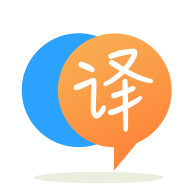
[英]Java iterating through all the files and only the unique RAR archives in a directory
[英]Iterating through a directory in java
嗨,現在我有以下方法,我用來在與具有此方法的類相同的目錄中一次讀取一個文件:
private byte[][] getDoubleByteArrayOfFile(String fileName, Region region)
throws IOException
{
BufferedImage image = ImageIO.read(getClass().getResource(fileName));
byte[][] alphaInputData =
new byte[region.getInputXAxisLength()][region.getInputYAxisLength()];
for (int x = 0; x < alphaInputData.length; x++)
{
for (int y = 0; y < alphaInputData[x].length; y++)
{
int color = image.getRGB(x, y);
alphaInputData[x][y] = (byte)(color >> 23);
}
}
return alphaInputData;
}
我想知道我怎么能這樣做,以便不是將“fileName”作為參數,而是將目錄名作為參數,然后遍歷該目錄中的所有文件並對其執行相同的操作。 謝謝!
如果您使用的是Java 7,那么您需要查看NIO.2 。
具體來說,請查看列表目錄的內容部分。
Path dir = Paths.get("/directory/path");
try (DirectoryStream<Path> stream = Files.newDirectoryStream(dir)) {
for (Path file: stream) {
getDoubleByteArrayOfFile(file.getFileName(), someRegion);
}
} catch (IOException | DirectoryIteratorException x) {
// IOException can never be thrown by the iteration.
// In this snippet, it can only be thrown by newDirectoryStream.
System.err.println(x);
}
這是一個可能有用的快速示例:
private ArrayList<byte[][]> getDoubleByteArrayOfDirectory(String dirName,
Region region) throws IOException {
ArrayList<byte[][]> results = new ArrayList<byte[][]>();
File directory = new File(dirName);
if (!directory.isDirectory()) return null //or handle however you wish
for (File file : directory.listFiles()) {
results.add(getDoubleByteArrayOfFile(file.getName()), region);
}
return results;
}
不完全是你要求的,因為它包裝你的舊方法而不是重寫它,但我發現它更清潔這種方式,讓你可以選擇仍然處理單個文件。 務必根據您的實際要求調整返回類型以及如何處理該region
(很難從問題中得知)。
它很簡單,使用File#listFiles()
返回指定File中的文件列表,該列表必須是目錄。 要確保File是目錄,只需使用File#isDirectory()
。 在您決定如何返回字節緩沖區時會出現問題。 由於該方法返回2d緩沖區,因此必須使用3d字節緩沖區數組,或者在這種情況下,List似乎是最佳選擇,因為相關目錄中將存在未知數量的文件。
private List getDoubleByteArrayOfDirectory(String directory, Region region) throws IOException {
File directoryFile = new File(directory);
if(!directoryFile.isDirectory()) {
throw new IllegalArgumentException("path must be a directory");
}
List results = new ArrayList();
for(File temp : directoryFile.listFiles()) {
if(temp.isDirectory()) {
results.addAll(getDoubleByteArrayOfDirectory(temp.getPath(), region));
}else {
results.add(getDoubleByteArrayOfFile(temp.getPath(), region));
}
}
return results;
}
您可以查看列表和listFiles文檔,了解如何執行此操作。
我們也可以使用遞歸來處理帶子目錄的目錄。 這里我將逐個刪除文件,您可以調用任何其他函數來處理它。
public static void recursiveProcess(File file) {
//to end the recursive loop
if (!file.exists())
return;
//if directory, go inside and call recursively
if (file.isDirectory()) {
for (File f : file.listFiles()) {
//call recursively
recursiveProcess(f);
}
}
//call processing function, for example here I am deleting
file.delete();
System.out.println("Deleted (Processed) file/folder: "+file.getAbsolutePath());
}
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.