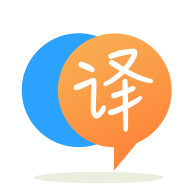
[英]What is the difference between “long”, “long long”, “long int”, and “long long int” in C++?
[英]What is the difference between an int and a long in C++?
如果我错了请纠正我,
int为4个字节,取值范围为-2,147,483,648至2,147,483,647(2 ^ 31)
long是4个字节,取值范围为-2,147,483,648到2,147,483,647(2 ^ 31)
C ++有什么区别? 它们可以互换使用吗?
它取决于实现。
例如,在Windows下它们是相同的,但是例如在Alpha系统上,long是64位,而int是32位。 本文介绍了可变平台上的Intel C ++编译器的规则。 总结一下:
OS arch size
Windows IA-32 4 bytes
Windows Intel 64 4 bytes
Windows IA-64 4 bytes
Linux IA-32 4 bytes
Linux Intel 64 8 bytes
Linux IA-64 8 bytes
Mac OS X IA-32 4 bytes
Mac OS X Intel 64 8 bytes
您唯一的保证是:
sizeof(char) == 1
sizeof(char) <= sizeof(short) <= sizeof(int) <= sizeof(long) <= sizeof(long long)
// FROM @KTC. The C++ standard also has:
sizeof(signed char) == 1
sizeof(unsigned char) == 1
// NOTE: These size are not specified explicitly in the standard.
// They are implied by the minimum/maximum values that MUST be supported
// for the type. These limits are defined in limits.h
sizeof(short) * CHAR_BIT >= 16
sizeof(int) * CHAR_BIT >= 16
sizeof(long) * CHAR_BIT >= 32
sizeof(long long) * CHAR_BIT >= 64
CHAR_BIT >= 8 // Number of bits in a byte
参见: 是long
保证至少有32位?
当为x64进行编译时,int和long之间的差异在0到4个字节之间,具体取决于您使用的编译器。
GCC使用LP64模型,这意味着在64位模式下,整数是32位,而long是64位。
例如,MSVC使用LLP64模型,这意味着即使在64位模式下,int和long均为32位。
C ++规范本身 (旧版本,但对此已经足够好了)使此问题悬而未决。
有四种有符号整数类型:'
signed char
','short int
','int
'和'long int
'。 在此列表中,每种类型提供的存储量至少与列表中位于其前面的类型相同。 普通整数具有执行环境的体系结构建议的自然大小*;[脚注:即,它足够大以包含标题
<climits>
定义的INT_MIN和INT_MAX范围内的任何值。 ---结束foonote]
正如Kevin Haines指出的那样,int具有执行环境建议的自然大小,必须适合INT_MIN和INT_MAX。
C89标准规定UINT_MAX
应该至少为2 ^ 16-1, USHRT_MAX
2 ^ 16-1和ULONG_MAX
2 ^ 32-1。 这使得short和int的位数至少为16,而long的位数至少为32。 对于char,它明确指出它应至少具有8位( CHAR_BIT
)。 C ++继承了limits.h文件的这些规则,因此在C ++中,我们对这些值具有相同的基本要求。 但是,您不应该得到从这个int至少为2个字节。 从理论上讲,char,int和long都可以是1个字节,在这种情况下CHAR_BIT
必须至少为32。请记住,“ byte”始终是char的大小,因此,如果char较大,则字节不仅是8位还有。
这取决于您的编译器。 您可以保证long至少与int一样大,但是不能保证它会更长。
在大多数情况下,字节数和值的范围由CPU的体系结构而不是C ++决定。 但是,C ++设置了最低要求,而litb对此进行了正确解释,而Martin York仅犯了一些错误。
您不能使用int和long互换的原因是因为它们的长度并不总是相同。 C是在PDP-11上发明的,其中一个字节有8位,int是两个字节,可以直接由硬件指令处理。 由于C程序员经常需要四字节算术,因此发明了long,它是四字节,由库函数处理。 其他机器具有不同的规格。 C标准提出了一些最低要求。
如果您曾经在另一台计算机体系结构,OS或另一家供应商的编译器上编译代码,那么依靠编译器供应商对基本类型大小的实现会困扰您。
大多数编译器供应商都提供一个头文件,该头文件定义具有显式类型大小的基本类型。 当有可能将代码移植到另一个编译器时,应使用这些原始类型(在每个实例中始终将其读取)。 例如,大多数UNIX编译器都具有int8_t uint8_t int16_t int32_t uint32_t
。 Microsoft具有INT8 UINT8 INT16 UINT16 INT32 UINT32
。 我更喜欢Borland / CodeGear的int8 uint8 int16 uint16 int32 uint32
。 这些名称还使您想起了预期值的大小/范围。
多年以来,我一直使用Borland的显式基元类型名称并#include
以下C / C ++头文件(primitive.h),该文件旨在为任何C / C ++编译器使用这些名称定义显式基元类型(此头文件可能实际上并不涵盖了所有编译器,但涵盖了我在Windows,UNIX和Linux上使用过的几个编译器,它也(尚未)定义64位类型)。
#ifndef primitiveH
#define primitiveH
// Header file primitive.h
// Primitive types
// For C and/or C++
// This header file is intended to define a set of primitive types
// that will always be the same number bytes on any operating operating systems
// and/or for several popular C/C++ compiler vendors.
// Currently the type definitions cover:
// Windows (16 or 32 bit)
// Linux
// UNIX (HP/US, Solaris)
// And the following compiler vendors
// Microsoft, Borland/Imprise/CodeGear, SunStudio, HP/UX
// (maybe GNU C/C++)
// This does not currently include 64bit primitives.
#define float64 double
#define float32 float
// Some old C++ compilers didn't have bool type
// If your compiler does not have bool then add emulate_bool
// to your command line -D option or defined macros.
#ifdef emulate_bool
# ifdef TVISION
# define bool int
# define true 1
# define false 0
# else
# ifdef __BCPLUSPLUS__
//BC++ bool type not available until 5.0
# define BI_NO_BOOL
# include <classlib/defs.h>
# else
# define bool int
# define true 1
# define false 0
# endif
# endif
#endif
#ifdef __BCPLUSPLUS__
# include <systypes.h>
#else
# ifdef unix
# ifdef hpux
# include <sys/_inttypes.h>
# endif
# ifdef sun
# include <sys/int_types.h>
# endif
# ifdef linux
# include <idna.h>
# endif
# define int8 int8_t
# define uint8 uint8_t
# define int16 int16_t
# define int32 int32_t
# define uint16 uint16_t
# define uint32 uint32_t
# else
# ifdef _MSC_VER
# include <BaseTSD.h>
# define int8 INT8
# define uint8 UINT8
# define int16 INT16
# define int32 INT32
# define uint16 UINT16
# define uint32 UINT32
# else
# ifndef OWL6
// OWL version 6 already defines these types
# define int8 char
# define uint8 unsigned char
# ifdef __WIN32_
# define int16 short int
# define int32 long
# define uint16 unsigned short int
# define uint32 unsigned long
# else
# define int16 int
# define int32 long
# define uint16 unsigned int
# define uint32 unsigned long
# endif
# endif
# endif
# endif
#endif
typedef int8 sint8;
typedef int16 sint16;
typedef int32 sint32;
typedef uint8 nat8;
typedef uint16 nat16;
typedef uint32 nat32;
typedef const char * cASCIIz; // constant null terminated char array
typedef char * ASCIIz; // null terminated char array
#endif
//primitive.h
C ++标准这样说:
3.9.1,§2:
有五种有符号整数类型:“有符号字符”,“ short int”,“ int”,“ long int”和“ long long int”。 在此列表中,每种类型提供的存储量至少与列表中位于其前面的类型相同。 普通整数具有执行环境的架构所建议的自然大小(44); 提供其他有符号整数类型以满足特殊需要。
(44),即足够大以包含标头
<climits>
定义的INT_MIN和INT_MAX范围内的任何值 。
结论:这取决于您正在使用的体系结构。 任何其他假设都是错误的。
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.