var destinations = (function(){
var max_destinations = 7;
var counter = 0;
function increment(){
counter += 1;
if(counter > max_destinations){
throw 'Too many destinations. (Max 7)'
}
}
function decrement(){
counter += 0;
if(counter < 0){
alert('Cannot have less than 0 destinations..')
throw 'Too few destinations. Get out of the console!'
}
}
return {
add : function(form){
try{
var formInput = form.elements[0];
var destination = formInput.value;
// Dont do anything if the input is empty
if(destination == ""){
return false;
}else{
// increment the destinations counter
increment();
}
}catch(err){
alert(err);
return false;
}
// add the text value to a visual element
var elem = document.createElement('div');
elem.setAttribute('class','destination');
// create the input
var input = document.createElement('input');
input.setAttribute('id','dest'+String(counter));
input.setAttribute('class','destinationText');
input.setAttribute('style','border: none');
input.setAttribute('name','destinations');
input.setAttribute('readonly','readonly');
input.setAttribute('value',destination);
// create the remove button
var button = document.createElement('button');
button.setAttribute('onclick','this.form.submitted=this;');//'return destinations.remove(this);');
button.setAttribute('class','removeButton')
button.setAttribute('id','but'+String(counter))
var buttonText = document.createTextNode('Remove');
button.appendChild(buttonText);
// add the elements to the div
elem.appendChild(input);
elem.appendChild(button);
var parent = document.getElementById('destinationsDiv');
parent.appendChild(elem);
// clear the input box
formInput.value = '';
return false;
},
enter : function(form){
alert('hi')
var button = form.submitted;
if(button.id != 'submitBtn'){
return remove(button);
}else{
return true;
}
return false;
},
remove : function(button){
try{
decrement();
}catch(err){
// do not allow less than 0 counter
alert(err);
return false;
}
// remove the button's parent div altogether
var toDelete = button.parentNode;
toDelete.parentNode.removeChild(toDelete);
return false;
}
}
})();
和HTML:
<div>
<form id='hi' onsubmit="return destinations.add(this);">
<input type="text" value="" />
</form>
<!--form id='submitDiv' method="post" onsubmit="alert(this.submitted);return submitDestinations(this);"-->
<form id='submitDiv' method="post" onsubmit="alert(this.submitted);return destinations.enter(this);">
<div id='destinationsDiv'>
<div>
<input id="dest1" class="destinationText" style="border: none" name="destinations" readonly="readonly" value="aadasd" \>
<button onclick="this.form.submitted=this;" class="removeButton" id="but1" \></button>
</div>
<div>
<input id="dest2" class="destinationText" style="border: none" name="destinations" readonly="readonly" value="Hi Stackoverflow" \>
<button onclick="this.form.submitted=this;" class="removeButton" id="but2" \></button>
</div>
</div>
<input type="submit" id='submitBtn' onclick="this.form.submitted=this;"/>
</form>
</div>
如果我將以下javascript函數添加到全局范圍並調用它,一切正常。 這與destinations.enter
完全相同
function submitDestinations(form){
var button = form.submitted;
if(button.id != 'submitBtn'){
return destinations.remove(button);
}else{
return true;
}
}
我在html中更改的是在提交時調用的方法:
<div>
<form id='hi' onsubmit="return destinations.add(this);">
<input type="text" value="" />
</form>
<form id='submitDiv' method="post" onsubmit="alert(this.submitted);return submitDestinations(this);">
<!--form id='submitDiv' method="post" onsubmit="alert(this.submitted);return destinations.enter(this);"-->
<div id='destinationsDiv'>
<div>
<input id="dest1" class="destinationText" style="border: none" name="destinations" readonly="readonly" value="aadasd" \>
<button onclick="this.form.submitted=this;" class="removeButton" id="but1" \></button>
</div>
<div>
<input id="dest2" class="destinationText" style="border: none" name="destinations" readonly="readonly" value="Hi Stackoverflow" \>
<button onclick="this.form.submitted=this;" class="removeButton" id="but2" \></button>
</div>
</div>
<input type="submit" id='submitBtn' onclick="this.form.submitted=this;"/>
</form>
</div>
事實證明存在命名沖突。 我正在創建的文本輸入的name屬性設置為“destinations”,與我在調用提交的javascript對象的名稱相同。 因此,“onsubmit”中的javascript嘗試引用DOM元素並在其上調用enter而不是引用我的javascript函數。
destination.enter()的實現存在錯誤。 它調用remove()來刪除按鈕,但名稱'remove'未綁定在定義targets.enter()的范圍內。
看起來好像不會在其他地方調用destinations.remove(),因此最簡單的修復只是將其移動到目標構造函數中的私有函數,而不是將其作為目標對象上的方法。
修改后的版本之所以有效,是因為您已將submitDestinations()的主體更改為調用目標.remove(),該范圍綁定在該范圍內。
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.