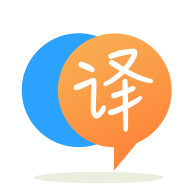
[英]boost.Python How to expose typedef of boost::shared_ptr?
[英]Using shared_ptr to abstract base as holder with boost.python
我們小組正在使用C ++開發一個數字框架。 我們現在想要將我們框架的基本部分包裝在Python中。 我們選擇的武器是Boost.Python,因為我們已經將Boost用於其他目的。 我們只使用smart_ptrs來支持多態性。 以下代碼段是我們如何應用策略模式的簡單示例:
#include <boost/shared_ptr.hpp>
struct AbsStrategy
{
virtual std::string talk( ) = 0;
};
typedef boost::shared_ptr<AbsStrategy> StrategyPtr;
struct Foo : AbsStrategy
{
std::string talk( )
{
return "I am a Foo!";
}
};
struct Bar : AbsStrategy
{
std::string talk( )
{
return "I am a Bar!";
}
};
struct Client
{
Client( StrategyPtr strategy ) :
myStrategy( strategy )
{
}
bool checkStrategy( StrategyPtr strategy )
{
return ( strategy == myStrategy );
}
StrategyPtr myStrategy;
};
如果我像這樣用Boost.Python包裝整個東西
#include <boost/python.hpp>
using namespace boost::python;
BOOST_PYTHON_MODULE( BoostPython )
{
class_<Foo>( "Foo" )
.def( "talk", &Foo::talk );
class_<Bar>( "Bar" )
.def( "talk", &Bar::talk );
class_<Client>( "Client", init<StrategyPtr>( ) )
.def( "checkStrategy", &Client::checkStrategy );
}
編譯期間會彈出以下警告
C:/boost/include/boost-1_51/boost/python/object/instance.hpp:14:36: warning: type attributes ignored after type is already defined [-Wattributes]
當我嘗試在python中使用包裝器時,我收到以下錯誤
>>> from BoostPython import *
>>> foo = Foo()
>>> bar = Bar()
>>> client = Client(foo)
Traceback (most recent call last):
File "<stdin>", line 1, in <module>
Boost.Python.ArgumentError: Python argument types in
Tree.__init__(Tree, Foo)
did not match C++ signature:
__init__(_object*, boost::shared_ptr<AbsStrategy>)
>>> client = Client(bar)
Traceback (most recent call last):
File "<stdin>", line 1, in <module>
Boost.Python.ArgumentError: Python argument types in
Tree.__init__(Tree, Bar)
did not match C++ signature:
__init__(_object*, boost::shared_ptr<AbsStrategy>)
在不改變框架的情況下,讓整個工作變得有效的缺失是什么? 包裝紙當然可以自由調整。
好的,我找到了解決方案。 必須在模塊聲明中使用implicitly_convertible
,如下所示。
#include <boost/python.hpp>
using namespace boost::python;
BOOST_PYTHON_MODULE( BoostPython )
{
class_<Foo>( "Foo" )
.def( "talk", &Foo::talk );
class_<Bar>( "Bar" )
.def( "talk", &Bar::talk );
class_<Client>( "Client", init<StrategyPtr>( ) )
.def( "checkStrategy", &Client::checkStrategy );
implicitly_convertible<boost::shared_ptr<Foo> , StrategyPtr>();
implicitly_convertible<boost::shared_ptr<Bar> , StrategyPtr>();
}
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.