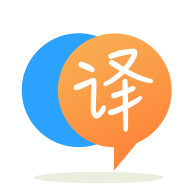
[英]Type error in C++ when instantiating a priority_queue of int pairs with a custom comparator (to implement a min heap)
[英]C++ overloading > for priority_queue (min heap): yields “invalid operands to binary expression ('const Node' and 'const Node')” on push() for heap
我正在尝试重载>运算符,因此可以对优先级队列执行此操作:
priority_queue<Node, vector<Node>, greater<Node> > unexplored_nodes;
我想将其用作最小堆。
这是Node结构的代码(我知道,最好不要使用带有公共变量的结构,但我只想快速又脏一些):
struct Node {
string repr;
float d;
vector<pair<Node, float> > neighbors;
Node(string repr) {
this->repr = repr;
this->d = FLT_MAX;
}
// **All three of these forms yield errors**
// Compiles without push() call below, but yields "invalids operands to binary expression" if I do have the push() call
//bool operator()(const Node* lhs, const Node* rhs) const {
// return lhs->d > rhs->d;
//}
// Compiles without push() call below, but yields "invalids operands to binary expression" if I have do the push() call
bool operator>(const Node &rhs) {
return d > rhs.d;
}
// Error regardless of priority queue below: overloaded 'operator>' must be a binary operator (has 3 parameters)
//bool operator>(const Node &lhs, const Node &rhs) {
// return lhs.d > rhs.d;
//}
};
void foo(const vector<Node> &list) {
priority_queue<Node, vector<Node>, greater<Node> > q;
q.push(list[0]); // this causes the 1st & 2nd overload attempt in the struct to have the "invalid operands" error
}
这就是我的编译方式:
clang++ -std=c++11 -stdlib=libc++ thefile.cc
在我尝试的所有3种方法中,都出现了一些错误(在代码中进行了注释)。 我曾从STL Priority Queue中创建Min Heap,以了解实现该方法的方法(我由larsmans尝试过),但是它们没有用。
如果有人可以提供帮助,我将不胜感激。 (另外,如果您有更好的方式将优先级队列用作最小堆,以避免操作员重载,那也将是个好主意……这是主要目的。)
您必须将运算符标记为const
:
bool operator> (const Node &rhs) const {
return d > rhs.d;
}
编辑
如果不想重载运算符,则可以为队列提供自己的比较器:
struct Comparator
{
bool operator() (const Node &lhs, const Node &rhs) const
{
return lhs.d > rhs.d;
}
};
void foo(const vector<Node> &list) {
priority_queue<Node, vector<Node>, Comparator> q;
q.push(list[0]);
}
编辑2
这是使用自定义地图的方法:
struct Comparator
{
typedef std::map<Node, float> Map;
explicit Comparator(const Map &map) : map(&map) {}
bool operator() (const Node &lhs, const Node &rhs) const
{
return map->find(lhs)->second > map->find(rhs)->second;
}
private:
const Map *map;
};
void foo(const vector<Node> &list, const Comparator::Map &map) {
priority_queue<Node, vector<Node>, Comparator> q(Comparator(map));
q.push(list[0]);
}
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.